How to implement stock quotes and fund statistics in uniapp
uniapp is a cross-platform application framework developed based on Vue.js, which can quickly and efficiently develop mobile applications. It is a very common requirement to implement stock quotes and fund statistics in uniapp. Specific code examples will be given below to help you realize this function.
First, we need to obtain stock market data. In uniapp, you can obtain real-time stock market data by calling third-party APIs. The following is a code example for obtaining stock quotes:
// 导入uni-app的网络请求模块 import { request } from '@flyio/uni-app' // 获取股票行情数据 export function getStockQuotes() { return new Promise((resolve, reject) => { request({ method: 'GET', url: 'http://api.stockquotes.com/quotes', success: (res) => { resolve(res.data) }, fail: (err) => { reject(err) } }) }) }
In the above example, the @flyio/uni-app module of uni-app is used to send network requests and obtain stock quotes data. The specific request method and URL will be modified according to the actual situation.
Next, we need to implement the function of fund statistics. Fund statistics mainly count users' assets or transaction records, and perform corresponding calculations and displays. The following is a simple code example of fund statistics:
// 获取用户资产 export function getUserAssets() { return new Promise((resolve, reject) => { request({ method: 'GET', url: 'http://api.stockquotes.com/user/assets', success: (res) => { resolve(res.data) }, fail: (err) => { reject(err) } }) }) } // 获取用户交易记录 export function getUserTransactions() { return new Promise((resolve, reject) => { request({ method: 'GET', url: 'http://api.stockquotes.com/user/transactions', success: (res) => { resolve(res.data) }, fail: (err) => { reject(err) } }) }) } // 计算用户资金统计 export function calculateUserStatistics() { return new Promise((resolve, reject) => { Promise.all([getUserAssets(), getUserTransactions()]) .then(([assets, transactions]) => { // 进行资金统计计算 let totalAssets = 0 let totalTransactions = 0 // 对资产进行统计计算 assets.forEach(asset => { totalAssets += asset.value }) // 对交易记录进行统计计算 transactions.forEach(transaction => { totalTransactions += transaction.amount }) resolve({ totalAssets, totalTransactions }) }) .catch(err => { reject(err) }) }) }
In the above example, we use the getUserAssets() and getUserTransactions() functions to obtain the user's assets and transaction records respectively. Then use the Promise.all() function to merge the two asynchronous requests into a Promise object, and use the .then() and .catch() methods to handle the return result or error. In the calculateUserStatistics() function, we perform statistical calculations on the user's assets and transaction records and return the calculation results.
Finally, use the above function in the Vue component to display stock quotes and financial statistics:
<template> <div> <h1 id="股票行情">股票行情</h1> <ul> <li v-for="quote in stockQuotes" :key="quote.id"> {{quote.name}} - {{quote.price}} </li> </ul> <h1 id="资金统计">资金统计</h1> <p>总资产:{{statistics.totalAssets}}</p> <p>交易总额:{{statistics.totalTransactions}}</p> </div> </template> <script> import { getStockQuotes, calculateUserStatistics } from '@/api' export default { data() { return { stockQuotes: [], statistics: {} } }, mounted() { // 获取股票行情数据 getStockQuotes() .then(data => { this.stockQuotes = data }) .catch(err => { console.error(err) }) // 获取用户资金统计 calculateUserStatistics() .then(statistics => { this.statistics = statistics }) .catch(err => { console.error(err) }) } } </script>
In the above example, we obtain the stock quotes data by calling the getStockQuotes() function, and Save the data in stockQuotes array. Then call the calculateUserStatistics() function to obtain user fund statistics and save the data in the statistics object. Finally, use v-for instructions and data binding in the HTML template to display stock quotes and financial statistics.
The above are specific code examples to implement stock quotes and fund statistics in uniapp. Through the above examples, we can see that uniapp provides very convenient network request and data binding functions, which can help us quickly implement complex functions. I hope to be helpful!
