


How to implement the shopping cart function of developing a grocery shopping system with PHP
With the continuous development of online shopping, the shopping cart has become an indispensable function, and the grocery shopping system is no exception. The shopping cart is also extremely important and practical. This article will introduce how to use PHP to develop the shopping cart function of the grocery shopping system.
1. Storage method of shopping cart data
The essence of a shopping cart is a container in which product information needs to be stored. Common storage methods are as follows:
- MySQL database:
Store the shopping cart information in the database. MySQL can be used to handle the storage, processing and management of shopping cart related information. For example, create a cart table and store related information such as user ID, product ID and quantity.
- Session session:
Shopping cart information can also be stored using Session session to store the product information that users add to the shopping cart in Session, avoiding frequent database operations. , reducing the pressure on the server side.
2. Steps to implement the shopping cart function
- Add items to the shopping cart:
When the user is browsing the dishes, click the add to shopping cart button . At this time, the product information needs to be added to the shopping cart. For the MySQL database storage method, you can create an add_to_cart.php page and connect to the MySQL database through PHP to add the product information to the shopping cart table of the database.
If you use Session storage, you can use the following code to achieve it:
<?php session_start(); // 将商品加入购物车 $_SESSION['cart'][$product_id]['name'] = $product_name; $_SESSION['cart'][$product_id]['price'] = $product_price; $_SESSION['cart'][$product_id]['quantity'] = $product_quantity; ?>
- Update the number of items in the shopping cart:
The user is in the shopping cart Modify the quantity of the product. At this time, you need to update the quantity of the product in the shopping cart. For the MySQL storage method, you can create an update_cart.php page, obtain the user's modified product quantity through POST, and then update it to the MySQL database.
If you use the Session storage method, you can use the following code to achieve it:
<?php session_start(); // 更新购物车中商品的数量 $_SESSION['cart'][$product_id]['quantity'] = $new_quantity; ?>
- Delete items in the shopping cart:
The user is in the shopping cart Delete the product. At this time, the product needs to be removed from the shopping cart. For the MySQL storage method, you can create a delete_from_cart.php page, obtain the product ID that needs to be deleted through GET, and then delete it from the MySQL database.
If you use Session storage, you can use the following code to achieve it:
<?php session_start(); // 从购物车中删除商品 unset($_SESSION['cart'][$product_id]); ?>
- Display the shopping cart product list and total price:
The user is browsing When shopping, you need to see the product list and total price in the shopping cart. For the MySQL storage method, you can create a view_cart.php page, connect to the MySQL database, read the product information and quantity information in the shopping cart table, then display it on the page, and calculate the total price of the products in the shopping cart.
If you use the Session storage method, you can use the following code to achieve it:
<?php session_start(); $total_price = 0; // 遍历购物车中的商品信息 foreach($_SESSION['cart'] as $product_id => $product) { $product_name = $product['name']; $product_price = $product['price']; $product_quantity = $product['quantity']; // 计算总价 $total_price += $product_price * $product_quantity; // 在页面上显示出商品信息和数量 echo "<p>{$product_name}数量:{$product_quantity}</p>"; } // 输出商品总价 echo "<p>商品总价:{$total_price}元</p>"; ?>
3. Implementation effect of the shopping cart function
After the shopping cart function is implemented, the user will browse When selecting dishes, you can add items to the shopping cart by clicking the Add to Cart button. The shopping cart will display the product information and total price in the shopping cart in real time. Users can modify the quantity of items on the shopping cart page, or delete an item from the shopping cart.
The above is how to implement the shopping cart function of developing a grocery shopping system using PHP. You can choose the storage method and implementation steps that suit you according to the specific situation.
The above is the detailed content of How to implement the shopping cart function of developing a grocery shopping system with PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


