


Generators in PHP7: How to handle large amounts of data and lazy loading efficiently?
The concept of generator (Generator) was introduced in PHP7, which provides a method to efficiently handle large amounts of data and lazy loading. This article will start with concepts and principles, combined with specific code examples, to introduce the usage and advantages of generators in PHP7.
A generator is a special function that does not return all data at once, but generates data on demand. When the function executes the yield statement, the currently generated value will be returned, and the function's state will be saved. The next time the generator function is called, the function continues execution from the previous state until it encounters a yield statement again, and then returns a value again.
The benefit of a generator is that it can reduce memory usage, especially when processing large amounts of data. The traditional way is to save all the data in an array and then return it to the caller at once. But for a large amount of data, this approach will occupy a lot of memory space. The generator only returns one value at a time and does not occupy too much memory at one time, thus improving the performance and efficiency of the program.
The following uses a practical case to specifically illustrate the usage of the generator. Suppose we have a very large file with each line containing a number, and we want to read the file and return the square of all the numbers. The traditional way is to save all the numbers into an array and then square each number in the array. But this method will occupy a lot of memory. We can use generators to solve this problem.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
|
The above code defines a generator function squareNumbers
that accepts a file name as a parameter and then uses the fopen
function to open the file and read the file line by line content. Each time a row is read, the number in the row is squared and returned using the yield
statement. Through the yield
statement, we can return each generated value to the caller one by one.
In the main program, we can use the generator like iterating an array and print out the values returned by the generator function one by one through the foreach
loop. What needs to be noted here is that the generator function will re-execute the code in the generator function body every time it is called, rather than executing the entire function. This avoids loading large amounts of data into memory at once.
By using generators, we can efficiently process large amounts of data. Whether it is reading data from files, obtaining data from databases, or other types of data operations, generators can help us reduce memory usage. , improve program performance.
In addition to handling large amounts of data, generators can also be used for lazy loading. Lazy loading refers to generating data only when needed, rather than generating it all at once. This can be useful in certain situations, especially when working with large collections or operations that need to run for a long time. By using generators, we can generate data only when needed, thereby reducing unnecessary computation and resource consumption.
In short, generators in PHP7 provide a way to efficiently handle large amounts of data and lazy loading. By generating values one by one instead of all at once, we can reduce memory usage and improve program performance and efficiency. In actual development, we can use generators flexibly to improve code readability and maintainability.
The above is the detailed content of Generators in PHP7: How to handle large amounts of data and lazy loading efficiently?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


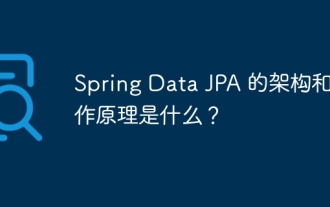
SpringDataJPA is based on the JPA architecture and interacts with the database through mapping, ORM and transaction management. Its repository provides CRUD operations, and derived queries simplify database access. Additionally, it uses lazy loading to only retrieve data when necessary, thus improving performance.
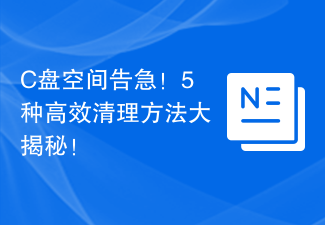
C drive space is running out! 5 efficient cleaning methods revealed! In the process of using computers, many users will encounter a situation where the C drive space is running out. Especially after storing or installing a large number of files, the available space of the C drive will decrease rapidly, which will affect the performance and running speed of the computer. At this time, it is very necessary to clean up the C drive. So, how to clean up C drive efficiently? Next, this article will reveal 5 efficient cleaning methods to help you easily solve the problem of C drive space shortage. 1. Clean up temporary files. Temporary files are temporary files generated when the computer is running.
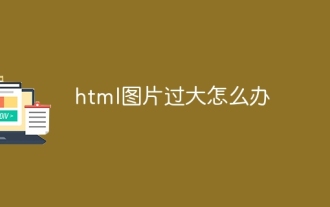
Here are some ways to optimize HTML images that are too large: Optimize image file size: Use a compression tool or image editing software. Use media queries: Dynamically resize images based on device. Implement lazy loading: only load the image when it enters the visible area. Use a CDN: Distribute images to multiple servers. Use image placeholder: Display a placeholder image while the image is loading. Use thumbnails: Displays a smaller version of the image and loads the full-size image on click.

Decoding Laravel performance bottlenecks: Optimization techniques fully revealed! Laravel, as a popular PHP framework, provides developers with rich functions and a convenient development experience. However, as the size of the project increases and the number of visits increases, we may face the challenge of performance bottlenecks. This article will delve into Laravel performance optimization techniques to help developers discover and solve potential performance problems. 1. Database query optimization using Eloquent delayed loading When using Eloquent to query the database, avoid
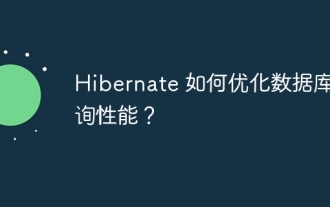
Tips for optimizing Hibernate query performance include: using lazy loading to defer loading of collections and associated objects; using batch processing to combine update, delete, or insert operations; using second-level cache to store frequently queried objects in memory; using HQL outer connections , retrieve entities and their related entities; optimize query parameters to avoid SELECTN+1 query mode; use cursors to retrieve massive data in blocks; use indexes to improve the performance of specific queries.
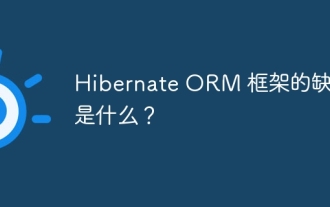
The HibernateORM framework has the following shortcomings: 1. Large memory consumption because it caches query results and entity objects; 2. High complexity, requiring in-depth understanding of the architecture and configuration; 3. Delayed loading delays, leading to unexpected delays; 4. Performance bottlenecks, in May occur when a large number of entities are loaded or updated at the same time; 5. Vendor-specific implementation, resulting in differences between databases.

Python and C++ are two popular programming languages, each with its own advantages and disadvantages. For people who want to learn programming, choosing to learn Python or C++ is often an important decision. This article will explore the learning costs of Python and C++ and discuss which language is more worthy of the time and effort. First, let's start with Python. Python is a high-level, interpreted programming language known for its ease of learning, clear code, and concise syntax. Compared to C++, Python
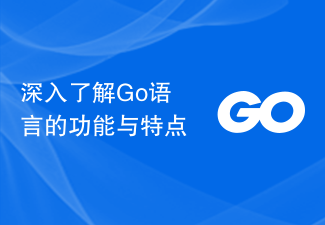
Functions and features of Go language Go language, also known as Golang, is an open source programming language developed by Google. It was originally designed to improve programming efficiency and maintainability. Since its birth, Go language has shown its unique charm in the field of programming and has received widespread attention and recognition. This article will delve into the functions and features of the Go language and demonstrate its power through specific code examples. Native concurrency support The Go language inherently supports concurrent programming, which is implemented through the goroutine and channel mechanisms.
