


What are the characteristics of the dish liking function of the door-to-door cooking system developed using Go language?
What are the characteristics of the dish liking function of the door-to-door cooking system developed using Go language?
In modern society, as the pace of life accelerates, more and more people choose to have professional chefs come to their homes to cook delicious food for them. In order to meet this demand, we can use Go language to develop a door-to-door cooking system. In this system, the function of liking dishes is a very important part.
The function of dish like function is to allow users to evaluate and like the dishes in the door-to-door cooking system, so that other users can choose their favorite dishes based on the number of likes. When using Go language to develop this function, we can consider the following features:
- User identification and authorization:
In the dish like function, we need to ensure that only logged in users can Perform a like operation. Therefore, we need to implement user identification and authorization functions in the system. The user's unique identifier (such as user ID, mobile phone number, etc.) can be obtained through user registration and login, and identity verification can be performed when the user performs a like operation. - Low latency and high concurrency:
In actual applications, the dish liking function may have a large number of concurrent operations. In order to support fast response and processing in high concurrency situations, we can use the Go language's goroutine and channel to implement asynchronous processing. By putting the like request into a buffer channel, and then having multiple coroutines take the request out of the channel for processing, the concurrency performance of the system can be effectively improved.
The following is a simple sample code to demonstrate how to use Go language to implement the dish like function:
package main import ( "fmt" "sync" ) type Dish struct { ID int Name string Likes int likedUser map[string]bool // 存储用户点赞信息 lock sync.RWMutex // 读写锁,用于并发保护 } func (d *Dish) Like(userID string) { d.lock.Lock() defer d.lock.Unlock() if _, ok := d.likedUser[userID]; !ok { d.likedUser[userID] = true d.Likes++ } } func main() { d := &Dish{ ID: 1, Name: "红烧肉", Likes: 0, likedUser: make(map[string]bool), } go func() { for i := 0; i < 100; i++ { d.Like(fmt.Sprintf("user%d", i)) } }() go func() { for i := 0; i < 100; i++ { d.Like(fmt.Sprintf("user%d", i)) } }() // 等待异步点赞操作完成 for d.Likes < 200 { } fmt.Printf("菜品 %s 点赞数:%d ", d.Name, d.Likes) }
In the above sample code, we define a Dish structure , used to represent dishes. The structure contains the dish's ID, name, number of likes, and a likedUser map that stores user like information. When liking, we use a read-write lock to protect the concurrent access of likedUser, and record whether the user has liked it through the key-value pair of the map. The like operation is completed by checking and updating likedUser.
In the main function, we use two coroutines to like the dishes 100 times. Since the like operation is asynchronous, in order to wait for the like operation to complete, we use a simple loop to determine whether the number of likes has reached 200.
To sum up, using the Go language to develop the dish liking function of the door-to-door cooking system has the characteristics of user identification and authorization, low latency and high concurrency. By making reasonable use of the language features and concurrency mechanism of the Go language, we can implement a stable and efficient like function and provide users with a better user experience.
The above is the detailed content of What are the characteristics of the dish liking function of the door-to-door cooking system developed using Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
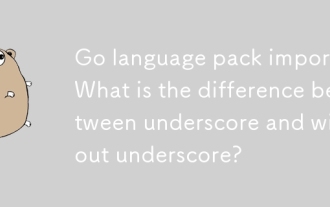
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t
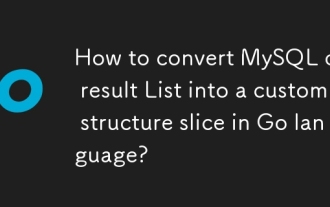
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
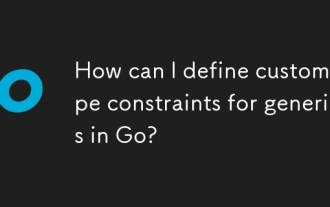
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
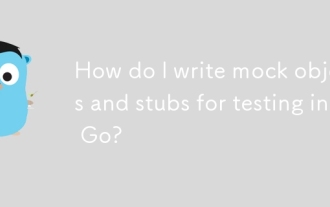
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
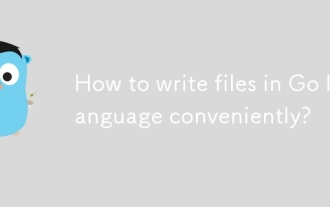
This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
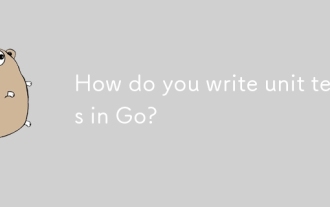
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
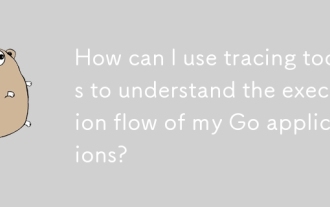
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
