


uniapp implements how to use the image cropping and compression library to implement image processing functions
uniapp implements how to use image cropping and compression libraries to implement image processing functions
When developing mobile applications, image processing requirements are often involved, such as image cropping and compression. In response to these needs, uniapp provides a wealth of plug-ins and components, allowing developers to easily implement image processing functions. This article will introduce how to use the image cropping and compression library in uniapp to implement image processing functions, and provide corresponding code examples.
- Picture cropping
Picture cropping refers to cutting out a part of the picture as needed. The commonly used image cropping plug-in in uniapp is "uniCropper". The following is a code example for using uniCropper to implement image cropping:
<template> <view> <image :src="imageSrc" mode="aspectFit" @tap="chooseImage"></image> <uni-cropper ref="cropper" :src="imageSrc" :styleType="styleType" :aspectRatio="aspectRatio" :minCropBoxWidth="minCropBoxWidth" :minCropBoxHeight="minCropBoxHeight" :maxCropBoxWidth="maxCropBoxWidth" :maxCropBoxHeight="maxCropBoxHeight" @ready="ready" @crop="crop" ></uni-cropper> <button @click="cropImage">裁剪</button> </view> </template> <script> export default { data() { return { imageSrc: '', styleType: 1, aspectRatio: 1, minCropBoxWidth: 50, minCropBoxHeight: 50, maxCropBoxWidth: 200, maxCropBoxHeight: 200 }; }, methods: { chooseImage() { uni.chooseImage({ success: (res) => { this.imageSrc = res.tempFilePaths[0]; } }); }, ready() { this.$refs.cropper.setDragMode('crop'); }, crop(e) { console.log('裁剪结果:', e.detail); }, cropImage() { this.$refs.cropper.crop(); } } }; </script>
In the above code example, the "uniCropper" component is first introduced and the "uni-cropper" tag is used in the template tag. By clicking the "chooseImage" method, you can select an image, and the selected image will be displayed in the image tag. Next, by specifying various attributes of the uni-cropper tag, the configuration of the cropping frame is achieved. After clicking the crop button, the cropImage method will be triggered, the $refs.cropper.crop() method will be called to perform cropping, and the cropping result will be obtained through the crop method.
- Image Compression
Image compression refers to saving storage space and improving network transmission speed by reducing the file size of images. The commonly used image compression plug-in in uniapp is "uni.compressImage". The following is a code example that uses uni.compressImage to implement image compression:
//选择图片并压缩 uni.chooseImage({ success: (res) => { let tempFilePath = res.tempFilePaths[0]; uni.compressImage({ src: tempFilePath, quality: 80, // 压缩质量,取值范围为0-100 success: (res) => { let compressedFilePath = res.tempFilePath; // 在这里可以处理压缩后的图片 console.log(compressedFilePath); } }); } });
In the above code example, an image is selected through the uni.chooseImage method, and the image is compressed through uni.compressImage. Compression quality can be set by specifying the quality attribute, which ranges from 0-100. After the compression is successful, the compressed image path can be obtained through the success callback function, and the image can be processed in the callback function.
Through the above code examples, we can implement the image cropping and compression functions in uniapp. According to specific needs, corresponding attributes and parameters can be configured to achieve different processing effects. The image processing function has a wide range of application scenarios in actual development. I hope this article will be helpful to you.
