Type Hinting feature in PHP7: How to prevent potential type errors?
Type Hinting feature in PHP7: How to prevent potential type errors?
Overview:
In software development, type errors are a common problem. Since PHP is a weakly typed language, it allows developers to define variable and function parameter types without restrictions. However, this can also lead to potential type errors, making debugging and maintenance more difficult.
In order to solve this problem, PHP7 introduced the Type Hinting feature. This feature allows developers to specify the expected data types in function parameters and return values, and enforce type checking at function call time. This article will introduce the use of Type Hinting in detail, and use specific code examples to illustrate how to prevent potential type errors.
- Type Hinting of basic types:
In PHP7, we can use the following basic data types for Type Hinting:
- int : Integer
- float: Floating point number
- string: String
- bool: Boolean value
- array: Array
The following is a sample code that demonstrates how to use Type Hinting to prevent potential type errors:
function calculateSum(int $num1, int $num2) { return $num1 + $num2; } $sum = calculateSum(5, 10); // 输出:15 $sum = calculateSum("5", "10"); // 报错:Argument 1 passed to calculateSum() must be of the type int, string given
In the above example, we define a calculateSum function, and the function parameters $num1 and $num2 are both specified. It is of type int. When we call the calculateSum function and pass in the integer parameter, the function executes normally and returns the correct result. However, when we pass in a string parameter, PHP throws a TypeError exception, telling us that the parameter type does not match the expected type.
- Type constraints and inheritance:
In addition to basic data types, we can also use custom classes for Type Hinting. This is useful for interactions between objects in large projects.
The following is a sample code for using a custom class for Type Hinting:
class User { private $name; public function __construct($name) { $this->name = $name; } public function getName() { return $this->name; } } function greetUser(User $user) { echo "Hello, " . $user->getName(); } $user = new User("John Doe"); greetUser($user); // 输出:Hello, John Doe $invalidUser = "John Doe"; greetUser($invalidUser); // 报错:Argument 1 passed to greetUser() must be an instance of User, string given
In the above example, we defined a User class and modified the User class in the greetUser function. Type constraints. When we pass a User object as a parameter, the function executes normally and outputs the correct results. However, when we pass a non-User object (such as a string), PHP throws a TypeError exception.
- Nullable type:
Sometimes, function parameters may be empty. To allow function parameters to be null, we can use question marks (?) in Type Hinting to represent nullable types.
The following is a sample code that demonstrates how to use nullable types:
function printMessage(?string $message) { echo $message; } printMessage("Hello, world!"); // 输出:Hello, world! printMessage(null); // 输出:无输出
In the above example, we define a printMessage function and specify the $message parameter as a nullable character String type. When we pass a non-empty string as argument, the function executes normally and outputs the result. However, when we pass null as parameter, the function does not output anything.
Conclusion:
Type Hinting is an important feature introduced in PHP7 that can help developers prevent potential type errors. By specifying the expected data types in function parameters and return values, we can enforce type checking when the function is called, helping us find and resolve type errors earlier. Using Type Hinting can improve the readability and maintainability of your code and reduce the time spent debugging and fixing errors. Therefore, we should make full use of the Type Hinting feature and use it reasonably during the development process to improve code quality.
The above is the detailed content of Type Hinting feature in PHP7: How to prevent potential type errors?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


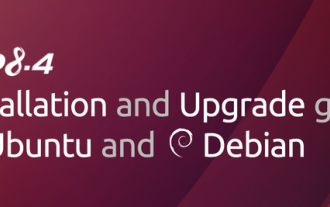
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
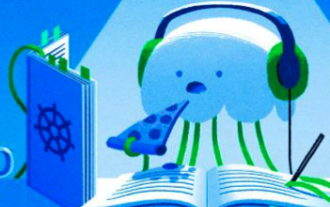
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
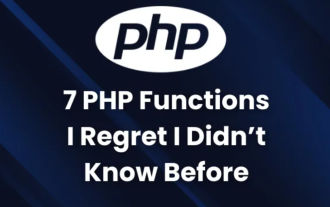
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
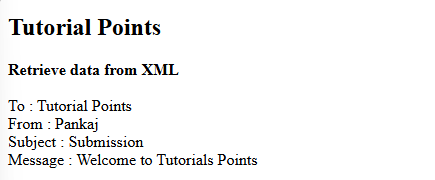
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
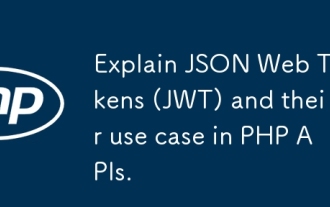
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
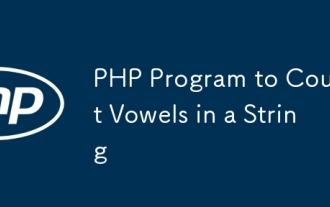
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
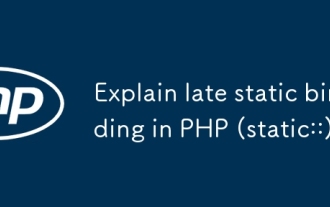
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
