


How to implement a real-time monitoring system using Go language and Redis
How to use Go language and Redis to implement a real-time monitoring system
Introduction:
Real-time monitoring systems play an important role in today's software development. It can collect, analyze and display various system indicators in a timely manner, helping us understand the current operating status of the system and make timely adjustments and optimizations to the system. This article will introduce how to use Go language and Redis to implement a simple real-time monitoring system, and provide specific code examples.
1. What is a real-time monitoring system
A real-time monitoring system refers to a system that can collect and display various system indicators in real time, and can make timely adjustments and optimizations based on these indicators. It usually includes the following key components:
- Data collection: The monitoring system needs to be able to collect various system indicators, such as CPU usage, memory usage, network traffic, etc.
- Data storage: The collected indicators need to be stored for subsequent analysis and display. Commonly used data storage solutions include databases, file systems, caches, etc.
- Data analysis: The stored indicator data needs to be analyzed in order to generate charts, reports and other forms for display. Commonly used data analysis tools include Elasticsearch, Grafana, etc.
- Data display: Display the analyzed indicator data to users in the form of charts, reports, etc. to help users understand the operating status of the system.
- Alarm mechanism: When an abnormality occurs in the system, the administrator will be notified promptly via email, SMS, etc.
2. Implementation steps
- Install Go and Redis: First, we need to install the Go programming language and Redis database. Go can download the installation package from the official website, and Redis can be installed from the official website or package management tool.
- Introducing the Redis package: We can use Go's third-party Redis client package for development, such as "github.com/go-redis/redis".
-
Connection to Redis: In the program, we first need to establish a connection with the Redis database for subsequent data reading and writing.
The sample code is as follows:import ( "github.com/go-redis/redis" ) func main() { client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", DB: 0, }) _, err := client.Ping().Result() if err != nil { panic(err) } }
Copy after login Collect system indicators and store them in Redis: We can use the relevant libraries provided by Go to collect various system indicators and store them in Redis .
The sample code is as follows:import ( "github.com/shirou/gopsutil/cpu" "github.com/shirou/gopsutil/mem" "github.com/shirou/gopsutil/net" "time" ) func collectAndStoreMetrics(client *redis.Client) { for { cpuUsage, _ := cpu.Percent(time.Second, false) memUsage, _ := mem.VirtualMemory() netStats, _ := net.IOCounters(true) client.HSet("metrics", "cpuUsage", cpuUsage[0]) client.HSet("metrics", "memUsage", memUsage.UsedPercent) client.HSet("metrics", "netStats", netStats[0].BytesSent) time.Sleep(time.Second) } }
Copy after login- Analyze and display indicator data: We can use tools such as Grafana to analyze and display the data in Redis by querying it.
- Abnormal alarm mechanism: We can monitor changes in Redis data and when a certain indicator exceeds the specified threshold, notify the administrator in a timely manner via email, SMS, etc.
Conclusion:
Using Go language and Redis to implement a real-time monitoring system is an efficient and concise way. This article introduces the basic steps to implement a real-time monitoring system and provides corresponding code examples. I hope that through this example, readers can understand how to use Go and Redis to implement a real-time monitoring system, thereby providing some help for their own software development work.
The above is the detailed content of How to implement a real-time monitoring system using Go language and Redis. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
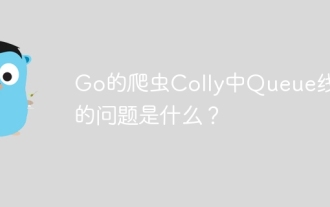
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
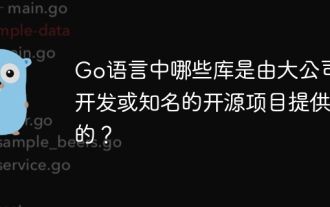
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
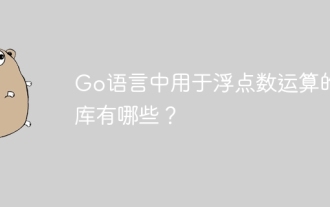
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
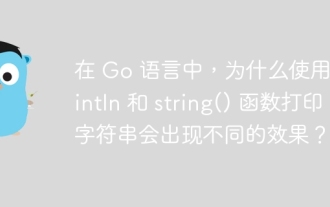
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
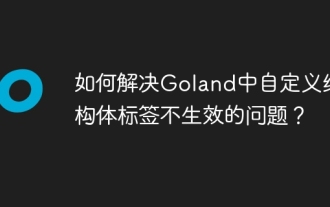
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
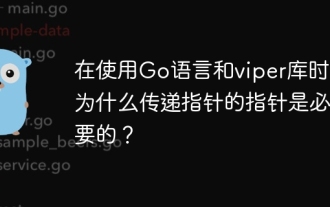
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
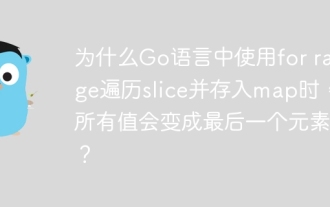
Why does map iteration in Go cause all values to become the last element? In Go language, when faced with some interview questions, you often encounter maps...
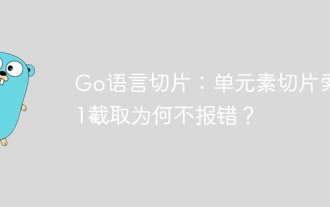
Go language slice index: Why does a single-element slice intercept from index 1 without an error? In Go language, slices are a flexible data structure that can refer to the bottom...
