How to implement reader and novel recommendations in uniapp
Title: Using UniApp to implement reader and novel recommendations
Introduction:
UniApp is a cross-platform application framework developed based on Vue.js. By using It provides multi-terminal unified development capabilities, and we can easily implement reader and novel recommendation functions. This article will detail how to implement these two functions in UniApp and provide corresponding code examples.
1. Implementation of reader function
- Create page structure
Create a page in UniApp and name it Reader. The page structure is as follows:
<template> <view class="reader"> <!-- 阅读器内容显示区域 --> <view class="content">{{ content }}</view> <!-- 阅读器功能区域 --> <view class="footer"> <!-- 前进按钮 --> <button class="prevBtn" @click="prevPage">上一页</button> <!-- 后退按钮 --> <button class="nextBtn" @click="nextPage">下一页</button> </view> </view> </template> <script> export default { data() { return { content: '' // 阅读器内容 } }, methods: { prevPage() { // 上一页操作 }, nextPage() { // 下一页操作 } } } </script> <style> .reader { height: 100vh; padding: 20px; } .content { height: 90%; font-size: 16px; line-height: 1.5; } .footer { display: flex; justify-content: space-between; padding-top: 20px; } .prevBtn, .nextBtn { padding: 10px; background-color: #333; color: #fff; } </style>
- Get novel data
In the above code, we define a content attribute in data to display the content in the reader. We need to obtain the corresponding novel data in the prevPage and nextPage methods in the methods section. You can use the axios or uni.request method to make a network request to obtain the chapter content of the novel. The sample code is as follows:
methods: { prevPage() { uni.request({ url: 'http://example.com/api/prevChapter', success: (res) => { this.content = res.data.content; } }); }, nextPage() { uni.request({ url: 'http://example.com/api/nextChapter', success: (res) => { this.content = res.data.content; } }); } }
- Page jump and data transfer
In practical applications, we usually click on a novel in the novel list and then jump to the reader page , and pass the corresponding novel id or chapter id. You can use the uni.navigateTo method to jump to the page and pass data through the url parameter. The sample code is as follows:
onItemClick(novelId, chapterId) { uni.navigateTo({ url: `/pages/reader/reader?novelId=${novelId}&chapterId=${chapterId}` }); }
In the Reader page, you can obtain the novelId and chapterId in the url parameter through the uni.getLaunchOptionsSync method.
2. Implementation of the novel recommendation function
- Obtain the recommended list data
In UniApp, we can use the uni.request method to send a network request to obtain the recommended novel list data . The sample code is as follows:
// 小说推荐页面代码 <template> <view class="recommend"> <view v-for="novel in novelList" :key="novel.id" class="novelItem"> <!-- 小说封面 --> <image class="coverImage" :src="novel.coverImageUrl"></image> <!-- 小说标题 --> <view class="title">{{ novel.title }}</view> </view> </view> </template> <script> export default { data() { return { novelList: [] // 推荐小说列表数据 } }, mounted() { this.getNovelList(); }, methods: { getNovelList() { uni.request({ url: 'http://example.com/api/recommendList', success: (res) => { this.novelList = res.data; } }); } } } </script> <style> .recommend { padding: 20px; } .novelItem { display: flex; align-items: center; margin-bottom: 20px; } .coverImage { width: 100px; height: 150px; margin-right: 10px; } .title { font-size: 16px; color: #333; } </style>
- Page jump and data transfer
In the novel recommendation page, after clicking on a novel, it will usually jump to the corresponding reader page, and Pass the novel id and chapter id. You can use the uni.navigateTo method in the click event of novelItem to jump to the page and pass the data through the url parameter. The sample code is as follows:
onItemClick(novelId, chapterId) { uni.navigateTo({ url: `/pages/reader/reader?novelId=${novelId}&chapterId=${chapterId}` }); }
The above is the sample code for using UniApp to implement the reader and novel recommendation functions. Through the above code examples, we can easily implement these two functions in UniApp and expand and optimize them according to specific needs. Hope this article helps you!
