How are iterators implemented in Python?
How are iterators implemented in Python?
Iterator is a very important concept in Python, which allows us to easily traverse and access elements in a collection. In Python, almost all iterable objects, such as lists, tuples, dictionaries, and sets, can be traversed through iterators. So how is iterator implemented? This article will introduce in detail how iterators are implemented in Python and provide specific code examples.
In Python, an iterator is an object that implements a method named __iter__() and __next__(). The __iter__() method returns the iterator object itself, while the __next__() method returns the next element in the iterator. When no elements can be returned, the __next__() method raises a StopIteration exception, indicating the end of the iteration. Let’s take a look at a specific code example:
class MyIterator: def __iter__(self): return self def __next__(self): # 实现迭代器的逻辑 pass
In the above example code, we have created an iterator class called MyIterator. This class implements the __iter__() and __next__() methods. The __iter__() method returns the iterator object itself, which allows us to iterate over the iterator. The __next__() method is used to return the next element in the iterator.
So, how do we implement the iterator logic in the __next__() method? Here is a simple example that shows how to use an iterator to traverse a list:
class MyIterator: def __init__(self, data): self.data = data self.index = 0 def __iter__(self): return self def __next__(self): if self.index >= len(self.data): raise StopIteration result = self.data[self.index] self.index += 1 return result # 创建一个迭代器对象 my_iterator = MyIterator([1, 2, 3, 4, 5]) # 遍历迭代器 for num in my_iterator: print(num)
In the above example, we created an iterator class called MyIterator and passed in a list as a parameter. In the __next__() method of the iterator, we use self.index to track the currently traversed index position, and access the elements in the list through self.data[self.index]. When all elements have been traversed, we use raise StopIteration to end the iteration.
Finally, we iterate over the iterator object by using a for-in loop. Each iteration, the __next__() method is called to return the next element in the iterator and print it out.
Summary: Iterators in Python are implemented by implementing the __iter__() and __next__() methods. The __iter__() method returns the iterator object itself, while the __next__() method returns the next element in the iterator. In an iterator, we can implement the logic of traversing and accessing elements by using indexes. I hope this article helps you understand how iterators are implemented in Python.
The above is the detailed content of How are iterators implemented in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


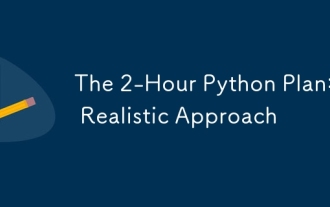
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
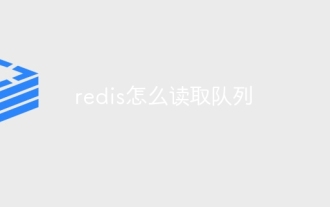
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
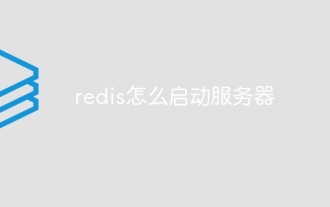
The steps to start a Redis server include: Install Redis according to the operating system. Start the Redis service via redis-server (Linux/macOS) or redis-server.exe (Windows). Use the redis-cli ping (Linux/macOS) or redis-cli.exe ping (Windows) command to check the service status. Use a Redis client, such as redis-cli, Python, or Node.js, to access the server.
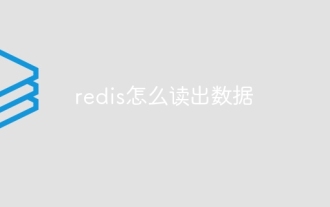
To read data from Redis, you can follow these steps: 1. Connect to the Redis server; 2. Use get(key) to get the value of the key; 3. If you need string values, decode the binary value; 4. Use exists(key) to check whether the key exists; 5. Use mget(keys) to get multiple values; 6. Use type(key) to get the data type; 7. Redis has other read commands, such as: getting all keys in a matching pattern, using cursors to iterate the keys, and sorting the key values.
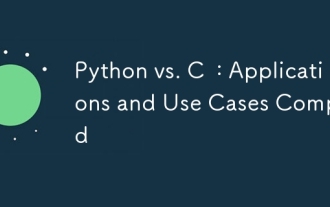
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
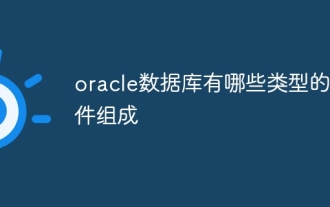
Oracle database file structure includes: data file: storing actual data. Control file: Record database structure information. Redo log files: record transaction operations to ensure data consistency. Parameter file: Contains database running parameters to optimize performance. Archive log file: Backup redo log file for disaster recovery.

There are several ways to find keys in Redis: Use the SCAN command to iterate over all keys by pattern or condition. Use GUI tools such as Redis Explorer to visualize the database and filter keys by name or schema. Write external scripts to query keys using the Redis client library. Subscribe to keyspace notifications to receive alerts when key changes.
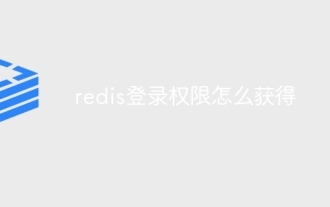
To obtain Redis login permission, you need to perform the following steps: 1. Create a username and password; 2. Allow remote connections; 3. Restart the Redis server; 4. Connect using the Redis CLI or programming language.
