


How to use JavaScript to achieve the up and down sliding switching effect of images while adding zoom and fade animations?
JavaScript How to achieve the up and down sliding switching effect of images while adding zoom and fade animations?
In web design, image switching effects are often used to improve user experience. Among these switching effects, sliding up and down, zooming and fading animations are relatively common and attractive. This article will introduce how to use JavaScript to achieve the combination of these three animation effects.
First, we need to use HTML to build a basic web page structure, which contains the picture elements to be displayed. The following is an example HTML code:
<!DOCTYPE html> <html> <head> <title>图片切换效果</title> <style> .container { position: relative; width: 500px; height: 300px; overflow: hidden; } .slide { position: absolute; display: none; width: 100%; height: 100%; } </style> </head> <body> <div class="container"> <img class="slide" src="image1.jpg"> <img class="slide" src="image2.jpg"> <img class="slide" src="image3.jpg"> </div> <script src="main.js"></script> </body> </html>
In the above code, we use a container div containing three image elements, each of which has the class "slide". Among them, the container class defines the style of the container, and the slide class defines the style of the picture element. By setting the overflow attribute of the container to hidden, the hiding effect of the part of the picture element beyond the container is achieved.
Next, we need to use JavaScript to achieve the up and down sliding switching, scaling and fade-in and fade-out animation effects of the image. The following is an example JavaScript code:
window.addEventListener('DOMContentLoaded', function() { var slides = document.querySelectorAll('.slide'); var currentIndex = 0; function showSlide(index) { // 隐藏当前显示的图片 slides[currentIndex].style.display = 'none'; // 显示指定索引的图片 slides[index].style.display = 'block'; // 设置当前索引 currentIndex = index; } function animateSlide(index) { var slide = slides[index]; // 先缩小图片 slide.style.transform = 'scale(0)'; slide.style.opacity = 0; // 等缩放动画完成后,再展示出来 setTimeout(function() { slide.style.transition = 'transform 0.5s, opacity 0.5s'; slide.style.transform = 'scale(1)'; slide.style.opacity = 1; }, 100); } function nextSlide() { var nextIndex = currentIndex + 1; if (nextIndex >= slides.length) { nextIndex = 0; } showSlide(nextIndex); animateSlide(nextIndex); } // 每隔3秒切换到下一张图片 setInterval(nextSlide, 3000); });
In the above code, we first obtain all image elements with slide class through document.querySelectorAll('.slide') and save them in the slides variable. currentIndex is used to record the currently displayed image index.
Then the showSlide function is defined to display the picture at the specified index. In the function, we set the display attribute of the currently displayed image element to 'none' to hide it, and set the display attribute of the image element at the specified index to 'block' to display it.
The animateSlide function is used to achieve the zoom and fade effects of images. In the function, we first set the transform and opacity properties of the image element to a reduced state and a transparency of 0. Then delay 0.1 seconds through the setTimeout function, and set the transition, transform and opacity attributes of the image element to the state of magnification and transparency to 1. Due to the setting of the transition attribute, there will be a transition effect during the fade-in and fade-out process.
Finally, the picture switching is realized through the nextSlide function. In the function, we first calculate the index of the next picture, display it and add animation effects through the showSlide function and animateSlide function respectively.
In the DOMContentLoaded event of the window object, we perform the above operations. And set the timer to automatically switch to the next picture every 3 seconds through the setInterval function.
Combined with the above HTML and JavaScript code, we have achieved the up and down sliding switching effect of the image, and added the zoom and fade animation effects. In this way, the switching of pictures is made more vivid and attractive, providing users with a better experience in web browsing. Note that the image path in the example needs to be adjusted according to the actual situation.
The above is the detailed content of How to use JavaScript to achieve the up and down sliding switching effect of images while adding zoom and fade animations?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


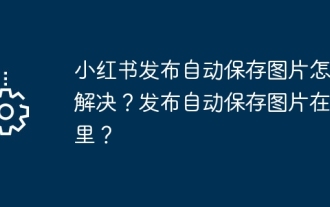
With the continuous development of social media, Xiaohongshu has become a platform for more and more young people to share their lives and discover beautiful things. Many users are troubled by auto-save issues when posting images. So, how to solve this problem? 1. How to solve the problem of automatically saving pictures when publishing on Xiaohongshu? 1. Clear the cache First, we can try to clear the cache data of Xiaohongshu. The steps are as follows: (1) Open Xiaohongshu and click the "My" button in the lower right corner; (2) On the personal center page, find "Settings" and click it; (3) Scroll down and find the "Clear Cache" option. Click OK. After clearing the cache, re-enter Xiaohongshu and try to post pictures to see if the automatic saving problem is solved. 2. Update the Xiaohongshu version to ensure that your Xiaohongshu
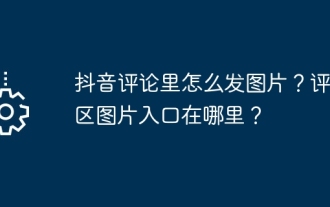
