


HTML, CSS, and jQuery: A technical guide to implementing image tile layouts
HTML, CSS and jQuery: Technical Guide to Implementing Image Tile Layout
Abstract: This article will introduce how to use HTML, CSS and jQuery to implement image tile layout . By using these techniques, you can create a beautiful and professional web layout to showcase your graphic artwork, product images, or any other images that require tiling. This article will provide you with a detailed technical guide and provide specific code examples to help you get started creating your own image tile layouts.
- HTML Layout
First, we need to create a basic HTML layout to accommodate our images. In this example, we will use a div container to contain all the images. You can change and adjust it to suit your needs.
<!DOCTYPE html> <html> <head> <style> .container { display: flex; flex-wrap: wrap; justify-content: center; } .container img { width: 200px; height: 200px; margin: 10px; } </style> </head> <body> <div class="container"> <!--在这里插入您的图片--> <img src="/static/imghw/default1.png" data-src="image1.jpg" class="lazy" alt="Image 1"> <img src="/static/imghw/default1.png" data-src="image2.jpg" class="lazy" alt="Image 2"> <img src="/static/imghw/default1.png" data-src="image3.jpg" class="lazy" alt="Image 3"> <img src="/static/imghw/default1.png" data-src="image4.jpg" class="lazy" alt="Image 4"> <img src="/static/imghw/default1.png" data-src="image5.jpg" class="lazy" alt="Image 5"> <img src="/static/imghw/default1.png" data-src="image6.jpg" class="lazy" alt="Image 6"> </div> </body> </html>
In the above code, we create a div container with the container
class and use the display: flex
property of CSS to make it a flex container. flex-wrap: The wrap
attribute will cause the image to automatically wrap in the container to adapt to changes in page width.
- CSS Style
In the above HTML code, we also define a CSS style that applies to all images. In this example, we set the width, height, and margins of the images so that they line up the way we want them to. You can customize it according to your needs.
.container img { width: 200px; height: 200px; margin: 10px; }
- jQuery Tile Layout
Now, we will introduce how to use jQuery to implement tile layout. First, you need to add a link to the jQuery library in your web page. You can download the latest version of the jQuery library from the official website (https://jquery.com/) and link it to your HTML file.
<!DOCTYPE html> <html> <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <style> .container { display: flex; flex-wrap: wrap; justify-content: center; } .container img { width: 200px; height: 200px; margin: 10px; } </style> <script> $(document).ready(function(){ // 获取容器和图片对象 var container = $(".container"); var images = container.find("img"); // 将图片按照平铺方式排列 function tileLayout() { var containerWidth = container.width(); var imageWidth = images.eq(0).outerWidth() + 20; // 加上外边距 var numPerRow = Math.floor(containerWidth / imageWidth); for (var i = 0; i < images.length; i += numPerRow) { images.slice(i, i + numPerRow).wrapAll('<div class="row"></div>'); } } // 页面加载完成后进行排列 tileLayout(); // 窗口大小改变时重新排列 $(window).resize(function(){ container.find(".row").unwrap(); tileLayout(); }); }); </script> </head> <body> <div class="container"> <!--在这里插入您的图片--> <img src="/static/imghw/default1.png" data-src="image1.jpg" class="lazy" alt="Image 1"> <img src="/static/imghw/default1.png" data-src="image2.jpg" class="lazy" alt="Image 2"> <img src="/static/imghw/default1.png" data-src="image3.jpg" class="lazy" alt="Image 3"> <img src="/static/imghw/default1.png" data-src="image4.jpg" class="lazy" alt="Image 4"> <img src="/static/imghw/default1.png" data-src="image5.jpg" class="lazy" alt="Image 5"> <img src="/static/imghw/default1.png" data-src="image6.jpg" class="lazy" alt="Image 6"> </div> </body> </html>
In the above code, we use jQuery's document ready event $(document).ready(function(){})
to ensure that the page is fully loaded before executing our code. First, we obtained the container and image objects, and defined a function named tileLayout()
, which is used to wrap the image in a tiled manner in a <div> element. <p>After the page is loaded, we first call the <code>tileLayout()
function to perform a layout. Then, we use the $(window).resize()
event to rearrange the images when the browser window size changes.
Summary:
By using HTML, CSS and jQuery, we can easily implement image tile layout. By using HTML's <div> container and CSS's <code>display: flex
property, we can create an automatically adapting layout. By using jQuery and some simple JavaScript code, we can dynamically adjust the layout of the image based on the page width. I hope this article will be helpful to you in implementing image tile layout!
The above is the detailed content of HTML, CSS, and jQuery: A technical guide to implementing image tile layouts. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
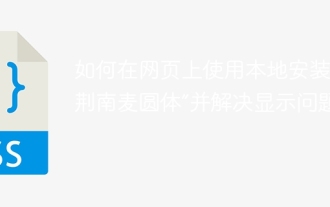
How to use locally installed font files on web pages In web development, users may want to use specific fonts installed on their computers to enhance the network...
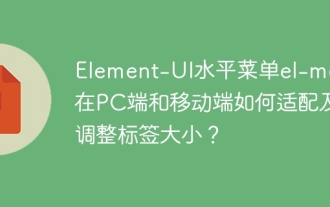
The adaptation issues of the Element-UI menu component el-menu and label size adjustment During the development process of using the Element-UI framework, the flexibility and ease of use of the el-menu component...
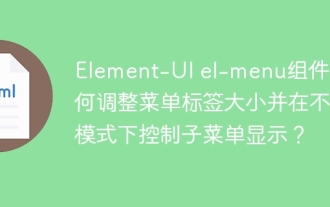
The label size adjustment of the Element-UI menu component el-menu and the behavior differences under the mode attributes of the Element-UI menu component will be used to determine the different mode modes of the el-menu component in the Element-UI framework...
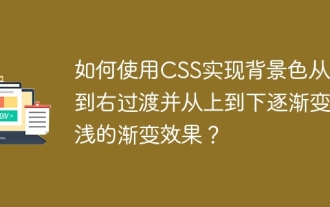
CSS gradient color effect implementation: Gradient background color from top to bottom In web design, how to transition from left to right in the search box and the background color under the carousel image...
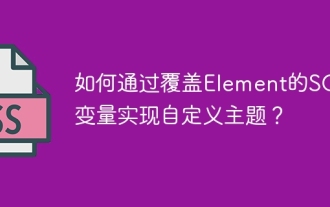
How to implement a custom theme by overriding the SCSS variable of Element? Using Element...
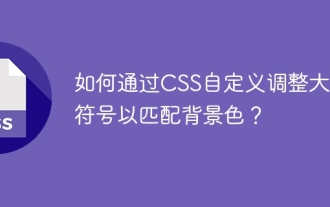
How to customize resize symbols with CSS to match background color? In web design, the details of the user experience can often significantly improve the overall effect. For example...
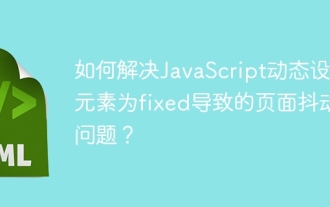
How to solve the problem of page jitter caused by dynamically setting elements to fixed by JS. When dynamically setting elements to fixed by JavaScript, you sometimes encounter page jitter...
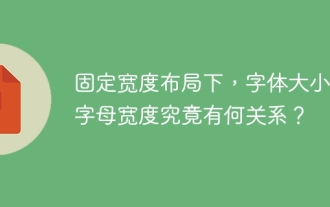
Under fixed width layout, the subtle relationship between font size and letter width When designing web pages, we often encounter the need to line up in fixed width containers...
