HTML, CSS and jQuery: Make an animated accordion menu
HTML, CSS and jQuery: Making an animated collapsible menu
In web development, collapsible menus are a common interactive element that can save pages space, while also improving user experience. This article will introduce how to use HTML, CSS and jQuery to create an animated folding menu and provide specific code examples.
- HTML structure
First, we need to define an HTML structure to build a collapsible menu. The following is a simple HTML structure example:
<div class="menu-item"> <h3 id="菜单标题">菜单标题</h3> <div class="menu-content"> <!-- 菜单内容 --> </div> </div>
In the above code, .menu-item
is the outermost container, .menu-title
is the title of the menu, .menu-content
is the content of the menu, which is hidden in the initial state.
- CSS Style
Next, we need to add some CSS styles to the collapse menu to define the appearance and animation effects of the menu. The following is a basic CSS style example:
.menu-item { margin-bottom: 10px; } .menu-title { cursor: pointer; } .menu-content { display: none; } .menu-content.show { display: block; animation: fadeIn 0.3s ease-in-out; } @keyframes fadeIn { 0% { opacity: 0; } 100% { opacity: 1; } }
In the above code, we added some bottom spacing to .menu-item
to give a certain interval between menus. cursor: pointer
is set for .menu-title
to change the mouse style to indicate that the menu can be clicked to expand or collapse. The initial state of .menu-content
is hidden. When the .show
class name is added, the menu content will be displayed with a fade-in animation effect.
- jQuery animation effect
In order to realize the menu expansion and collapse function, we can use jQuery to add animation effects. The following is a basic jQuery code example:
$(document).ready(function() { $('.menu-title').click(function() { $(this).siblings('.menu-content').toggleClass('show'); }); });
In the above code, we use $(document).ready()
to ensure that the page is loaded before executing the code. When the .menu-title
element is clicked, use the toggleClass()
method to switch the .show
class name to achieve the expansion and collapse effect of the menu content.
- Complete sample code and effect preview
The following is a complete HTML file code example. You can copy and paste the code into an HTML file and view the effect in the browser:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>折叠菜单</title> <style> .menu-item { margin-bottom: 10px; } .menu-title { cursor: pointer; } .menu-content { display: none; } .menu-content.show { display: block; animation: fadeIn 0.3s ease-in-out; } @keyframes fadeIn { 0% { opacity: 0; } 100% { opacity: 1; } } </style> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <script> $(document).ready(function() { $('.menu-title').click(function() { $(this).siblings('.menu-content').toggleClass('show'); }); }); </script> </head> <body> <div class="menu-item"> <h3 id="菜单标题">菜单标题1</h3> <div class="menu-content"> <p>菜单内容1</p> </div> </div> <div class="menu-item"> <h3 id="菜单标题">菜单标题2</h3> <div class="menu-content"> <p>菜单内容2</p> </div> </div> <div class="menu-item"> <h3 id="菜单标题">菜单标题3</h3> <div class="menu-content"> <p>菜单内容3</p> </div> </div> </body> </html>
You can run the above code in the browser, click on the menu title, and you will see the menu content expand and collapse with a fade-in animation effect.
To sum up, by using HTML, CSS and jQuery, we can easily create a folding menu with animated effects. I hope the sample code in this article can be helpful to you, go and try it!
