


How to use ChatGPT PHP to implement multi-language intelligent chat function
How to use ChatGPT PHP to implement multi-language intelligent chat function
Introduction:
With the continuous development of artificial intelligence technology, intelligent chat robots have been used in various fields widely used. ChatGPT is an open source model based on deep learning technology that can achieve natural language processing and dialogue generation tasks. This article will introduce how to use ChatGPT PHP to implement multi-language intelligent chat function and provide specific code examples.
1. Install ChatGPT PHP
-
Create the composer.json file in the project root directory and add the following content:
{ "require" : { "openai/openai" : "dev-master" } }
Copy after login Run the following command to install ChatGPT PHP:
$ composer install
Copy after login
2. Create Chatbot class
Create a Chatbot.php file in the project and define a Chatbot class for implementing ChatGPT Interaction.
<?php require 'vendor/autoload.php'; use OpenAIOpenAI; class Chatbot { private $openai; public function __construct() { $this->openai = new OpenAI('YOUR_API_KEY'); } public function chat($message, $language = 'en') { $response = $this->openai->complete([ 'model' => 'gpt-3.5-turbo', 'prompt' => $this->getLanguagePrompt($language) . $message, 'temperature' => 0.7, 'max_tokens' => 100, 'stop' => [' '], ]); $chat_output = $response['choices'][0]['text']; // 处理ChatGPT生成的响应 // 示例代码省略 return $chat_output; } private function getLanguagePrompt($language) { // 根据语言选择合适的prompt // 示例代码省略 return ''; } }
3. Use the Chatbot class to implement multi-language chat function
- Create an index.php file in the project to implement the chat interface.
<?php require 'Chatbot.php'; $chatbot = new Chatbot(); $language = 'en'; // 默认语言为英语 if (isset($_POST['message']) && !empty($_POST['message'])) { $message = $_POST['message']; if (isset($_POST['language']) && !empty($_POST['language'])) { $language = $_POST['language']; } $response = $chatbot->chat($message, $language); } ?> <!DOCTYPE html> <html> <head> <title>Chatbot</title> </head> <body> <h1>Chatbot</h1> <form action="/" method="POST"> <label for="message">Message:</label> <input type="text" id="message" name="message" required><br><br> <label for="language">Language:</label> <select id="language" name="language"> <option value="en">English</option> <option value="zh">Chinese</option> <!-- 其他语言选项--> </select><br><br> <input type="submit" value="Send"> </form> <?php if (isset($response)) : ?> <h3>Response:</h3> <p><?php echo $response; ?></p> <?php endif; ?> </body> </html>
4. Use Chatbot for multi-language chat
Open the terminal, enter the project root directory, and run the following command to start the PHP built-in server:
$ php -S localhost:8000
Copy after login- Enter http://localhost:8000 in the browser to access the chat interface.
- Enter the message and select the language, and click the Send button. ChatGPT will generate a reply and display it on the interface.
Summary:
This article introduces how to use ChatGPT PHP to implement multi-language intelligent chat function. By introducing the Chatbot class, we can easily interact with ChatGPT and generate corresponding replies based on the language selected by the user. I hope this article helps you in the process of developing multi-language chat functionality.
The above are code examples in this article for reference.
The above is the detailed content of How to use ChatGPT PHP to implement multi-language intelligent chat function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
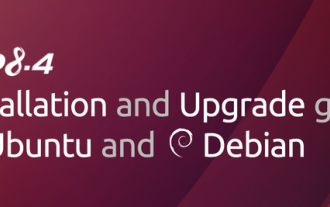
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
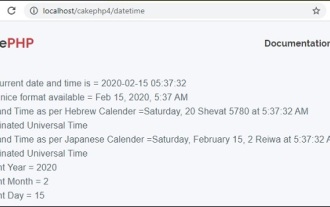
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
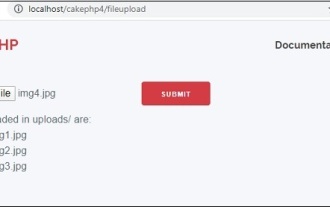
To work on file upload we are going to use the form helper. Here, is an example for file upload.
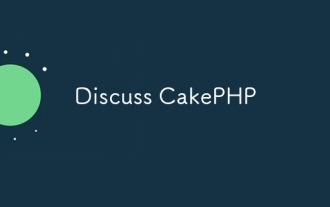
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
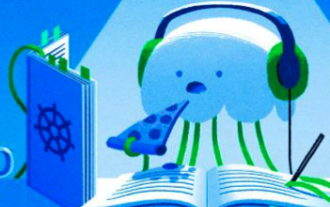
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
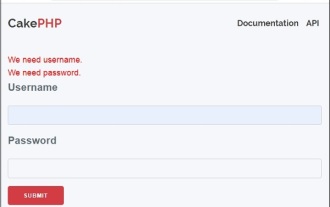
Validator can be created by adding the following two lines in the controller.
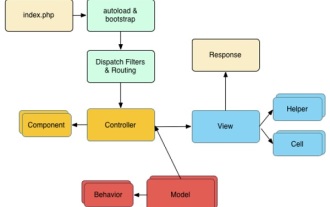
CakePHP is an open source MVC framework. It makes developing, deploying and maintaining applications much easier. CakePHP has a number of libraries to reduce the overload of most common tasks.
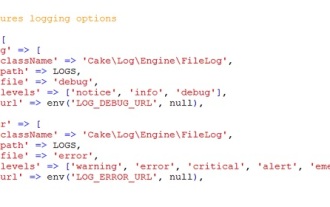
Logging in CakePHP is a very easy task. You just have to use one function. You can log errors, exceptions, user activities, action taken by users, for any background process like cronjob. Logging data in CakePHP is easy. The log() function is provide
