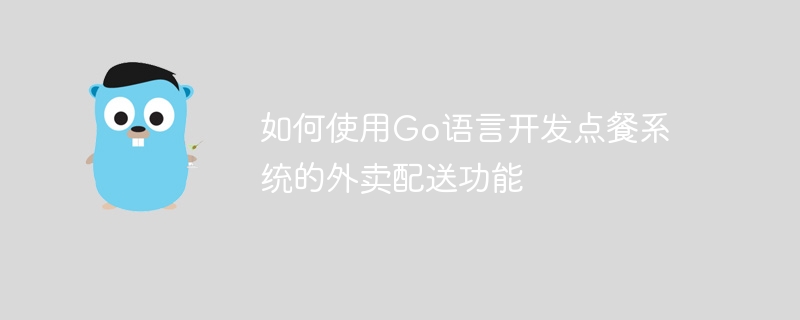
How to use Go language to develop the takeout delivery function of the ordering system
With the rapid development of the takeout industry, more and more restaurants and users are beginning to use ordering system and takeout delivery services. This article will introduce how to use Go language to develop a takeout delivery function based on the ordering system, including order management, rider delivery, order status tracking, etc.
- System Overview
The takeout delivery function of the ordering system is a complex system, which needs to involve multiple roles and modules such as users, restaurants, riders, and orders. Among them, after the user submits the order, the restaurant receives the order and prepares the food, and then assigns the order to the rider for delivery. After the rider receives the order, he will deliver the order according to the address of the order and update the order status to the system.
- Database Design
Before starting development, we need to design the database. The following are some commonly used table design examples:
- User table: Contains the user's basic information, such as user name, password, mobile phone number, etc.
- Restaurant table: Contains restaurant-related information, such as name, address, contact information, etc.
- Meal table: Contains meal information, such as name, price, restaurant ID, etc.
- Order table: Contains order information, such as user ID, restaurant ID, meal ID, order status, etc.
- Rider table: Contains rider information, such as name, mobile phone number, work status, etc.
- User submits order
Users select meals in the ordering system and submit orders. In the backend, we need to process the user's request to submit an order, save the order information to the order table of the database, and set the order status to pending order.
Sample code:
func submitOrder(userId int, restaurantId int, items []int) error {
// 将订单信息保存到数据库中,并设置状态为待接单
orderId, err := saveOrder(userId, restaurantId, items, OrderStatusPending)
if err != nil {
return err
}
// 向餐馆发送通知,告知有新订单
err = notifyRestaurant(restaurantId, orderId)
if err != nil {
return err
}
return nil
}
Copy after login
- Restaurant receiving orders
The restaurant will periodically poll the system to obtain new orders and process them. When the restaurant receives a new order, it needs to update the status of the order to pending delivery and prepare the food.
Sample code:
func handleNewOrder(orderId int) error {
// 将订单状态更新为待配送
err := updateOrderStatus(orderId, OrderStatusReady)
if err != nil {
return err
}
// 准备食物
err = prepareFood(orderId)
if err != nil {
return err
}
return nil
}
Copy after login
- Rider receiving orders
The rider will periodically poll the system to obtain the orders assigned to itself. After the rider receives the order, he needs to update the order status to being delivered and start delivery.
Sample code:
func acceptOrder(orderId int, riderId int) error {
// 将订单状态更新为配送中,并指定骑手ID
err := updateOrderStatusAndRider(orderId, OrderStatusDelivering, riderId)
if err != nil {
return err
}
// 开始配送
err = startDelivery(orderId)
if err != nil {
return err
}
return nil
}
Copy after login
- Order status tracking
Users can check the status of the order through the ordering system to understand the progress of the order. In the backend, we need to provide an interface for obtaining order status.
Sample code:
func getOrderStatus(orderId int) (string, error) {
// 查询订单状态
status, err := queryOrderStatus(orderId)
if err != nil {
return "", err
}
return status, nil
}
Copy after login
Summary
This article introduces how to use Go language to develop the takeout delivery function of a food ordering system. Through order management, rider delivery and order status tracking, a complete takeout delivery system is implemented. Of course, this is just a simple example, and actual systems need to consider more scenarios and functions. I hope this article can be helpful to you. If you have any questions, please feel free to ask.
The above is the detailed content of How to use Go language to develop the takeout delivery function of the ordering system. For more information, please follow other related articles on the PHP Chinese website!