


How to use annotation functions in Java for custom annotations and metadata processing
How to use annotation functions in Java for custom annotations and metadata processing
Introduction:
In Java programming, annotation (Annotation) is a Special syntax constructs that attach additional metadata to the code and are processed by compilers, interpreters, or other tools. An annotation function is a special annotation that can be used to mark functions, methods, or method parameters, and these annotations can be accessed and processed through the reflection mechanism at runtime. This article will introduce how to use annotation functions in Java for custom annotations and metadata processing, and provide specific code examples.
1. Understand the basic concepts of annotation functions
Annotation functions are a feature of the Java language that allow developers to add additional metadata information to the code for processing at runtime. The annotation function consists of two parts: annotation definition and annotation processing. Annotation definition is an annotation type customized by developers. Java meta-annotations (such as @Target, @Retention, @Documented, etc.) can be used to limit the usage scope and life cycle of annotations. Annotation processing is the process of accessing and processing annotation information through the reflection mechanism at runtime. Developers can customize annotation processors to perform specific processing logic.
2. Custom annotation type
1. Define annotation type
Use Java's @interface keyword to define an annotation type. The name of the annotation type should start with a capital letter and usually starts with " Annotation" at the end to distinguish it from other classes. Some elements (member variables) can be defined in annotation types, and these elements can have default values and can be assigned values when using annotations. The following is a simple annotation type definition example:
1 2 3 4 5 6 7 8 |
|
In the above code, we use the @Retention and @Target meta-annotations to specify the life cycle and usage scope of the annotation. Two elements are defined in the MyAnnotation annotation type: a value of type String and an intValue of type int, and both elements have default values.
2. Use annotation types
After defining the annotation type, we can use it in the code to mark methods, functions or method parameters. When using annotations, you can assign values to annotation elements or use default values. The following is an example of using annotation types:
1 2 3 4 |
|
In the above code, we use the @MyAnnotation annotation to mark the myMethod method, and assign the values and intValue to "Hello" and 100 respectively.
3. Custom annotation processor
In Java, you can use the reflection mechanism to access and process annotation information to implement a custom annotation processor. The following is a simple annotation processor example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
In the above code, we define an AnnotationProcessor class, whose processAnnotations method can handle methods with @MyAnnotation annotations. Through reflection, we can obtain the annotation instance and access the annotation's element value.
4. Use annotation processors for metadata processing
Using custom annotation processors and annotation types, we can perform metadata processing on methods with specific annotations at runtime. The following is an example of using an annotation processor for metadata processing:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
In the above code, we first call the AnnotationProcessor.processAnnotations method and pass in an object with annotations (MyClass). In the annotation processor, we can access the method annotated with @MyAnnotation and output the value of the annotation element.
Conclusion:
Annotation functions are an important feature in Java programming. They can add additional metadata information to the code and can be accessed and processed through the reflection mechanism at runtime. This article describes how to use annotation functions in Java for custom annotations and metadata processing, and provides specific code examples. By learning and mastering the use of annotation functions, we can improve the readability, maintainability and reusability of the code.
The above is the detailed content of How to use annotation functions in Java for custom annotations and metadata processing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
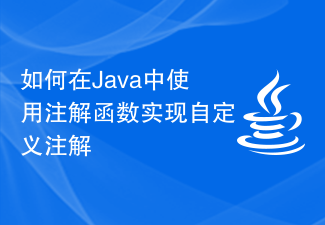
How to use annotation functions in Java to implement custom annotations. Annotation is a special syntax element in Java that can be used to add metadata information to the code for parsing and processing at runtime. Java provides some predefined annotations (such as @Override, @Deprecated, etc.), and also supports user-defined annotations. In some scenarios, using custom annotations can make the code more concise and readable. This article will introduce how to use
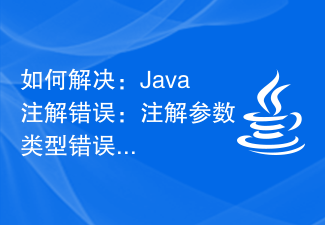
How to solve: Java annotation error: Wrong annotation parameter type Introduction: In Java development, annotation (Annotation) is a form of metadata used to add additional information to program elements (classes, methods, fields, etc.). However, sometimes we may encounter issues with annotation parameters being of the wrong type, which can lead to compilation errors or runtime exceptions. This article will introduce how to solve Java annotation parameter type errors and provide code examples to help readers better understand. Understanding annotation parameter type error: Annotation parameter type error
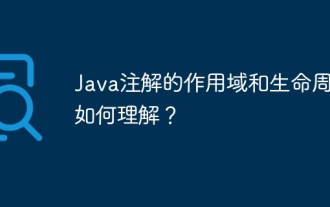
The scope of an annotation determines which parts of the code they apply to, while the lifetime describes how long they live in the code. The scope has element level, declaration type level and code block level, and the life cycle is divided into compile time, class loading time and run time. The life cycle of annotations includes being added to the class file during compilation, processed by the JVM when the class is loaded, and accessible through reflection at runtime.