The above is the detailed content of HTML, CSS and jQuery: Make an animated accordion menu. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


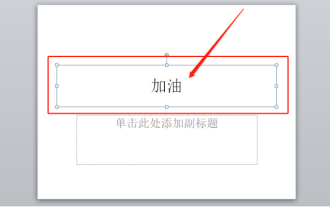
We often use ppt in our daily work, so are you familiar with every operating function in ppt? For example: How to set animation effects in ppt, how to set switching effects, and what is the effect duration of each animation? Can each slide play automatically, enter and then exit the ppt animation, etc. In this issue, I will first share with you the specific steps of entering and then exiting the ppt animation. It is below. Friends, come and take a look. Look! 1. First, we open ppt on the computer, click outside the text box to select the text box (as shown in the red circle in the figure below). 2. Then, click [Animation] in the menu bar and select the [Erase] effect (as shown in the red circle in the figure). 3. Next, click [
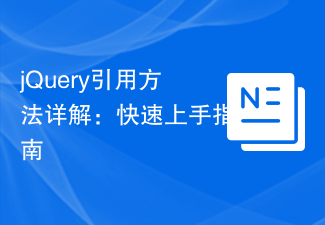
Detailed explanation of jQuery reference method: Quick start guide jQuery is a popular JavaScript library that is widely used in website development. It simplifies JavaScript programming and provides developers with rich functions and features. This article will introduce jQuery's reference method in detail and provide specific code examples to help readers get started quickly. Introducing jQuery First, we need to introduce the jQuery library into the HTML file. It can be introduced through a CDN link or downloaded
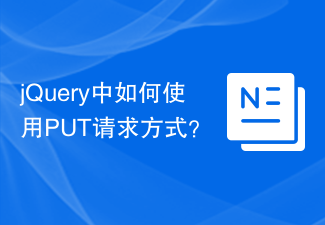
How to use PUT request method in jQuery? In jQuery, the method of sending a PUT request is similar to sending other types of requests, but you need to pay attention to some details and parameter settings. PUT requests are typically used to update resources, such as updating data in a database or updating files on the server. The following is a specific code example using the PUT request method in jQuery. First, make sure you include the jQuery library file, then you can send a PUT request via: $.ajax({u
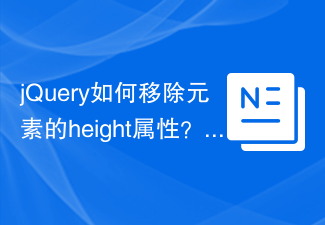
How to remove the height attribute of an element with jQuery? In front-end development, we often encounter the need to manipulate the height attributes of elements. Sometimes, we may need to dynamically change the height of an element, and sometimes we need to remove the height attribute of an element. This article will introduce how to use jQuery to remove the height attribute of an element and provide specific code examples. Before using jQuery to operate the height attribute, we first need to understand the height attribute in CSS. The height attribute is used to set the height of an element
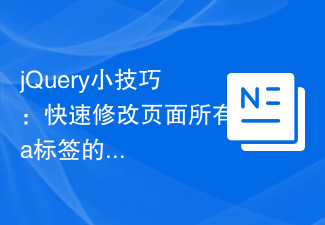
Title: jQuery Tips: Quickly modify the text of all a tags on the page In web development, we often need to modify and operate elements on the page. When using jQuery, sometimes you need to modify the text content of all a tags in the page at once, which can save time and energy. The following will introduce how to use jQuery to quickly modify the text of all a tags on the page, and give specific code examples. First, we need to introduce the jQuery library file and ensure that the following code is introduced into the page: <
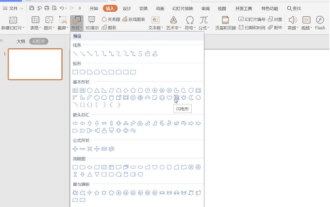
Sometimes we encounter the need to add animation to a ppt. For example, if we want to make a thunderstorm ppt and add some animated thunderstorm effects to it, what should we do? Today, the editor will introduce to you how to make an animated thunderstorm in thunderstorm ppt. It is actually very simple, come and learn it! 1. First we open a PPT page, "Insert" - "Shape" - "Basic Shape" - "Lightning Shape", as shown in the picture. 2. In the "Fill and Line" tab on the right, select "Fill": white; "Select" "Line": black, as shown in the figure. 3. Click "Animation" - "Custom Animation" - "Add Effects" - "Emphasis" - "Subtle" - "Flickering",
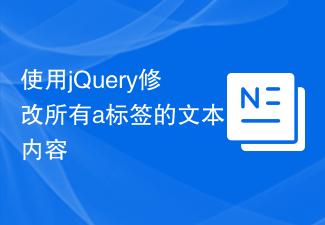
Title: Use jQuery to modify the text content of all a tags. jQuery is a popular JavaScript library that is widely used to handle DOM operations. In web development, we often encounter the need to modify the text content of the link tag (a tag) on the page. This article will explain how to use jQuery to achieve this goal, and provide specific code examples. First, we need to introduce the jQuery library into the page. Add the following code in the HTML file:
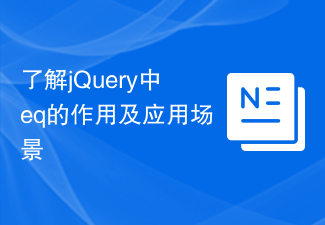
jQuery is a popular JavaScript library that is widely used to handle DOM manipulation and event handling in web pages. In jQuery, the eq() method is used to select elements at a specified index position. The specific usage and application scenarios are as follows. In jQuery, the eq() method selects the element at a specified index position. Index positions start counting from 0, i.e. the index of the first element is 0, the index of the second element is 1, and so on. The syntax of the eq() method is as follows: $("s
