


How to use PHP to develop the reservation ordering function of the food ordering system?
With the development of the catering industry, more and more restaurants have begun to provide reservation ordering services, which not only provides customers with a more convenient dining experience, but also provides restaurants with A more orderly and efficient management method. This article will introduce how to use PHP to develop the reservation ordering function of the food ordering system.
1. The basic structure of the reservation and ordering function
The basic structure of the reservation and ordering function includes two main parts: the reservation system and the ordering system. The reservation system is mainly responsible for managing customers' reservation information, including table reservations, customer information management, etc.; while the ordering system is mainly responsible for customers' ordering operations, dish management, etc.
2. Implementation of reservation system
- Database design
Create database tables: reservation, customer, table
① reservation table
id: self-increasing primary key
customer_id: foreign key, associated with the id field of the customer table
table_id: foreign key, associated with the id field of the table table
reservation_time: Reservation time
status: Reservation status, 0 means not used, 1 means used
② customer table
id: self-increasing primary key
name: Customer name
phone: Customer mobile phone number
email: Customer email address
③table table
id: auto-increment primary key
table_number:Table number
table_name:Table name
capacity:Table capacity
- Reservation function implementation
In the reservation In the system, functions such as table reservation and table status query need to be implemented.
(1) Table reservation
Here is taking a new reservation as an example. Before making a reservation, you need to first determine whether the table has been reserved, determine whether the reservation is successful and return the corresponding information.
$conn = mysqli_connect("localhost", "root", "root", "test_db");
if (!$conn) {
die( "Connection failed: " . mysqli_connect_error());
}
$reservation_time = $_POST['reservation_time'];
$customer_name = $_POST['customer_name'];
$ customer_phone = $_POST['customer_phone'];
$table_id = $_POST['table_id'];
$sql_check = "SELECT * FROM reservation WHERE table_id='$table_id' AND reservation_time='$ reservation_time'";
$result_check = mysqli_query($conn, $sql_check);
if (mysqli_num_rows($result_check) > 0) {
echo 'The table has been reserved at this time , please select another time. ';
} else {
$sql_customer = "INSERT INTO customer (name, phone) VALUES ('$customer_name', '$customer_phone')";
mysqli_query($conn, $sql_customer);
$customer_id = mysqli_insert_id($conn);
$sql_reservation = "INSERT INTO reservation (customer_id, table_id, reservation_time, status) VALUES ('$customer_id', '$table_id', '$reservation_time', 0)" ;
mysqli_query($conn, $sql_reservation);
echo 'Reservation successful. ';
}
mysqli_close($conn);
?>
(2) Query the table status
You can query the table status by querying the reservation table To achieve this, 0 means not used, 1 means used.
$conn = mysqli_connect("localhost", "root", "root", "test_db");
if (!$conn) {
die( "Connection failed: " . mysqli_connect_error());
}
$table_id = $_POST['table_id'];
$reservation_time = $_POST['reservation_time'];
$sql_check = "SELECT * FROM reservation WHERE table_id='$table_id' AND reservation_time='$reservation_time'";
$result_check = mysqli_query($conn, $sql_check);
if (mysqli_num_rows ($result_check) > 0) {
$row = mysqli_fetch_assoc($result_check);
$status = $row['status'];
if ($status == 0) {
echo '该餐桌未被预约。';
} else {
echo '该餐桌已被预约。';
}
} else {
echo 'The table has not been reserved. ';
}
mysqli_close($conn);
?>
3. Implementation of the ordering system
In the ordering system, it is necessary Realize menu management, order management, payment and other functions.
- Database design
Create database tables: dish, order
① dish table
id: self-increasing primary key
dish_name: dish name
price: dish price
description: dish description
image: dish picture
② order table
id: self-increasing primary key
customer_id: foreign key, associated with the id field of the customer table
table_id: foreign key, associated with the id field of the table table
dish_info: order Details
status: order status, 0 means not completed, 1 means completed
order_time: order time
total_price: total order price
- Dish Management
Dish management includes operations such as adding, modifying, and deleting dishes.
(1) Newly added dishes
Here is an example of new dishes.
$conn = mysqli_connect("localhost", "root", "root", "test_db");
if (!$conn) {
die( "Connection failed: " . mysqli_connect_error());
}
$dish_name = $_POST['dish_name'];
$price = $_POST['price'];
$ description = $_POST['description'];
$image = $_POST['image'];
$sql = "INSERT INTO dish (dish_name, price, description, image) VALUES ('$ dish_name', $price, '$description', '$image')";
if (mysqli_query($conn, $sql)) {
echo "Dish added successfully.";
} else {
echo "Error: " . mysqli_error($conn);
}
mysqli_close($conn);
?>
(2) Dishes modification
Here is an example of modifying the price of a dish.
$conn = mysqli_connect("localhost", "root", "root", "test_db");
if (!$conn) {
die( "Connection failed: " . mysqli_connect_error());
}
$id = $_POST['id'];
$price = $_POST['price'];
$sql = "UPDATE dish SET price=$price WHERE id=$id";
if (mysqli_query($conn, $sql)) {
echo "The dish was modified successfully.";
} else {
echo "Error: " . mysqli_error($conn);
}
mysqli_close($conn);
?>
(3 )Dish deletion
Here is an example of deleting a dish.
