How to use JavaScript to dynamically generate tables?
How to use JavaScript to dynamically generate tables?
In web development, tables are often used to display data or create forms for data entry. Using JavaScript can realize the function of dynamically generating tables, so that the table contents can be dynamically updated according to changes in data. This article will introduce in detail how to use JavaScript to dynamically generate tables through specific code examples.
1. HTML structure preparation
First, prepare a container in HTML to host the generated table. For example:
<div id="tableContainer"></div>
2. JavaScript code implementation
Next, we use JavaScript to dynamically generate tables. The specific implementation steps are as follows:
- Create table data
First, we need to prepare the data that needs to be displayed in the table. It can be existing data or data obtained from the backend. For the sake of simplicity, we first create a data for demonstration, as shown below:
var tableData = [ { name: '张三', age: 20, gender: '男' }, { name: '李四', age: 25, gender: '女' }, { name: '王五', age: 30, gender: '男' } ];
- Dynamic Generate table function
Next, we define a JavaScript function to dynamically generate a table. The specific implementation code is as follows:
function generateTable(tableData) { var container = document.getElementById('tableContainer'); var table = document.createElement('table'); var thead = document.createElement('thead'); var tbody = document.createElement('tbody'); // 生成表头 var theadRow = document.createElement('tr'); for (var key in tableData[0]) { var th = document.createElement('th'); th.appendChild(document.createTextNode(key)); theadRow.appendChild(th); } thead.appendChild(theadRow); table.appendChild(thead); // 生成表格内容 for (var i = 0; i < tableData.length; i++) { var tbodyRow = document.createElement('tr'); for (var key in tableData[i]) { var td = document.createElement('td'); td.appendChild(document.createTextNode(tableData[i][key])); tbodyRow.appendChild(td); } tbody.appendChild(tbodyRow); } table.appendChild(tbody); container.appendChild(table); }
- Call the dynamically generated table function
Finally, we call this function at the appropriate time, such as after the page is loaded or after the data is updated. . As shown below:
window.onload = function() { generateTable(tableData); };
At this point, we have completed the function of dynamically generating tables through JavaScript. When the page is loaded, you can see a table dynamically generated based on tableData
data in the tableContainer
container.
Summary
Using JavaScript to dynamically generate tables allows us to flexibly display, enter and edit table data according to data changes. The above is how to use JavaScript to dynamically generate tables. I hope it will be helpful to you.
The above is the detailed content of How to use JavaScript to dynamically generate tables?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


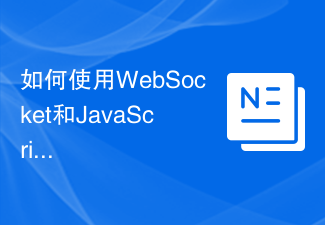
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
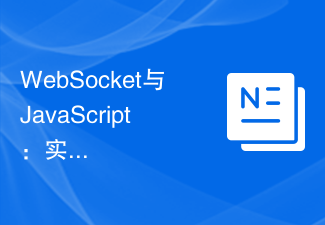
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
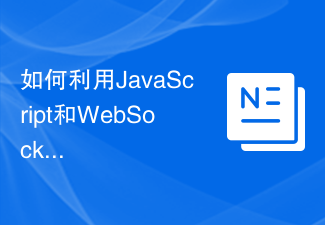
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
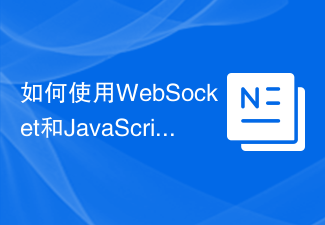
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
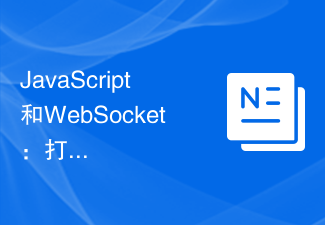
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
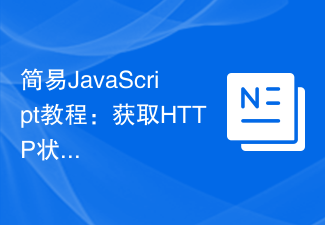
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
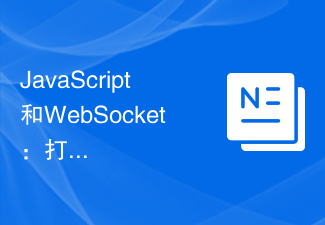
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