The above is the detailed content of How to implement stock quotes and fund statistics in uniapp. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


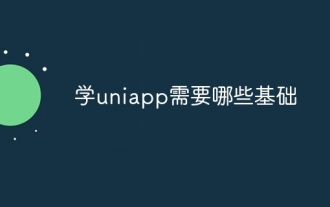
uniapp development requires the following foundations: front-end technology (HTML, CSS, JavaScript) mobile development knowledge (iOS and Android platforms) Node.js other foundations (version control tools, IDE, mobile development simulator or real machine debugging experience)
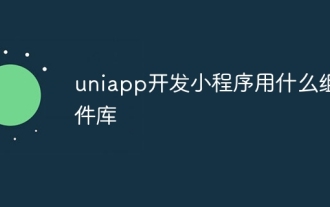
Recommended component library for uniapp to develop small programs: uni-ui: Officially produced by uni, it provides basic and business components. vant-weapp: Produced by Bytedance, with a simple and beautiful UI design. taro-ui: produced by JD.com and developed based on the Taro framework. fish-design: Produced by Baidu, using Material Design design style. naive-ui: Produced by Youzan, modern UI design, lightweight and easy to customize.
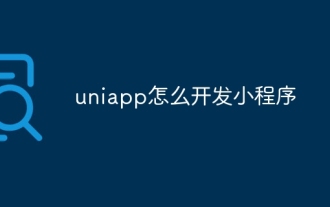
Cross-platform applets can be developed using a single set of code through the UniApp framework, including iOS, Android, and H5. The UniApp app development guide includes the following steps: Install the UniApp tool Create the project Select the coding language Add the app configuration Write the app code Compile the app Upload the app
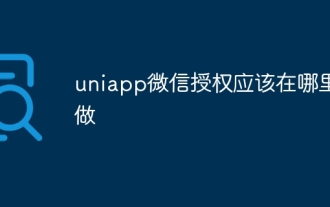
In uniapp development, WeChat authorization should be performed in the user interface component. The authorization process includes: obtaining the user code, exchanging the code for openId and unionId, and applying the openId or unionId for subsequent operations. The specific location depends on the business scenario. For example, authorization can be performed in the button click event handler that requires authorization.
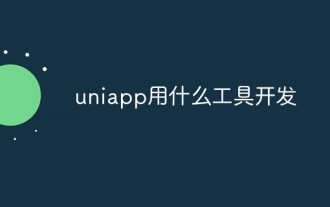
UniApp is a cross-platform mobile application development framework that allows the development of native applications for iOS, Android, HarmonyOS and the Web using a single code base. UniApp development tools provide tools to simplify the development process, including: HBuilderX: an IDE for code editing and debugging; CLI: a command line interface for executing UniApp commands; UniCloud: a back-end cloud service that provides data storage, etc. Function.
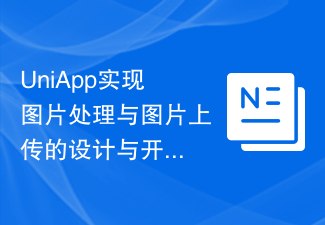
UniApp (UniversalApplication) is a cross-platform application development framework, an integrated solution developed based on Vue.js and Dcloud. It supports writing once and running on multiple platforms, enabling rapid development of high-quality mobile applications. In this article, we will introduce how to use UniApp to implement the design and development practice of image processing and image uploading. 1. Design and development of image processing In mobile application development, image processing is a common requirement. UniA
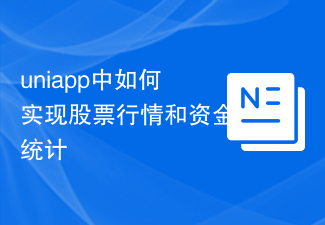
uniapp is a cross-platform application framework developed based on Vue.js, which can quickly and efficiently develop mobile applications. It is a very common requirement to implement stock quotes and fund statistics in uniapp. Specific code examples will be given below to help you realize this function. First, we need to obtain stock market data. In uniapp, you can obtain real-time stock market data by calling third-party APIs. The following is a code example to obtain stock quotes: //Import the network request of uni-app
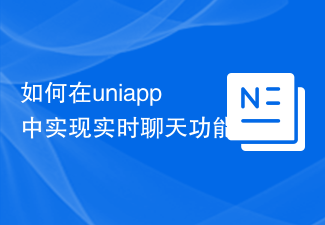
How to implement real-time chat function in uniapp Nowadays, with the continuous development of mobile Internet, real-time chat function has become one of the necessary functions of many applications. For developers, how to implement real-time chat functionality in uniapp has become an important topic. This article will introduce how to use WebSocket to implement real-time chat function in uniapp and provide code examples. 1. What is WebSocket? WebSocket is a full-double connection on a single TCP connection.