$conn = mysqli_connect("localhost", "root", "root", "test_db");
if (!$conn) {
die( "Connection failed: " . mysqli_connect_error());
}
$id = $_POST['id'];
$sql = "DELETE FROM dish WHERE id=$id ";
if (mysqli_query($conn, $sql)) {
echo "The dish was deleted successfully.";
} else {
echo "Error: " . mysqli_error($conn );
}
mysqli_close($conn);
?>
- Order management
Order management includes the addition of orders , modify, delete and other operations.
(1) New orders
Here we take new orders as an example.
$conn = mysqli_connect("localhost", "root", "root", "test_db");
if (!$conn) {
die( "Connection failed: " . mysqli_connect_error());
}
$table_id = $_POST['table_id'];
$customer_id = $_POST['customer_id'];
$ dish_info = $_POST['dish_info'];
$total_price = $_POST['total_price'];
$sql = "INSERT INTO order
(customer_id, table_id, dish_info, status, order_time, total_price) VALUES ('$customer_id', '$table_id', '$dish_info', 0, now(), $total_price)";
if (mysqli_query($conn, $sql) ) {
echo "The order was added successfully.";
} else {
echo "Error: " . mysqli_error($conn);
}
mysqli_close($conn);
?>
(2) Order modification
Here is an example of modifying the order status.
$conn = mysqli_connect("localhost", "root", "root", "test_db");
if (!$conn) {
die( "Connection failed: " . mysqli_connect_error());
}
$id = $_POST['id'];
$status = $_POST['status'];
$sql = "UPDATE order
SET status=$status WHERE id=$id";
if (mysqli_query($conn, $sql)) {
echo "Order Modification successful.";
} else {
echo "Error: " . mysqli_error($conn);
}
mysqli_close($conn);
?>
(3) Order deletion
Here is an example of deleting an order.
$conn = mysqli_connect("localhost", "root", "root", "test_db");
if (!$conn) {
die( "Connection failed: " . mysqli_connect_error());
}
$id = $_POST['id'];
$sql = "DELETE FROM order
WHERE id=$id";
if (mysqli_query($conn, $sql)) {
echo "The order was deleted successfully.";
} else {
echo "Error: " . mysqli_error($conn);
}
mysqli_close($conn);
?>
4. Summary
Reservation ordering function Implementation requires the combination of the reservation system and the ordering system. The reservation system is mainly responsible for managing the customer's reservation information, while the ordering system is mainly responsible for the customer's ordering operation, dish management, etc. In PHP development, these functions can be realized by designing the database and using relevant PHP functions. At the same time, attention must be paid to data security and user experience to make the reservation and ordering function more complete.
The above is the detailed content of How to use PHP to develop the reservation ordering function of the food ordering system?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


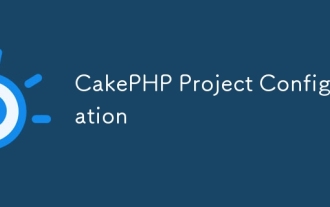
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
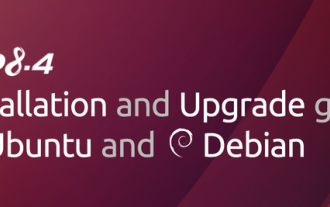
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
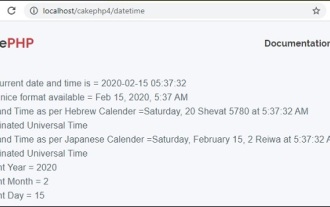
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
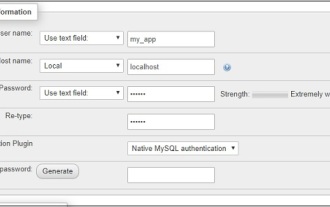
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
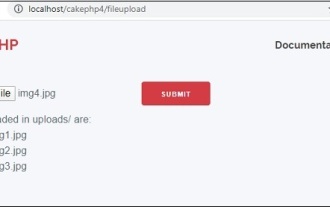
To work on file upload we are going to use the form helper. Here, is an example for file upload.
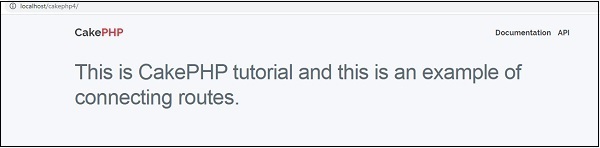
In this chapter, we are going to learn the following topics related to routing ?
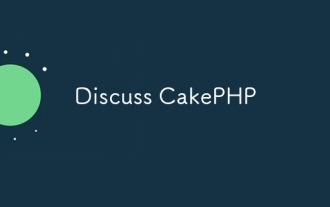
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
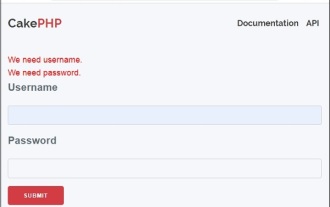
Validator can be created by adding the following two lines in the controller.
