


How to make a responsive vertical navigation using HTML, CSS and jQuery
How to use HTML, CSS and jQuery to make a responsive vertical navigation
The navigation menu is one of the important parts of the website, providing users with browsing and navigation Website functionality. How to make a responsive vertical navigation that can adapt to different screen sizes and devices has become a problem that must be solved. In this article, I will show you how to make a responsive vertical navigation using HTML, CSS, and jQuery.
First, we need to create a basic HTML structure, including the container and menu items of the navigation menu. We use the <nav></nav>
tag as the container for the navigation menu, and the <ul></ul>
and <li>
tags to create our menu items. The code is as follows:
<nav class="vertical-nav"> <ul> <li><a href="#">首页</a></li> <li><a href="#">关于我们</a></li> <li><a href="#">产品</a></li> <li><a href="#">联系我们</a></li> </ul> </nav>
Next, we will use CSS to style the navigation menu. We need to set the width, background color, text color, etc. of the navigation menu. At the same time, in order to achieve the effect of vertical arrangement, we also need to set the width and height of the menu items. The code is as follows:
.vertical-nav { width: 200px; background-color: #f1f1f1; } .vertical-nav ul { list-style-type: none; padding: 0; margin: 0; } .vertical-nav li { width: 100%; height: 40px; line-height: 40px; } .vertical-nav a { display: block; text-decoration: none; color: #333; padding: 0 20px; } .vertical-nav a:hover { background-color: #ccc; color: #fff; }
Now, we have completed the production of the basic vertical navigation menu. Next, we will use jQuery to make the menu items responsive.
First, we need to introduce the jQuery library file into HTML. You can download the latest version of the library file from jQuery's official website and introduce it in HTML using the <script>
tag. The code is as follows:
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
Then, we will use jQuery to add click events to expand or collapse the submenu when the menu item is clicked. The code is as follows:
$(document).ready(function() { $('.vertical-nav li').click(function() { $(this).find('ul').slideToggle(); }); });
In the above code, we first use the $(document).ready()
function to ensure that the jQuery code is executed after the document is loaded. Then, we use the .click()
function to bind the click event to the menu item. When a menu item is clicked, we use the .slideToggle()
function to show or hide the submenu.
Finally, we can also use CSS media queries to achieve responsive effects on the navigation menu. When the screen width is smaller than a certain value, we can hide the navigation menu and use a button to expand or collapse the menu. The code is as follows:
@media (max-width: 768px) { .vertical-nav { display: none; } .vertical-nav.toggle { display: block; background-color: #f1f1f1; padding: 10px; margin-bottom: 10px; text-align: center; } .vertical-nav.toggle:before { content: "0c9"; font-family: FontAwesome; font-size: 20px; } .vertical-nav.toggle.active:before { content: "00d"; } }
In the above code, we use @media (max-width: 768px)
to represent the style when the screen width is less than 768 pixels. In this media query, we hide the navigation menu and add a button to expand or collapse the menu. We also use the FontAwesome icon library to display a " " or "-" icon to indicate the expanded or collapsed state of the menu.
So far, we have completed the production of a responsive vertical navigation menu. Through the combination of HTML, CSS and jQuery, we realize the expansion or collapse effect of menu items, and provide responsive menu buttons when the screen width is less than a certain value. You can adjust and extend the code to suit different design and functional requirements.
The above is the detailed content of How to make a responsive vertical navigation using HTML, CSS and jQuery. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
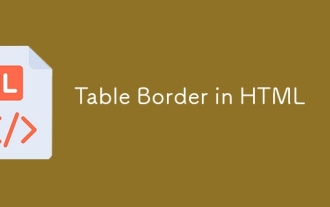
Guide to Table Border in HTML. Here we discuss multiple ways for defining table-border with examples of the Table Border in HTML.
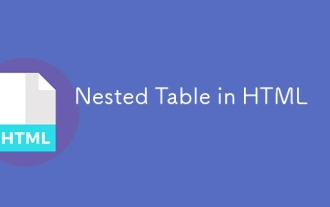
This is a guide to Nested Table in HTML. Here we discuss how to create a table within the table along with the respective examples.
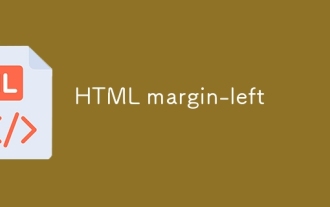
Guide to HTML margin-left. Here we discuss a brief overview on HTML margin-left and its Examples along with its Code Implementation.
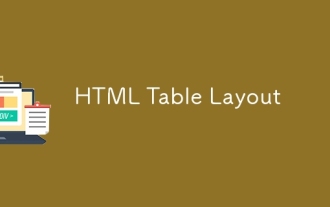
Guide to HTML Table Layout. Here we discuss the Values of HTML Table Layout along with the examples and outputs n detail.
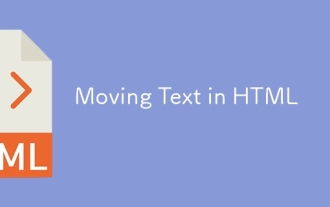
Guide to Moving Text in HTML. Here we discuss an introduction, how marquee tag work with syntax and examples to implement.
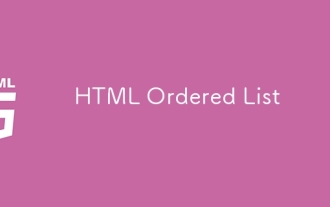
Guide to the HTML Ordered List. Here we also discuss introduction of HTML Ordered list and types along with their example respectively
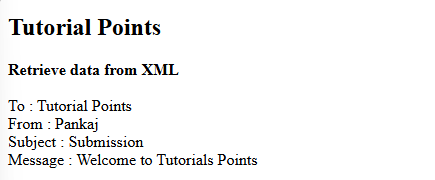
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
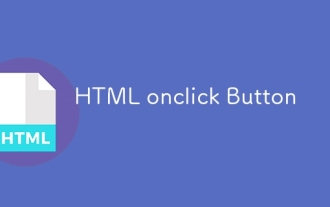
Guide to HTML onclick Button. Here we discuss their introduction, working, examples and onclick Event in various events respectively.