The above is the detailed content of uniapp implements how to use the image cropping and compression library to implement image processing functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


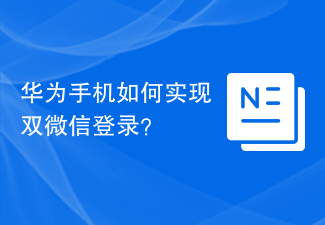
How to implement dual WeChat login on Huawei mobile phones? With the rise of social media, WeChat has become one of the indispensable communication tools in people's daily lives. However, many people may encounter a problem: logging into multiple WeChat accounts at the same time on the same mobile phone. For Huawei mobile phone users, it is not difficult to achieve dual WeChat login. This article will introduce how to achieve dual WeChat login on Huawei mobile phones. First of all, the EMUI system that comes with Huawei mobile phones provides a very convenient function - dual application opening. Through the application dual opening function, users can simultaneously
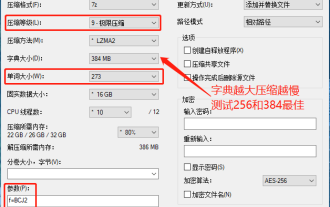
I found that the compressed package downloaded from a download website will be larger than the original compressed package after decompression. The difference is tens of Kb for a small one and several dozen Mb for a large one. If it is uploaded to a cloud disk or paid space, it does not matter if the file is small. , if there are many files, the storage cost will be greatly increased. I studied it specifically and can learn from it if necessary. Compression level: 9-Extreme compression Dictionary size: 256 or 384, the more compressed the dictionary, the slower it is. The compression rate difference is larger before 256MB, and there is no difference in compression rate after 384MB. Word size: maximum 273 Parameters: f=BCJ2, test and add parameter compression rate will be higher
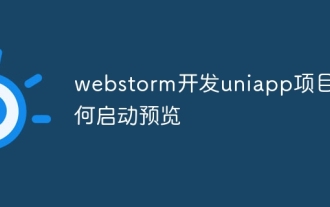
Steps to launch UniApp project preview in WebStorm: Install UniApp Development Tools plugin Connect to device settings WebSocket launch preview
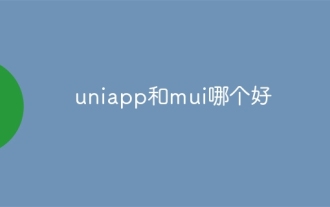
Generally speaking, uni-app is better when complex native functions are needed; MUI is better when simple or highly customized interfaces are needed. In addition, uni-app has: 1. Vue.js/JavaScript support; 2. Rich native components/API; 3. Good ecosystem. The disadvantages are: 1. Performance issues; 2. Difficulty in customizing the interface. MUI has: 1. Material Design support; 2. High flexibility; 3. Extensive component/theme library. The disadvantages are: 1. CSS dependency; 2. Does not provide native components; 3. Small ecosystem.
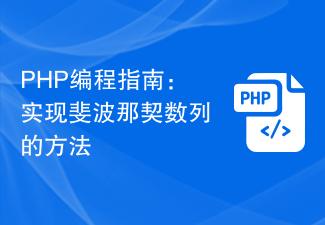
The programming language PHP is a powerful tool for web development, capable of supporting a variety of different programming logics and algorithms. Among them, implementing the Fibonacci sequence is a common and classic programming problem. In this article, we will introduce how to use the PHP programming language to implement the Fibonacci sequence, and attach specific code examples. The Fibonacci sequence is a mathematical sequence defined as follows: the first and second elements of the sequence are 1, and starting from the third element, the value of each element is equal to the sum of the previous two elements. The first few elements of the sequence
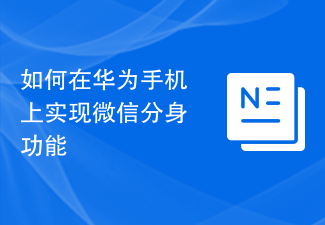
How to implement the WeChat clone function on Huawei mobile phones With the popularity of social software and people's increasing emphasis on privacy and security, the WeChat clone function has gradually become the focus of people's attention. The WeChat clone function can help users log in to multiple WeChat accounts on the same mobile phone at the same time, making it easier to manage and use. It is not difficult to implement the WeChat clone function on Huawei mobile phones. You only need to follow the following steps. Step 1: Make sure that the mobile phone system version and WeChat version meet the requirements. First, make sure that your Huawei mobile phone system version has been updated to the latest version, as well as the WeChat App.
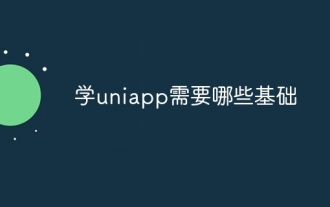
uniapp development requires the following foundations: front-end technology (HTML, CSS, JavaScript) mobile development knowledge (iOS and Android platforms) Node.js other foundations (version control tools, IDE, mobile development simulator or real machine debugging experience)
