Getting started with must-memorize code in java
Java is a widely used programming language with the characteristics of cross-platform, security, portability, multi-threading, etc., so it is widely used in various software development fields. When learning Java programming, there are some key concepts and codes that Java beginners must master.
1. Basic data types and variables
The basic data types of Java include byte, short, int, long, float, double, boolean and char. These data types are Java The basics of programming, you need to be familiar with their uses and features. In addition, learn how to declare, assign, and access variables.
public class Main { public static void main(String[] args) { byte b = 1; short s = 2; int i = 3; long l = 4L; float f = 5.0f; double d = 6.0; boolean bool = true; char c = 'A'; } }
2. Operators and expressions
Operators in Java include arithmetic operators, relational operators, logical operators, assignment operators, etc. Familiarity with the usage and precedence of these operators is fundamental to writing Java code.
public class Main { public static void main(String[] args) { int a = 10; int b = 20; int c = a + b; int d = a * b; int e = a > b ? 1 : 0; } }
3. Control statements
Control statements are statements used to control the execution flow of the program, including conditional statements (if, else, switch), loop statements (for , while, do-while) and jump statements (break, continue, return), etc. Mastering the use of these control statements can help you write more complex programs.
public class Main { public static void main(String[] args) { int i = 1; while (i <= 10) { System.out.println(i); i++; } } }
4, Arrays and Strings
An array in Java is a container used to store multiple data of the same type. Learning how to declare arrays, create arrays, access array elements, and manipulate arrays is an essential skill in Java programming. Also, the string is Another important data type in Java requires mastering the operation methods and techniques of strings.
public class Main { public static void main(String[] args) { int[] arr = {1, 2, 3, 4, 5}; for (int i = 0; i < arr.length; i++) { System.out.println(arr[i]); } String str = "Hello, World!"; System.out.println(str.length()); } }
5. Object-oriented programming
Java is an object-oriented programming language, so understanding the basic concepts and principles of object-oriented programming is a must for Java beginners class. These concepts include classes, objects, inheritance, encapsulation, polymorphism, etc.
public class Main { public static void main(String[] args) { Animal dog = new Animal(); dog.eat(); Animal cat = new Cat(); cat.eat(); } } class Animal { public void eat() { System.out.println("Animal eating..."); } } class Cat extends Animal { public void eat() { System.out.println("Cat eating..."); } }
6. Exception handling
In Java programming, exception handling is an important link. Learning how to handle exceptions can improve the robustness of your program. Exception handling mechanisms in Java include try-catch-finally statement, throws keyword, custom exception class, etc.
public class Main { public static void main(String[] args) { try { int[] arr = new int[10]; for (int i = 0; i < arr.length; i++) { arr[i] = 10; } } catch (ArrayIndexOutOfBoundsException e) { System.out.println("Array index out of bounds"); } }
The above is the detailed content of Getting started with must-memorize code in java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


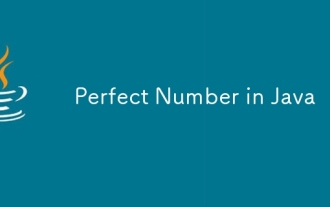
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
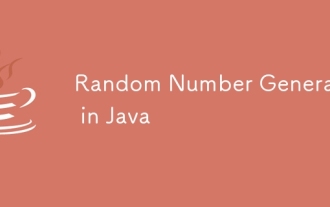
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
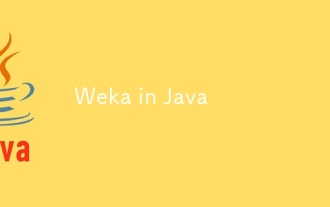
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
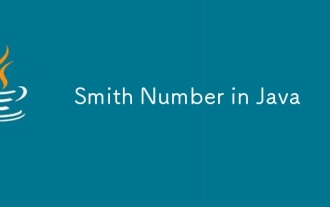
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
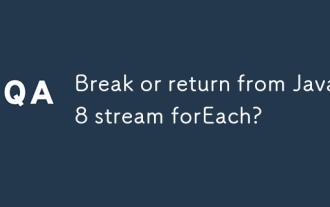
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
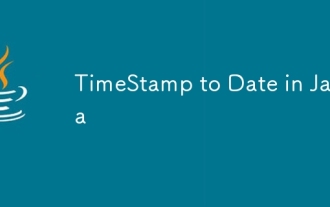
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
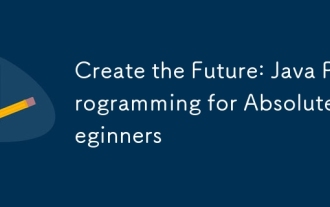
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