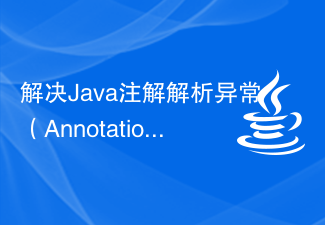
Methods to solve Java annotation parsing exception (AnnotationParsingException) Introduction: In Java development, annotations have become a very important technology, which can describe various information in the program by adding metadata to the source code. In the process of using annotations, sometimes we may encounter an AnnotationParsingException exception. This exception represents an error that occurs when parsing annotations. This article will explain how to solve this
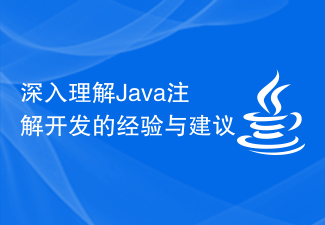
In-depth understanding of Java annotation development experience and suggestions. With the development of the Java language, annotations have become an indispensable part of Java development. As a kind of metadata, annotations can add additional descriptive information to the code and help developers better understand the code logic. At the same time, annotations can also be processed during compilation and runtime to achieve automated functions. In daily Java development, we often use annotations. However, to deeply understand and effectively apply annotations, you need to master a
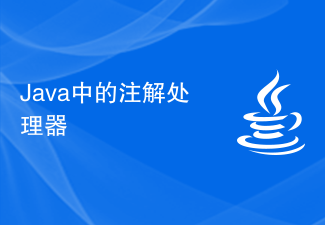
Annotation Processor in Java An annotation processor in Java is a tool that can detect and process annotations in Java code. Using annotation processors can enhance compile-time checking, generate additional code, and even modify existing code, thereby improving code readability, maintainability, and reusability. Annotation processors are usually written in Java rather than interpreted and executed at runtime. This provides a lot of convenience for the annotation processor, such as the use of a richer Java type system, object-oriented features and
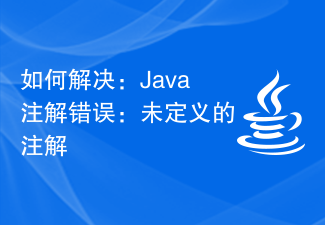
How to solve: Java annotation error: undefined annotation. In the process of using Java development, annotation is a very common technical means that can be used to add some additional information or behavior to the code. However, sometimes we may encounter an error: undefined annotation. This problem will cause the program to not work properly when compiling or running, so it is very important to solve this error. This article will introduce some methods to solve the undefined annotation error and provide some code examples. 1. Check the annotation guide package. When we use a self-
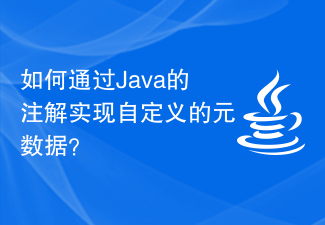
How to implement custom metadata through Java annotations? Introduction: During the Java development process, we often need to add some additional information to elements such as classes, methods, attributes, etc. for processing at runtime. Java's annotation (Annotation) mechanism provides us with a flexible way to implement customized metadata, allowing us to more easily add and use additional information during the coding process. This article will introduce how to implement customized metadata through Java's annotation mechanism, and give the corresponding
