How to use JavaScript to achieve a web page typewriter effect?
How to use JavaScript to achieve a web page typewriter effect?
In web design, the Typewriter Effect is a common dynamic effect that can add some fun and interactivity to the web page. This article will introduce how to use JavaScript to achieve a web page typewriter effect and provide specific code examples.
Before we start writing the code for the typewriter effect, we first need to prepare a text container to display the typewriter effect. It can be an HTML element such as <div>
or <span>
.
HTML code example:
<div id="typewriter"></div>
Next, we start writing JavaScript code.
First, we need to define a JavaScript function that will receive a string as a parameter and display the string verbatim in a text container. The code is as follows:
function typeWriter(text) { let i = 0; const speed = 100; // 打字速度(单位:毫秒) const container = document.getElementById("typewriter"); // 获取文本容器元素 container.innerHTML = ""; // 清空容器 // 创建定时器,每隔一定时间将下一个字符添加到容器中 const timer = setInterval(function() { // 判断是否已经到达字符串的末尾 if (i >= text.length) { clearInterval(timer); // 清除定时器 return; } // 将字符添加到容器中 container.innerHTML += text.charAt(i); i++; }, speed); }
In the above code, we first define a i
variable to record the number of characters that have been displayed. Then, we get the text container element through the getElementById
method and assign it to the container
variable. Next, we clear the contents of the container to ensure that each display of text starts from a blank state.
Next, we create a timer by using the setInterval
method. The timer will execute the function every speed
milliseconds. Inside the function, we first determine whether the complete string has been displayed, and if so, clear the timer and return. Otherwise, the next character of the string is added to the container and the value of the i
variable is updated.
Now, we have completed the function of the typewriter effect. Next, we can call the function elsewhere in the code, passing in the string we want to display as a parameter.
For example, we can automatically display a welcome message after the page is loaded:
window.onload = function() { const message = "欢迎访问我的网页!"; typeWriter(message); };
When the page is loaded, the typeWriter
function will automatically start displaying the string "Welcome" Visit my page!".
In addition to automatic execution, we can also trigger typewriter effects based on user operations. For example, when the user clicks a button, the execution of the function is triggered by binding the click event.
HTML code example:
<button id="start">开始打字机</button>
JavaScript code example:
const startButton = document.getElementById("start"); startButton.addEventListener("click", function() { const message = "这是一个打字机效果的例子。"; typeWriter(message); });
When the user clicks the "Start Typewriter" button, the typewriter effect will start displaying the string "This is a typewriter" Examples of effects."
Through the above code examples, we learned how to use JavaScript to implement the typewriter effect on web pages, and provided several ways to trigger the typewriter effect. The code can be further optimized and expanded based on specific project needs and design requirements. Hope this article helps you!
The above is the detailed content of How to use JavaScript to achieve a web page typewriter effect?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


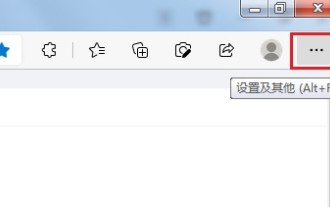
How to send web pages to the desktop as a shortcut in Edge browser? Many of our users want to display frequently used web pages on the desktop as shortcuts for the convenience of directly opening access pages, but they don’t know how to do it. In response to this problem, the editor of this issue will share the solution with the majority of users. , let’s take a look at the content shared in today’s software tutorial. The shortcut method of sending web pages to the desktop in Edge browser: 1. Open the software and click the "..." button on the page. 2. Select "Install this site as an application" in "Application" from the drop-down menu option. 3. Finally, click it in the pop-up window
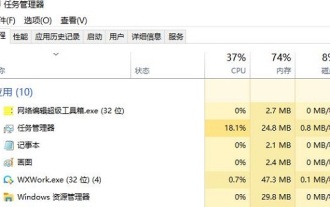
Some netizens found that when they opened the browser web page, the pictures on the web page could not be loaded for a long time. What happened? I checked that the network is normal, so where is the problem? The editor below will introduce to you six solutions to the problem that web page images cannot be loaded. Web page images cannot be loaded: 1. Internet speed problem The web page cannot display images. It may be because the computer's Internet speed is relatively slow and there are more softwares opened on the computer. And the images we access are relatively large, which may be due to loading timeout. As a result, the picture cannot be displayed. You can turn off the software that consumes more network speed. You can go to the task manager to check. 2. Too many visitors. If the webpage cannot display pictures, it may be because the webpages we visited were visited at the same time.
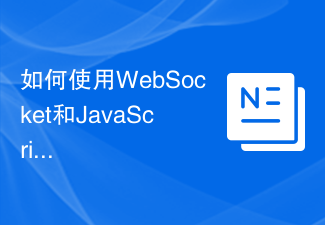
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
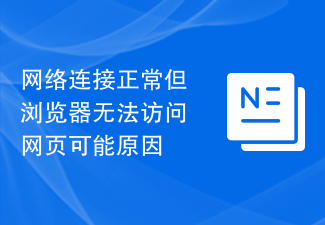
The browser cannot open the web page but the network is normal. There are many possible reasons. When this problem occurs, we need to investigate step by step to determine the specific cause and solve the problem. First, determine whether the webpage cannot be opened is limited to a specific browser or whether all browsers cannot open the webpage. If only one browser cannot open the web page, you can try to use other browsers, such as Google Chrome, Firefox, etc., for testing. If other browsers are able to open the page correctly, the problem is most likely with that specific browser, possibly
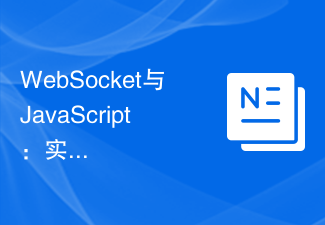
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
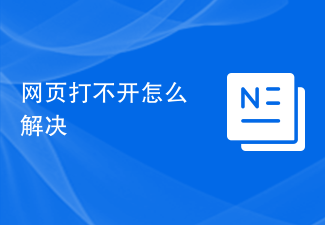
How to solve the problem of web pages not opening With the rapid development of the Internet, people increasingly rely on the Internet to obtain information, communicate and entertain. However, sometimes we encounter the problem that the web page cannot be opened, which brings us a lot of trouble. This article will introduce you to some common methods to help solve the problem of web pages not opening. First, we need to determine why the web page cannot be opened. Possible reasons include network problems, server problems, browser settings problems, etc. Here are some solutions: Check network connection: First, we need
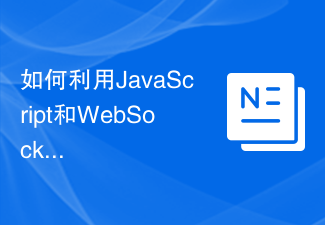
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order

Executing PHP code in a web page requires ensuring that the web server supports PHP and is properly configured. PHP can be opened in three ways: * **Server environment:** Place the PHP file in the server root directory and access it through the browser. * **Integrated Development Environment: **Place PHP files in the specified web root directory and access them through the browser. * **Remote Server:** Access PHP files hosted on a remote server via the URL address provided by the server.
