How the uniapp application implements data storage and local caching
Uniapp is a cross-platform development framework that can be used to develop WeChat applets, H5 web pages and other mobile applications. Data storage and local caching are very important features during development. This article will introduce how to implement data storage and local caching in Uniapp and provide specific code examples.
1. Data Storage
There are many ways to implement data storage in Uniapp. Several common methods will be introduced below.
- Using Vue's data attribute
In Vue, we can use the data attribute to store data. In Uniapp, data storage can also be implemented in this way. For example:
<template> <view> <button @click="changeData">改变数据</button> <view>{{ myData }}</view> </view> </template> <script> export default { data() { return { myData: 'Hello Uniapp', } }, methods: { changeData() { this.myData = 'New Data' }, }, } </script>
- Using Vuex
Vuex is a state management tool for Vue and can also be used in Uniapp. Through Vuex, we can store data in the global store to facilitate access and modification in different components. For example:
// store.js import Vue from 'vue' import Vuex from 'vuex' Vue.use(Vuex) const store = new Vuex.Store({ state: { myData: 'Hello Uniapp', }, mutations: { changeData(state, payload) { state.myData = payload }, }, }) export default store
// main.js import Vue from 'vue' import App from './App' import store from './store' Vue.config.productionTip = false App.mpType = 'app' const app = new Vue({ store, ...App, }) app.$mount()
// MyComponent.vue <template> <view> <button @click="changeData">改变数据</button> <view>{{ myData }}</view> </view> </template> <script> export default { computed: { myData() { return this.$store.state.myData }, }, methods: { changeData() { this.$store.commit('changeData', 'New Data') }, }, } </script>
2. Local cache
To implement local cache in Uniapp, you can use the uni-app API to operate local storage. Commonly used APIs include uni.setStorageSync, uni.getStorageSync, uni.removeStorageSync, etc. The following is a specific example:
// 存储数据 uni.setStorageSync('myData', 'Hello Uniapp') // 获取数据 const data = uni.getStorageSync('myData') console.log(data) // 输出 Hello Uniapp // 移除数据 uni.removeStorageSync('myData')
In addition to synchronous APIs, Uniapp also provides asynchronous APIs, such as uni.setStorage, uni.getStorage, etc. Using asynchronous APIs can improve the responsiveness of the user interface. The following is an example of an asynchronous API:
// 存储数据 uni.setStorage({ key: 'myData', data: 'Hello Uniapp', success: function () { console.log('数据存储成功') }, }) // 获取数据 uni.getStorage({ key: 'myData', success: function (res) { console.log(res.data) // 输出 Hello Uniapp }, }) // 移除数据 uni.removeStorage({ key: 'myData', success: function () { console.log('数据移除成功') }, })
This article introduces the method of implementing data storage and local caching in Uniapp, and provides specific code examples. Developers can choose a method that suits them based on actual needs to implement data storage and local caching to improve application performance and user experience.
The above is the detailed content of How the uniapp application implements data storage and local caching. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


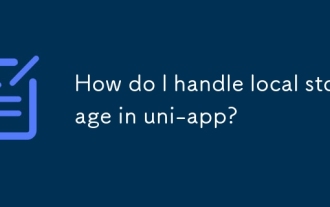
This article details uni-app's local storage APIs (uni.setStorageSync(), uni.getStorageSync(), and their async counterparts), emphasizing best practices like using descriptive keys, limiting data size, and handling JSON parsing. It stresses that lo

This article details making and securing API requests within uni-app using uni.request or Axios. It covers handling JSON responses, best security practices (HTTPS, authentication, input validation), troubleshooting failures (network issues, CORS, s
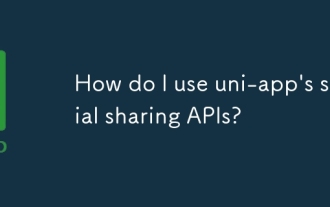
The article details how to integrate social sharing into uni-app projects using uni.share API, covering setup, configuration, and testing across platforms like WeChat and Weibo.
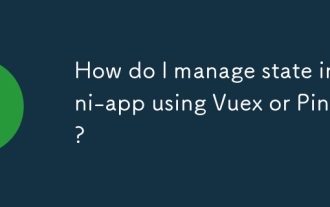
This article compares Vuex and Pinia for state management in uni-app. It details their features, implementation, and best practices, highlighting Pinia's simplicity versus Vuex's structure. The choice depends on project complexity, with Pinia suita
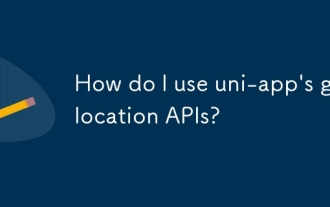
This article details uni-app's geolocation APIs, focusing on uni.getLocation(). It addresses common pitfalls like incorrect coordinate systems (gcj02 vs. wgs84) and permission issues. Improving location accuracy via averaging readings and handling

This article explains uni-app's easycom feature, automating component registration. It details configuration, including autoscan and custom component mapping, highlighting benefits like reduced boilerplate, improved speed, and enhanced readability.
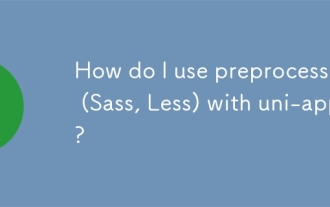
Article discusses using Sass and Less preprocessors in uni-app, detailing setup, benefits, and dual usage. Main focus is on configuration and advantages.[159 characters]
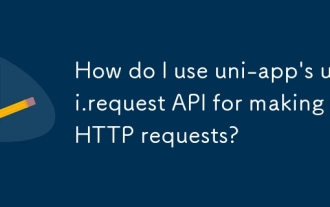
This article details uni.request API in uni-app for making HTTP requests. It covers basic usage, advanced options (methods, headers, data types), robust error handling techniques (fail callbacks, status code checks), and integration with authenticat