With the popularity of Douyin short videos, user interactions in the comment area have become more colorful. Some users wish to share images in comments to better express their opinions or emotions. So, how to post pictures in TikTok comments? This article will answer this question in detail and provide you with some related tips and precautions. 1. How to post pictures in Douyin comments? 1. Open Douyin: First, you need to open Douyin APP and log in to your account. 2. Find the comment area: When browsing or posting a short video, find the place where you want to comment and click the "Comment" button. 3. Enter your comment content: Enter your comment content in the comment area. 4. Choose to send a picture: In the interface for entering comment content, you will see a "picture" button or a "+" button, click
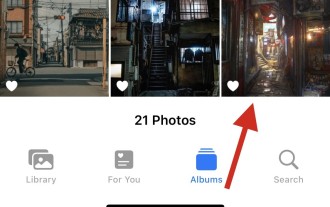
Apple's recent iPhones capture memories with crisp detail, saturation and brightness. But sometimes, you may encounter some issues that may cause the image to look less clear. While autofocus on iPhone cameras has come a long way, allowing you to take photos quickly, the camera can mistakenly focus on the wrong subject in certain situations, making the photo blurry in unwanted areas. If your photos on your iPhone look out of focus or lack sharpness overall, the following post should help you make them sharper. How to Make Pictures Clearer on iPhone [6 Methods] You can try using the native Photos app to clean up your photos. If you want more features and options
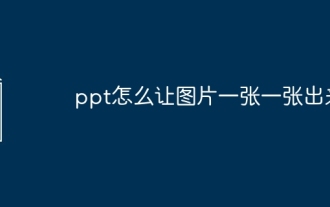
In PowerPoint, it is a common technique to display pictures one by one, which can be achieved by setting animation effects. This guide details the steps to implement this technique, including basic setup, image insertion, adding animation, and adjusting animation order and timing. Additionally, advanced settings and adjustments are provided, such as using triggers, adjusting animation speed and order, and previewing animation effects. By following these steps and tips, users can easily set up pictures to appear one after another in PowerPoint, thereby enhancing the visual impact of the presentation and grabbing the attention of the audience.
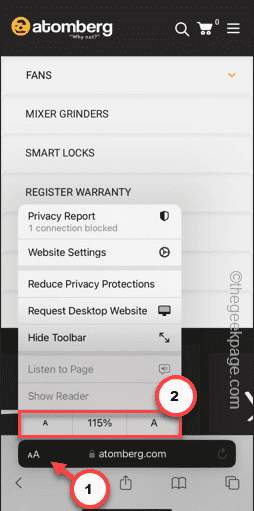
If you don't have control over the zoom level in Safari, getting things done can be tricky. So if Safari looks zoomed out, that might be a problem for you. Here are a few ways you can fix this minor zoom issue in Safari. 1. Cursor magnification: Select "Display" > "Cursor magnification" in the Safari menu bar. This will make the cursor more visible on the screen, making it easier to control. 2. Move the mouse: This may sound simple, but sometimes just moving the mouse to another location on the screen may automatically return it to normal size. 3. Use Keyboard Shortcuts Fix 1 – Reset Zoom Level You can control the zoom level directly from the Safari browser. Step 1 – When you are in Safari
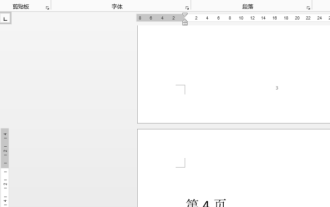
When we use word documents to edit files, sometimes there are many pages. We want to display them side by side and check the overall effect. However, because we don’t know how to operate, we often need to scroll for a long time to view page by page. I don’t know if you have ever encountered a similar situation. In fact, we can easily solve it at this time as long as we learn how to set the word zoom pages side by side. Below, let’s take a look and learn together. First, we create and open a new page in the Word document, and then enter some simple content to make it easier to distinguish. 2. For example, if we want to realize word zoom and side-by-side display, we need to find [View] in the menu bar, and then select [Multiple Pages] in the view tool options, as shown in the figure below: 3. Find [Multiple Pages] and click,
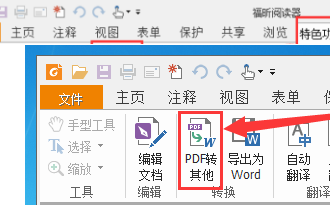
Are you also using Foxit PDF Reader software? So do you know how Foxit PDF Reader converts pdf documents into jpg images? The following article brings you how Foxit PDF Reader converts pdf documents into jpg images. For those who are interested in the method of converting jpg images, please come and take a look below. First start Foxit PDF Reader, then find "Features" on the top toolbar, and then select the "PDF to Others" function. Next, open a web page called "Foxit PDF Online Conversion". Click the "Login" button on the upper right side of the page to log in, and then turn on the "PDF to Image" function. Then click the upload button and add the pdf file you want to convert into an image. After adding it, click "Start Conversion"
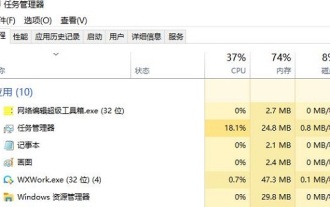
Some netizens found that when they opened the browser web page, the pictures on the web page could not be loaded for a long time. What happened? I checked that the network is normal, so where is the problem? The editor below will introduce to you six solutions to the problem that web page images cannot be loaded. Web page images cannot be loaded: 1. Internet speed problem The web page cannot display images. It may be because the computer's Internet speed is relatively slow and there are more softwares opened on the computer. And the images we access are relatively large, which may be due to loading timeout. As a result, the picture cannot be displayed. You can turn off the software that consumes more network speed. You can go to the task manager to check. 2. Too many visitors. If the webpage cannot display pictures, it may be because the webpages we visited were visited at the same time.
