How to implement a simple file management system using C++?
How to use C to implement a simple file management system?
Overview:
The file management system is a very important functional module in the computer. It is responsible for creating, modifying, deleting and other operations on the files in the computer. This article will introduce how to use C programming language to implement a simple file management system, through which the basic management functions of files can be realized.
1. Function implementation:
(1) File creation: Using the fstream library in C, you can create files. First, you need to include the
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
(2) File reading: Using the ifstream class in C, the file reading operation can be realized. The file contents can be read line by line through the open() function of a file object and its member function getline(). The sample code is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
|
(3) File modification: Using the ofstream class in C, you can modify the file. Contents can be written to a file through the open() function of a file object and its member function write(). The sample code is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
(4) File deletion: Use the remove() function in C to delete files. The sample code is as follows:
1 2 3 4 5 6 7 8 9 10 11 |
|
2. Testing and summary:
Integrate the above code into a file management system class to implement a simple file management system. Through this system, you can create, read, modify and delete files. When testing the file management system, you can create, read, modify and delete different file names to achieve operations on different files. By reading the code, you can find that C provides a wealth of file-related classes and functions, which can facilitate file operations.
In short, using C programming language you can implement a simple file management system that can create, read, modify and delete files. Through the implementation of this system, the basic concepts and principles of file management can be better understood and mastered. At the same time, the functions of the file management system can also be expanded through further design and improvement to make it more complete and practical.
The above is the detailed content of How to implement a simple file management system using C++?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


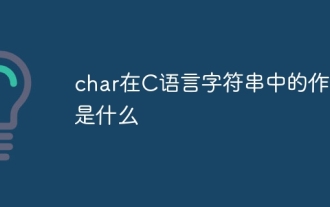
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
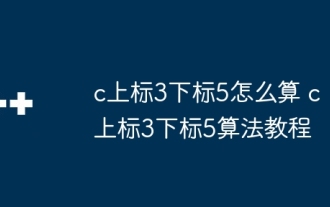
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
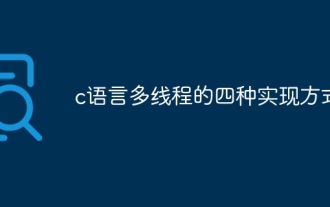
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
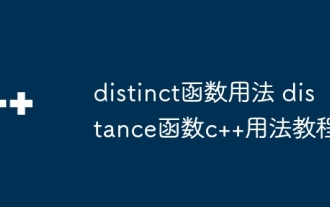
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
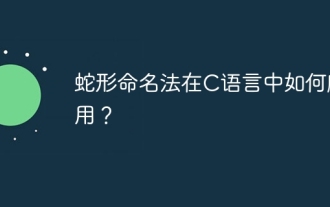
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
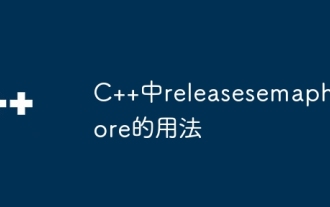
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
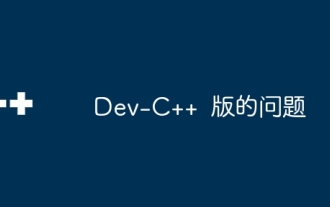
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
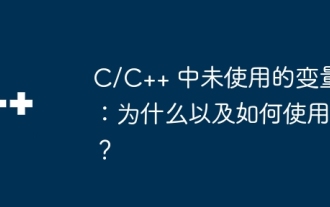
In C/C code review, there are often cases where variables are not used. This article will explore common reasons for unused variables and explain how to get the compiler to issue warnings and how to suppress specific warnings. Causes of unused variables There are many reasons for unused variables in the code: code flaws or errors: The most direct reason is that there are problems with the code itself, and the variables may not be needed at all, or they are needed but not used correctly. Code refactoring: During the software development process, the code will be continuously modified and refactored, and some once important variables may be left behind and unused. Reserved variables: Developers may predeclare some variables for future use, but they will not be used in the end. Conditional compilation: Some variables may only be under specific conditions (such as debug mode)
