How to make a responsive fixed navigation using HTML, CSS and jQuery
How to use HTML, CSS and jQuery to make a responsive fixed navigation
In today's web design, responsive design has become a trend. Fixed navigation is a very common component in building a responsive website. Below we will introduce how to use HTML, CSS and jQuery to create a responsive fixed navigation and give specific code examples.
- HTML structure
First, we need to define the HTML structure of the navigation menu. A typical navigation menu contains a navigation bar and several navigation items. In HTML, we can use an unordered list (ul) to represent the navigation bar, and each navigation item is represented by a list item (li). Here is a simple example:
<nav> <ul> <li><a href="#">导航项1</a></li> <li><a href="#">导航项2</a></li> <li><a href="#">导航项3</a></li> <li><a href="#">导航项4</a></li> </ul> </nav>
- CSS Styles
Next, we need to add CSS styles to the navigation menu so that it stays anchored to the top of the page, and Adaptable layout to different screen sizes. Here is a basic CSS styling example:
nav { position: fixed; top: 0; left: 0; width: 100%; background-color: #333; } nav ul { list-style: none; margin: 0; padding: 0; display: flex; justify-content: center; } nav li { margin: 0 10px; } nav a { color: #fff; text-decoration: none; }
In this example, we use the CSS position: fixed
property to fix the navigation menu at the top of the page. The top: 0
and left: 0
properties position the navigation bar to the upper left corner of the page. width: 100%
Make the navigation bar horizontally cover the entire page. The background color is set to black using background-color: #333
. The styles of
nav ul
and nav li
define the layout of navigation items. We used the display: flex
property of CSS to center-align the navigation items horizontally. nav a
Defines colors and text decorations for links in navigation items.
- jQuery effect
Finally, we can use jQuery to add some interactive effects to the navigation menu, such as drop-down menus. The following is a simple jQuery code example:
$(document).ready(function() { // 隐藏下拉菜单 $('.dropdown-menu').hide(); // 鼠标悬停时显示下拉菜单 $('nav li').hover(function() { $(this).find('.dropdown-menu').slideDown(); }, function() { $(this).find('.dropdown-menu').slideUp(); }); });
In this example, jQuery's .hide()
and .show()
methods are used to control the drop-down Show and hide menus. Through the $('nav li').hover()
method, when the mouse hovers over the navigation item, the drop-down menu will expand downwards and collapse upward when the mouse moves out of the navigation item.
Summary
By studying the above code examples, I believe you have mastered how to use HTML, CSS and jQuery to create a responsive fixed navigation. Of course, the above is just a simple example. You can customize the style and interactive effects of the navigation menu more according to your actual needs.
The above is the detailed content of How to make a responsive fixed navigation using HTML, CSS and jQuery. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
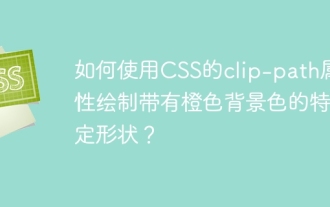
Practical application cases of CSS drawing function In modern web design, CSS can not only be used for layout and style, but also for creating complex graphics and animations. May...
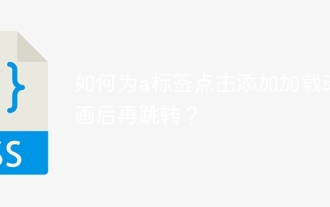
Cleverly implementing the short animation and jump after clicking the a tag, many times, we hope that after clicking the a tag, the page can first display a short loading event...
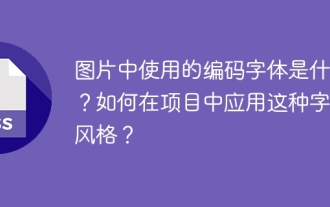
Introduction and use of encoded fonts In programming and web design, choosing the right font can greatly improve the readability and aesthetics of the code. recent,...
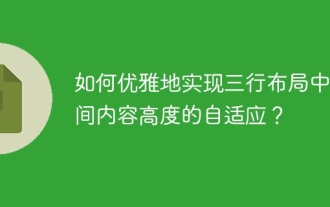
Discussion on the height of adaptive intermediate content in three-line layout In web layout, you often encounter the need to implement three-line layout and the intermediate content is highly variable...
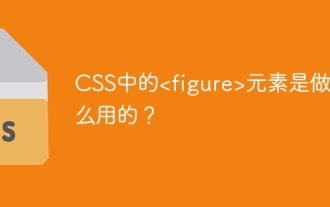
What are the elements in CSS for? During the learning and using CSS, you may encounter some less common HTML elements, such as <...
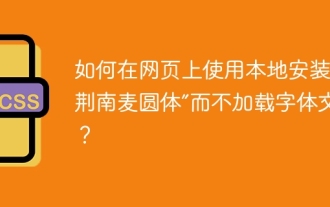
How to use locally installed font files on web pages In web page development, sometimes we will encounter the situation where we need to use specific fonts installed on our computer...
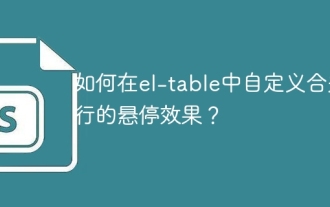
How to customize the hover effect of merge rows in el-table? Using Element...
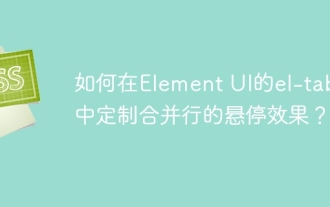
How to customize the el-table merge hover effect when using Element...