The above is the detailed content of How to implement reader and novel recommendations in uniapp. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


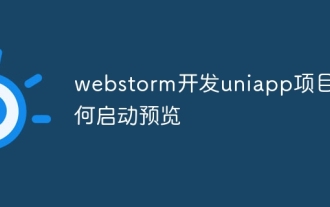
Steps to launch UniApp project preview in WebStorm: Install UniApp Development Tools plugin Connect to device settings WebSocket launch preview
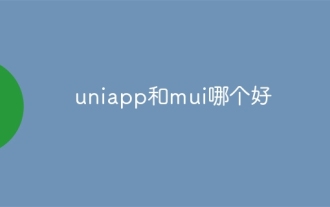
Generally speaking, uni-app is better when complex native functions are needed; MUI is better when simple or highly customized interfaces are needed. In addition, uni-app has: 1. Vue.js/JavaScript support; 2. Rich native components/API; 3. Good ecosystem. The disadvantages are: 1. Performance issues; 2. Difficulty in customizing the interface. MUI has: 1. Material Design support; 2. High flexibility; 3. Extensive component/theme library. The disadvantages are: 1. CSS dependency; 2. Does not provide native components; 3. Small ecosystem.
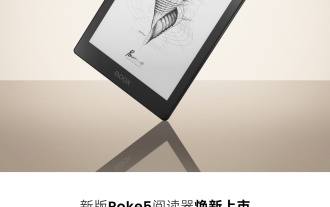
According to news from this website on March 13, Aragonite officially announced that the 2024 version of BOOXPoke5 reader is on the market, using a 6-inch Carta1300 ink screen, and the official starting price is 1,029 yuan. After checking the product details on this site, we learned that Aragonite Poke52024 has an upgraded screen compared to Poke5S. The Poke5S screen uses a non-flat glass cover and uses Carta1100 ink screen with a PPI of 212; Poke52024 has a flat screen cover. Microcrystal etched tempered glass uses a new Carta1300 ink screen, and the screen pixel density is increased to 300PPI. Other configurations of the new product are consistent with Poke5S: in terms of performance combination, Aragonite Poke52024 version adopts high-end
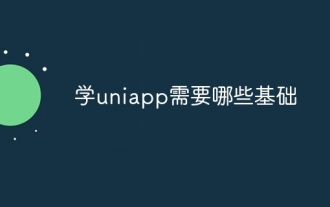
uniapp development requires the following foundations: front-end technology (HTML, CSS, JavaScript) mobile development knowledge (iOS and Android platforms) Node.js other foundations (version control tools, IDE, mobile development simulator or real machine debugging experience)
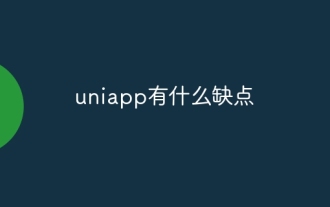
UniApp has many conveniences as a cross-platform development framework, but its shortcomings are also obvious: performance is limited by the hybrid development mode, resulting in poor opening speed, page rendering, and interactive response. The ecosystem is imperfect and there are few components and libraries in specific fields, which limits creativity and the realization of complex functions. Compatibility issues on different platforms are prone to style differences and inconsistent API support. The security mechanism of WebView is different from native applications, which may reduce application security. Application releases and updates that support multiple platforms at the same time require multiple compilations and packages, increasing development and maintenance costs.
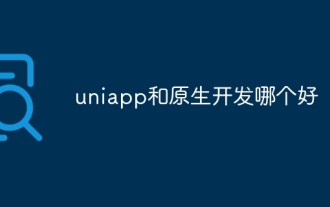
When choosing between UniApp and native development, you should consider development cost, performance, user experience, and flexibility. The advantages of UniApp are cross-platform development, rapid iteration, easy learning and built-in plug-ins, while native development is superior in performance, stability, native experience and scalability. Weigh the pros and cons based on specific project needs. UniApp is suitable for beginners, and native development is suitable for complex applications that pursue high performance and seamless experience.
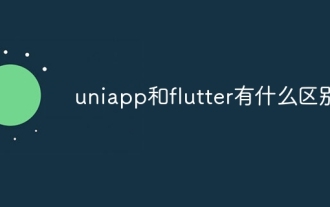
UniApp is based on Vue.js, and Flutter is based on Dart. Both support cross-platform development. UniApp provides rich components and easy development, but its performance is limited by WebView; Flutter uses a native rendering engine, which has excellent performance but is more difficult to develop. UniApp has an active Chinese community, and Flutter has a large and global community. UniApp is suitable for scenarios with rapid development and low performance requirements; Flutter is suitable for complex applications with high customization and high performance.
