


How to use Go language to develop the business time management function of the ordering system
How to use Go language to develop the business hours management function of the ordering system
Introduction:
Developing an ordering system, in addition to basic ordering and checkout In addition to functions, business hours management in different time periods also needs to be considered. In some restaurants, breakfast, lunch, and dinner have different menus and prices, so the system needs to be able to accurately control the menu display and price calculation at different time periods. This article will introduce how to use Go language to develop the business hours management function of the ordering system, and provide specific code examples for reference.
1. Demand analysis
When developing the business hours management function of the ordering system, we need to consider the following demand points:
- Be able to customize different time periods Business hours rules;
- In different time periods, display and price calculation should be limited to menus in that time period;
- When users select menus, they need to be reminded of information that is not currently in the business hours. ;
- The system should be able to automatically determine whether the current time is within business hours, and make corresponding displays and calculations based on the situation.
2. Design Plan
Based on the above demand analysis, we can design the following business hours management function implementation plan:
- Use Go language to write a time period management The structure of the controller is used to define business rules for different time periods;
- A function is needed to determine whether the current time is within a certain time period;
- Define a menu structure, including dishes Name, price and other related information;
- Define a global menu list variable to store all menu information;
- When displaying the order page, determine which menus to display based on the current time. And calculate the price;
- Provides a function for setting menus and price rules for different time periods.
3. Code Implementation
Next, we will give specific code implementation examples, please refer to the following sample code:
package main import ( "fmt" "time" ) type TimeRange struct { StartTime time.Time EndTime time.Time } type MenuItem struct { Name string Price float64 TimeRule TimeRange } var menuList []MenuItem func isTimeInRange(t time.Time, tr TimeRange) bool { return t.After(tr.StartTime) && t.Before(tr.EndTime) } func showMenuByTime() { currentTime := time.Now() for _, item := range menuList { if isTimeInRange(currentTime, item.TimeRule) { fmt.Println(item.Name, item.Price) } } } func main() { // 初始化菜单列表 menuList = []MenuItem{ {Name: "早餐A", Price: 20.5, TimeRule: TimeRange{ StartTime: time.Date(2022, time.January, 1, 8, 0, 0, 0, time.UTC), EndTime: time.Date(2022, time.January, 1, 10, 0, 0, 0, time.UTC), }}, {Name: "午餐A", Price: 30.0, TimeRule: TimeRange{ StartTime: time.Date(2022, time.January, 1, 11, 0, 0, 0, time.UTC), EndTime: time.Date(2022, time.January, 1, 14, 0, 0, 0, time.UTC), }}, {Name: "晚餐A", Price: 40.0, TimeRule: TimeRange{ StartTime: time.Date(2022, time.January, 1, 18, 0, 0, 0, time.UTC), EndTime: time.Date(2022, time.January, 1, 22, 0, 0, 0, time.UTC), }}, } showMenuByTime() }
In the above code examples, we use TimeRange
Structure to define business rules for different time periods. MenuItem
The structure contains the name, price, time rules and other information of the dish. menuList
is a global variable used to store all menu information.
isTimeInRange
The function is used to determine whether the current time is within the specified time range. showMenuByTime
The function displays the corresponding menu based on the current time.
In the main
function, we initialize the menu list and call the showMenuByTime
function to display the menu in the current time period.
4. Summary
Through the above implementation code, we can see that using Go language to develop the business time management function of the ordering system is not complicated. By defining the time period manager and menu structure, and combining the time judgment function and menu display logic, we can realize the business hours management capabilities of the ordering system in different time periods.
In actual development, we can further expand according to actual needs, such as adding and setting price rules for different time periods, providing an operation interface to facilitate management, and other functions. I hope that the solutions and code examples provided in this article can be helpful to students who develop ordering systems.