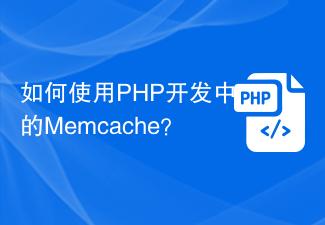
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
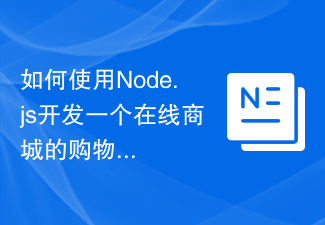
How to use Node.js to develop the shopping cart function of an online mall. In today's Internet era, e-commerce has become one of the main ways for people to shop. A complete shopping cart function is very important for online shopping malls. It can provide users with a convenient shopping experience and improve user conversion rates. This article will introduce how to use Node.js to develop a shopping cart function for an online mall and provide specific code examples. Environment preparation First, make sure that Node.js and npm are installed on your computer. you can
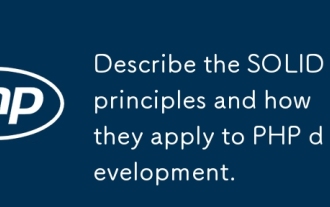
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.

How to implement permission-based multi-language support in Laravel Introduction: In modern websites and applications, multi-language support is a very common requirement. For some complex systems, we may also need to dynamically display different language translations based on the user's permissions. Laravel is a very popular PHP framework that provides many powerful features to simplify the development process. This article will introduce how to implement permission-based multi-language support in Laravel and provide specific code examples. Step 1: Configure multi-language support first
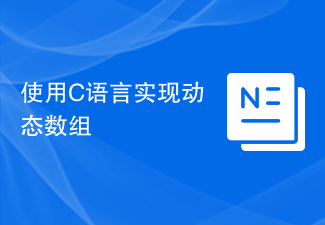
Dynamic array C language implementation method Dynamic array refers to a data structure that can dynamically allocate and release memory as needed during program running. Compared with static arrays, the length of dynamic arrays can be dynamically adjusted at runtime, thus more flexibly meeting the needs of the program. In C language, the implementation of dynamic arrays relies on the dynamic memory allocation functions malloc and free. The malloc function is used to apply for a memory space of a specified size, while the free function is used to release the previously applied memory space. Below is an example
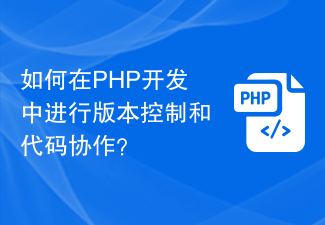
How to implement version control and code collaboration in PHP development? With the rapid development of the Internet and the software industry, version control and code collaboration in software development have become increasingly important. Whether you are an independent developer or a team developing, you need an effective version control system to manage code changes and collaborate. In PHP development, there are several commonly used version control systems to choose from, such as Git and SVN. This article will introduce how to use these tools for version control and code collaboration in PHP development. The first step is to choose the one that suits you
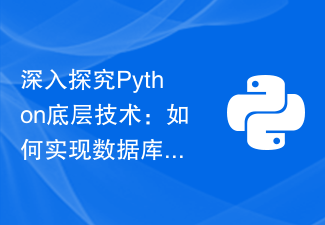
In-depth exploration of Python's underlying technology: how to implement database connection pooling Introduction: In modern application development, the database is an indispensable part. For database connections and management, connection pooling is a very important technology. This article will delve into how to implement a simple database connection pool in Python and provide specific code examples. 1. What is a database connection pool? A database connection pool is a technology for managing database connections. It maintains a certain number of database connections and effectively manages and manages the connections.
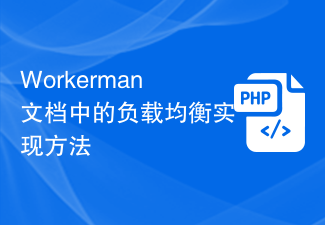
Workerman is a high-performance network framework developed based on PHP and is widely used to build real-time communication systems and high-concurrency services. In actual application scenarios, we often need to improve system reliability and performance through load balancing. This article will introduce how to implement load balancing in Workerman and provide specific code examples. Load balancing refers to allocating network traffic to multiple back-end servers to improve the system's load capacity, reduce response time, and increase system availability and scalability. In Wo
