


How to use memory management techniques and optimization algorithms in Python to improve code performance and resource utilization
How to use memory management techniques and optimization algorithms in Python to improve code performance and resource utilization
Introduction:
Python as a high-level programming language, with It is widely used for its concise, easy-to-read syntax and powerful functions. However, due to the nature of its dynamic typing and garbage collection mechanisms, Python may have some performance bottlenecks in memory management. In this article, I will introduce some memory management techniques and optimization algorithms in Python to help developers improve code performance and resource utilization.
1. Avoid frequent variable creation and deletion
In Python, the creation and deletion of variables will take up extra time and memory resources. Therefore, we should avoid frequent variable creation and deletion operations as much as possible. Consider using mutable objects to reduce the overhead of variable creation and deletion. For example, use lists to store data instead of creating new variables every time.
# 不推荐的写法 def sum_numbers(n): result = 0 for i in range(n): result += i return result # 推荐的写法 def sum_numbers(n): result = [] for i in range(n): result.append(i) return sum(result)
2. Using generators and iterators
Generators and iterators are very powerful memory management tools in Python. They are able to generate data on demand and access this data one by one without loading it all into memory at once.
# 不推荐的写法 def get_data(): records = db.query('SELECT * FROM big_table') return records # 推荐的写法 def get_data(): for record in db.query('SELECT * FROM big_table'): yield record
3. Use memory view(memory view)
Memory view is a tool in Python that efficiently accesses the underlying memory. It can operate the memory of an object as a sequence of bytes without copying. data. This reduces memory usage and improves code performance.
# 不推荐的写法 def change_array(arr): new_arr = [i * 2 for i in arr] return new_arr # 推荐的写法 def change_array(arr): mv = memoryview(arr) for i in range(len(arr)): mv[i] *= 2 return mv.tolist()
4. Use appropriate data structures and algorithms
Choosing appropriate data structures and algorithms is the key to improving code performance. There are many built-in data structures and algorithms in Python, such as dict, set, list, etc., which have different performance characteristics in different application scenarios. Depending on specific needs, choosing the right data structures and algorithms can greatly improve code performance and resource utilization.
# 不推荐的写法 def find_duplicate(nums): for i in range(len(nums)): for j in range(i+1, len(nums)): if nums[i] == nums[j]: return True return False # 推荐的写法 def find_duplicate(nums): nums_set = set() for num in nums: if num in nums_set: return True nums_set.add(num) return False
Conclusion:
We can improve the performance of Python code by avoiding frequent variable creation and deletion, using generators and iterators, using memory views, and choosing appropriate data structures and algorithms. Performance and resource utilization. Of course, these are just some common memory management techniques and optimization algorithms. There are many other methods and techniques in practical applications. I hope this article can provide you with some help in the Python programming process and make the code run more efficiently.
Reference:
- Python official documentation: https://docs.python.org/3/
- Python Cookbook, 3rd Edition, by David Beazley and Brian K. Jones
The above is the detailed content of How to use memory management techniques and optimization algorithms in Python to improve code performance and resource utilization. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

C++ object layout and memory alignment optimize memory usage efficiency: Object layout: data members are stored in the order of declaration, optimizing space utilization. Memory alignment: Data is aligned in memory to improve access speed. The alignas keyword specifies custom alignment, such as a 64-byte aligned CacheLine structure, to improve cache line access efficiency.
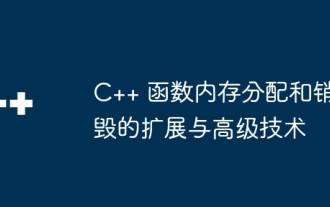
C++ function memory management provides extensions and advanced technologies, including: Custom allocator: allows users to define their own memory allocation strategies. placementnew and placementdelete: used when objects need to be allocated to specific memory locations. Advanced technologies: memory pools, smart pointers, and RAII to reduce memory leaks, improve performance, and simplify code.
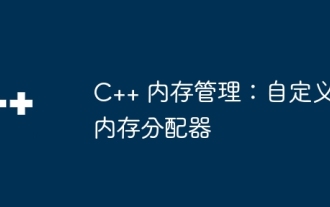
Custom memory allocators in C++ allow developers to adjust memory allocation behavior according to needs. Creating a custom allocator requires inheriting std::allocator and rewriting the allocate() and deallocate() functions. Practical examples include: improving performance, optimizing memory usage, and implementing specific behaviors. When using it, you need to pay attention to avoid freeing memory, manage memory alignment, and perform benchmark tests.
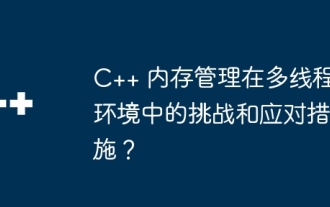
In a multi-threaded environment, C++ memory management faces the following challenges: data races, deadlocks, and memory leaks. Countermeasures include: 1. Use synchronization mechanisms, such as mutexes and atomic variables; 2. Use lock-free data structures; 3. Use smart pointers; 4. (Optional) implement garbage collection.

The reference counting mechanism is used in C++ memory management to track object references and automatically release unused memory. This technology maintains a reference counter for each object, and the counter increases and decreases when references are added or removed. When the counter drops to 0, the object is released without manual management. However, circular references can cause memory leaks, and maintaining reference counters increases overhead.
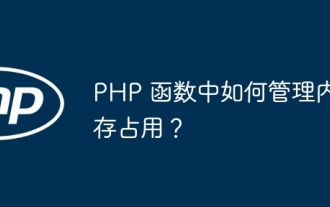
To manage memory usage in PHP functions: avoid declaring unnecessary variables; use lightweight data structures; release unused variables; optimize string processing; limit function parameters; optimize loops and conditions, such as avoiding infinite loops and using indexed arrays .
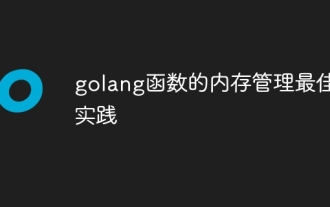
Memory management best practices in Go include: avoiding manual allocation/freeing of memory (using a garbage collector); using memory pools to improve performance when objects are frequently created/destroyed; using reference counting to track the number of references to shared data; using synchronized memory pools sync.Pool safely manages objects in concurrent scenarios.
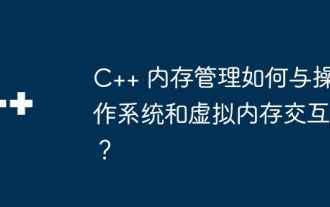
C++ memory management interacts with the operating system, manages physical memory and virtual memory through the operating system, and efficiently allocates and releases memory for programs. The operating system divides physical memory into pages and pulls in the pages requested by the application from virtual memory as needed. C++ uses the new and delete operators to allocate and release memory, requesting memory pages from the operating system and returning them respectively. When the operating system frees physical memory, it swaps less used memory pages into virtual memory.