The above is the detailed content of How to use Go language to develop the business time management function of the ordering system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
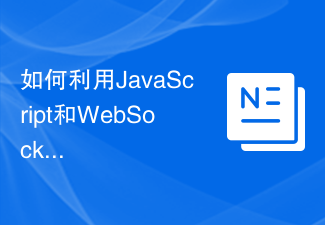
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
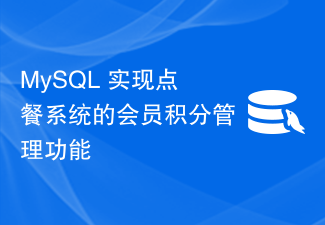
MySQL implements the member points management function of the ordering system 1. Background introduction With the rapid development of the catering industry, many restaurants have begun to introduce ordering systems to improve efficiency and customer satisfaction. In the ordering system, the member points management function is a very important part, which can attract customers to spend and increase the return rate through the accumulation and redemption of points. This article will introduce how to use MySQL to implement the member points management function of the ordering system and provide specific code examples. 2. Database design In MySQL, relational data can be used
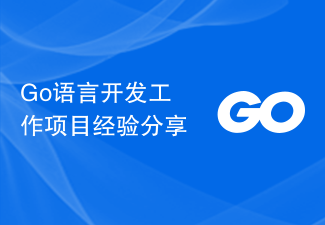
With the development of the Internet, the field of computer science has also ushered in many new programming languages. Among them, Go language has gradually become the first choice of many developers due to its concurrency and concise syntax. As an engineer engaged in software development, I was fortunate to participate in a work project based on the Go language, and accumulated some valuable experience and lessons in the process. First, choosing the right frameworks and libraries is crucial. Before starting the project, we conducted detailed research, tried different frameworks and libraries, and finally chose the Gin framework as our
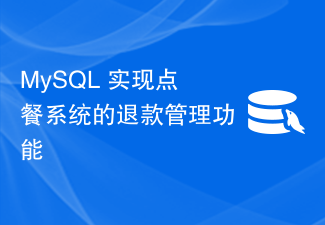
MySQL implements the refund management function of the food ordering system. With the rapid development of Internet technology, the food ordering system has gradually become a standard feature in the catering industry. In the ordering system, the refund management function is a very critical link, which has an important impact on the consumer experience and the efficiency of restaurant operations. This article will introduce in detail how to use MySQL to implement the refund management function of the ordering system and provide specific code examples. 1. Database design Before implementing the refund management function, we need to design the database. Mainly involves three tables: Order
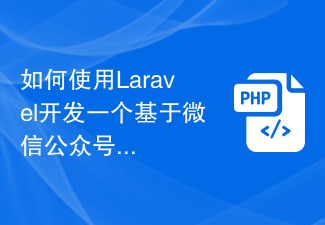
How to use Laravel to develop an online ordering system based on WeChat official accounts. With the widespread use of WeChat official accounts, more and more companies are beginning to use them as an important channel for online marketing. In the catering industry, developing an online ordering system based on WeChat public accounts can improve the efficiency and sales of enterprises. This article will introduce how to use the Laravel framework to develop such a system and provide specific code examples. Project preparation First, you need to ensure that the Laravel framework has been installed in the local environment. OK
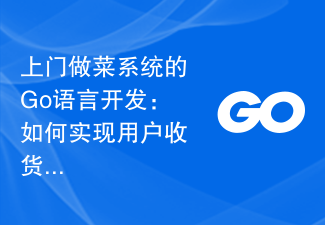
As people's quality of life improves, more and more families are choosing to enjoy high-quality catering services at home. The door-to-door cooking system emerged as the times require and has become a convenient, safe and healthy way to choose food. Under such a service, users can place an order online, and professional chefs will come to prepare the ingredients, cook the food, and deliver it to the user's home for enjoyment. The Go language has the characteristics of high efficiency, stability, and security, so it can achieve very good results when developed with a door-to-door cooking system. This article will introduce how to implement the user's delivery address in the door-to-door cooking system
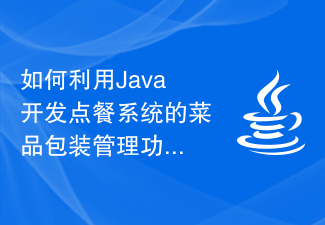
How to use Java to develop the dish packaging management function of the ordering system. With the development of society and the improvement of people's living standards, more and more people choose to eat out. The catering industry has also developed rapidly as a result, and various restaurants continue to emerge. In order to improve the competitiveness and service quality of restaurants, many restaurants have begun to introduce ordering systems to facilitate customers to order, pay, and manage menus. Dishes packaging management is one of the important links in the ordering system. This article will introduce how to use Java to develop the dish packaging management function of the ordering system. 1. Requirements analysis
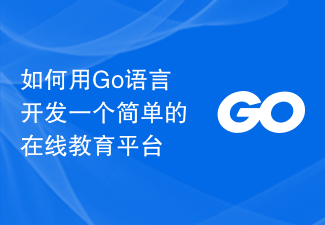
How to develop a simple online education platform using Go language Introduction: Today, the development of the Internet has penetrated into all walks of life, and the field of education is no exception. The emergence of online education platforms has made learning more flexible and convenient, and has been favored by students and parents. This article will introduce how to use Go language to develop a simple online education platform, including platform construction, function development and database design. 1. Platform construction First, we need to install the Go language development environment. You can download and install the latest version from the official website
