


How to Achieve Infinite Scroll Loading More Content Using HTML, CSS, and jQuery
How to use HTML, CSS and jQuery to achieve infinite scrolling to load more content
In modern web design, we often encounter situations where a large amount of content needs to be loaded. In order to improve the user experience, the infinite scroll loading function is particularly important. This article will introduce how to use HTML, CSS and jQuery to achieve this functionality, and provide some specific code examples.
Infinite scroll loading means that when the user scrolls to the bottom of the page, more content is automatically loaded without the need to click the page turn or load button. This seamless loading method can greatly improve users' browsing efficiency and also bring them a better user experience.
First, we need to set up the basic skeleton structure in the HTML file. Assuming that our content is presented in the form of a list, we can use the <ul></ul>
and <li>
tags to set the list.
1 2 3 4 5 6 7 8 9 |
|
Next, we need to define some CSS styles to control the style of the list and the height of the scroll loading area.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
Now, we can add jQuery code to implement infinite scroll loading. First, we need to use the $(window).scroll()
method to listen to the scroll event of the page.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
In the above code, we trigger the event of loading more content by calculating the distance of the scroll bar from the bottom. When the scroll bar is still 100px from the bottom, it is considered that the user has scrolled to the bottom of the page and the function to load more content is triggered.
Finally, we need to define the loadMoreContent()
function to load more content.
1 2 3 4 5 6 7 8 9 10 11 12 |
|
In the loadMoreContent()
function, we can obtain new content through Ajax requests or other methods. For the sake of demonstration here, we use a simple loop to generate new content and then append it to the list.
Through the above code, we have successfully implemented the infinite scroll loading function. When users scroll to the bottom of the page, new content is automatically loaded and displayed in the list, achieving a seamless browsing experience.
Summary:
Through the combination of HTML, CSS and jQuery, we can easily realize the infinite scroll loading function. First, we set up the skeleton structure and CSS style of the page; then, we use jQuery to listen for scroll events, determine the scroll position and trigger the loading function; finally, we obtain new content through Ajax or other methods and append it to the page.
Infinite scroll loading is a common technical application. In this way, users can continuously load new content, thereby improving the user's browsing efficiency and user experience. I hope this article can help you when implementing similar functions.
The above is the detailed content of How to Achieve Infinite Scroll Loading More Content Using HTML, CSS, and jQuery. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


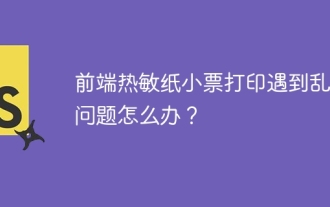
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
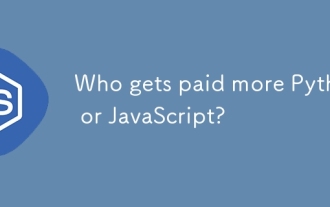
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
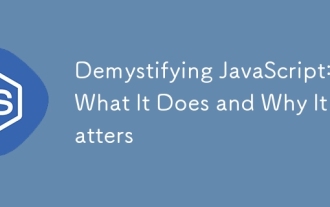
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
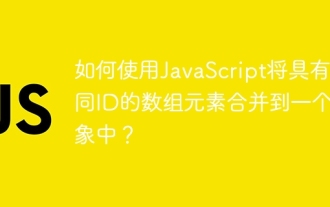
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
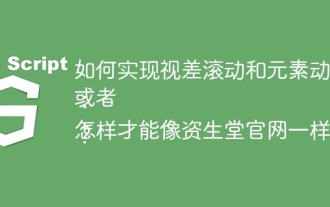
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
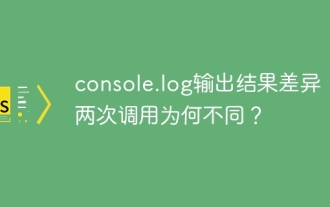
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
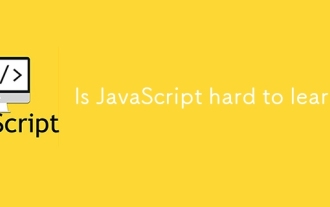
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
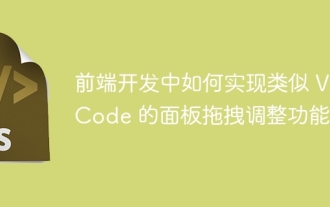
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
