


Implementation method of dish recommendation function in food ordering system developed with Go language
Go language development method to implement the dish recommendation function in the ordering system
With the development of Internet technology, mobile ordering systems have gradually become popular. In the ordering system, the implementation of the dish recommendation function plays an important role in improving user experience and increasing sales. This article will introduce how to use Go language to implement the dish recommendation function and provide specific code examples.
1. Data preparation
Before implementing the dish recommendation function, you first need to prepare relevant data. This data includes the name, type, taste, heat and other information of the dishes. The dish information can be represented by a structure, as shown below:
type Dish struct { Name string Type string Taste string Hot int }
We can use a slice to store all the dish information, as shown below:
var dishes = []Dish{ {"宫保鸡丁", "川菜", "麻辣", 5}, {"红烧肉", "家常菜", "咸甜", 4}, {"清蒸鱼", "海鲜", "清淡", 3}, // 其他菜品信息 }
2. Calculate dish recommendations Degree
Before implementing the dish recommendation function, it is necessary to calculate the recommendation degree of each dish in order to determine the recommended dish. Recommendation can be calculated based on the popularity, type, taste and other factors of the dish. The following is an example of a simple recommendation degree calculation function:
func calcRecommendation(d Dish) float64 { // 根据热度、类型、口味等因素来计算推荐度 return float64(d.Hot) * 0.3 + float64(d.Type=="川菜") * 0.2 + float64(d.Taste=="麻辣") * 0.3 }
3. Implementation of dish recommendation function
With the recommendation degree of dishes, dishes can be recommended according to the user's needs. It can be filtered according to the type and taste preferred by the user, and sorted in descending order according to the degree of recommendation, and finally a list of recommended dishes is returned to the user. The following is an example implementation of the dish recommendation function:
func recommendDishes(dishes []Dish, preferenceType string, preferenceTaste string) []Dish { var recommendations []Dish for _, d := range dishes { if d.Type == preferenceType && d.Taste == preferenceTaste { recommendations = append(recommendations, d) // 满足用户偏好的菜品加入推荐列表 } } // 按照推荐度降序排列 sort.Slice(recommendations, func(i, j int) bool { return calcRecommendation(recommendations[i]) > calcRecommendation(recommendations[j]) }) return recommendations }
4. Usage example
Next, we can use the above function to implement the dish recommendation function and demonstrate it. The following is an example of usage:
func main() { preferenceType := "川菜" preferenceTaste := "麻辣" recommendations := recommendDishes(dishes, preferenceType, preferenceTaste) fmt.Println("根据您的偏好为您推荐以下菜品:") for _, d := range recommendations { fmt.Printf("菜品名称:%s 推荐度:%f ", d.Name, calcRecommendation(d)) } }
In the above code, we set the user's preference type to "Sichuan Cuisine" and the taste to "Spicy", and then call the recommendDishes
function to obtain recommended dishes . Finally, the recommended menu list is output to the user.
Summary
This article introduces how to use Go language to implement the dish recommendation function. By calculating the recommendation degree of dishes and filtering and sorting according to user preferences, we can implement a simple and effective dish recommendation system. Of course, this is just a simple example. In actual applications, recommendations can also be made based on more factors, such as user history orders, satisfaction ratings, etc. I hope that through the introduction of this article, readers will have an understanding of how to implement the dish recommendation function in the Go language, and it can help everyone better implement the dish recommendation function when developing an ordering system.
The above is the detailed content of Implementation method of dish recommendation function in food ordering system developed with Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


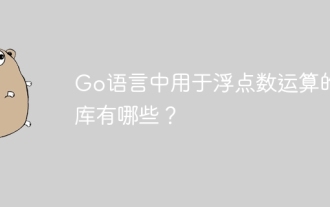
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
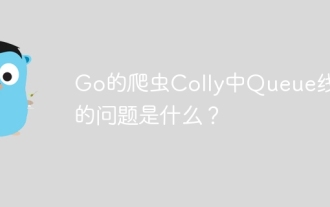
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
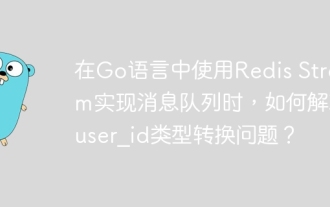
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
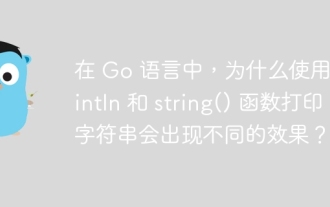
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
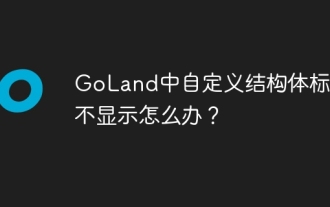
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
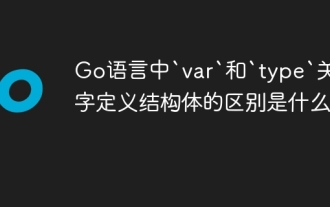
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
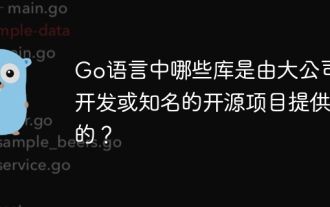
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
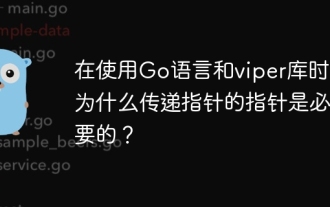
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
