How to use generic functions to define generic types and methods in Java
How to use generic functions to define generic types and methods in Java
Generics are a powerful feature in Java that can be provided when writing code Greater flexibility and reusability. A generic function is a function that can specify parameter types and can accept different types of parameters as needed. This article will introduce how to use generic functions to define generic types and methods in Java, and provide specific code examples.
- Definition of generic functions
In Java, we can use generic functions to define functions with generic types. To define a generic function, we need to add angle brackets before the function name and then define the generic type within the brackets. For example, the following is the definition of a simple generic function:
public <T> void printArray(T[] array) { for (T element : array) { System.out.println(element); } }
In the above example, the function is named printArray, which accepts a generic array as a parameter and loops through each element in the array. output.
- Call of generic types
When using generic functions, we can specify specific types as parameters, or we can automatically infer their types based on the parameters passed in. . The following are two ways to call the above generic function:
// 指定类型为Integer Integer[] intArray = {1, 2, 3, 4, 5}; printArray(intArray); // 自动推断类型为String String[] stringArray = {"Hello", "World"}; printArray(stringArray);
In the above example, we first defined an integer array and a string array, and then called the printArray function respectively and passed in the corresponding parameters. Since the printArray function is a generic function, it can automatically infer the generic type based on the type of parameters passed in.
- Definition of generic methods
In addition to defining generic functions, we can also use generic methods in classes. A generic method refers to a method with a generic type defined in the method. The following is the definition of a simple generic method:
public <T> T getLastElement(T[] array) { if (array.length == 0) { return null; } return array[array.length - 1]; }
In the above example, the getLastElement method accepts a generic array as a parameter and returns the last element in the array. The generic type T is used before the return type of the method, indicating that the method can return any type of value.
- Call of generic methods
When using generic methods, we can specify specific types as parameters as needed. The following is an example of calling the above generic method:
Integer[] intArray = {1, 2, 3, 4, 5}; String[] stringArray = {"Hello", "World"}; // 调用getLastElement方法并指定类型为Integer Integer lastInt = getLastElement(intArray); System.out.println("Last element of intArray: " + lastInt); // 调用getLastElement方法并指定类型为String String lastString = getLastElement(stringArray); System.out.println("Last element of stringArray: " + lastString);
In the above example, we called the getLastElement method respectively and specified different types as parameters. According to the parameter type passed in, the getLastElement method can return the corresponding type of value as needed.
Summary:
This article introduces how to use generic functions to define generic types and methods in Java, and provides specific code examples. Generic functions and methods can greatly improve the flexibility and reusability of code, allowing us to write more general code. By learning and using generics, you can handle different types of data more efficiently in Java programming.
The above is the detailed content of How to use generic functions to define generic types and methods in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
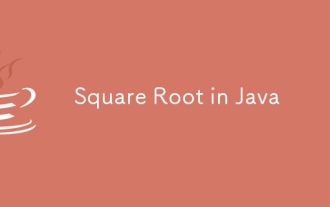
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
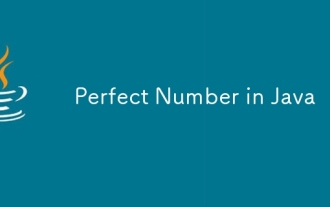
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
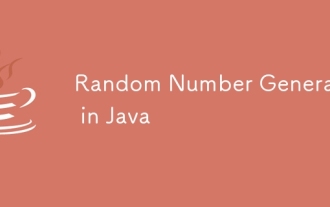
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
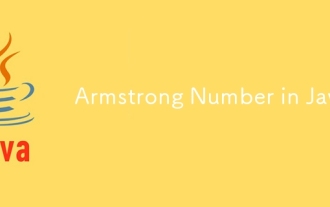
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
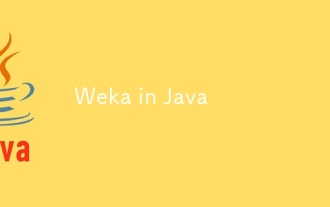
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
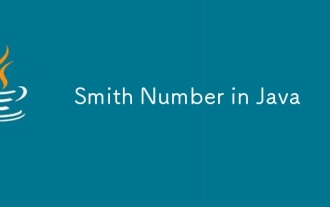
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
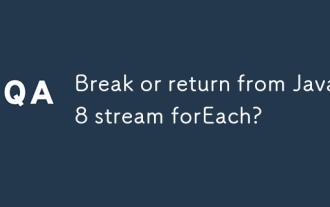
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
