


How to use Go language to write the delivery staff working time management module in the door-to-door cooking system?
How to use Go language to write the delivery staff working time management module in the door-to-door cooking system?
With the booming development of the takeout market, the door-to-door cooking system has also become a convenient choice in people's lives. In the door-to-door cooking system, the delivery person is a crucial part. Good working time management is very important for delivery staff's work efficiency and user experience. This article will introduce how to use Go language to write the delivery staff working time management module in the door-to-door cooking system, and provide specific code examples.
- Requirement Analysis
In the door-to-door cooking system, delivery personnel usually need to arrange delivery tasks according to the time when users place orders. The delivery staff's working hours should be within the scope of system management, and special handling of various situations needs to be taken into account. For example:
- Delivery staff should have rest and working time, and the working hours should be based on the user's Flexible adjustments according to needs.
- Delivery workers may have multiple working time models, such as full-time, part-time, etc.
- Delivery personnel may have other tasks or arrangements outside of working hours.
- Data model design
In order to implement the delivery staff working time management module, the corresponding data model needs to be designed first. You can consider using the following data structure:
type DeliveryTime struct { StartTime time.Time // 工作开始时间 EndTime time.Time // 工作结束时间 }
In the delivery person data model, add a field to represent the delivery person's working hours:
type DeliveryPerson struct { Name string // 配送员姓名 DeliveryTime []DeliveryTime // 配送员工作时间段 }
- Functional design
After completing the data model design, it is necessary to implement the relevant functions of the delivery staff working time management module:
- Add working time
- Delete working time
- Query Working hours
- Update working hours
You can define an externally exposed interface to implement the above functions:
type DeliveryPersonService interface { AddWorkTime(startTime time.Time, endTime time.Time) error RemoveWorkTime(startTime time.Time, endTime time.Time) error GetWorkTime() ([]DeliveryTime, error) UpdateWorkTime(oldStartTime, oldEndTime, newStartTime, newEndTime time.Time) error }
- Code implementation
Based on the above requirements and functional design, we can use Go language for code implementation. The following is a simple example:
type deliveryPersonService struct { deliveryPerson *DeliveryPerson } func (d *deliveryPersonService) AddWorkTime(startTime time.Time, endTime time.Time) error { d.deliveryPerson.DeliveryTime = append(d.deliveryPerson.DeliveryTime, DeliveryTime{ StartTime: startTime, EndTime: endTime, }) return nil } func (d *deliveryPersonService) RemoveWorkTime(startTime time.Time, endTime time.Time) error { for i, time := range d.deliveryPerson.DeliveryTime { if time.StartTime == startTime && time.EndTime == endTime { d.deliveryPerson.DeliveryTime = append(d.deliveryPerson.DeliveryTime[:i], d.deliveryPerson.DeliveryTime[i+1:]...) return nil } } return errors.New("work time not found") } func (d *deliveryPersonService) GetWorkTime() ([]DeliveryTime, error) { return d.deliveryPerson.DeliveryTime, nil } func (d *deliveryPersonService) UpdateWorkTime(oldStartTime, oldEndTime, newStartTime, newEndTime time.Time) error { for i, time := range d.deliveryPerson.DeliveryTime { if time.StartTime == oldStartTime && time.EndTime == oldEndTime { d.deliveryPerson.DeliveryTime[i] = DeliveryTime{ StartTime: newStartTime, EndTime: newEndTime, } return nil } } return errors.New("work time not found") }
- Usage example
func main() { // 创建一个配送员对象 deliveryPerson := &DeliveryPerson{ Name: "John", DeliveryTime: []DeliveryTime{}, } // 创建配送员服务对象 service := &deliveryPersonService{ deliveryPerson: deliveryPerson, } // 添加工作时间 service.AddWorkTime(time.Date(2022, time.January, 1, 9, 0, 0, 0, time.UTC), time.Date(2022, time.January, 1, 18, 0, 0, 0, time.UTC)) // 查询工作时间 workTime, _ := service.GetWorkTime() fmt.Println("Work Time:", workTime) // 删除工作时间 service.RemoveWorkTime(time.Date(2022, time.January, 1, 9, 0, 0, 0, time.UTC), time.Date(2022, time.January, 1, 18, 0, 0, 0, time.UTC)) // 查询工作时间 workTime, _ = service.GetWorkTime() fmt.Println("Work Time:", workTime) }
The above code example demonstrates how to use Go language to write delivery staff working time management in the door-to-door cooking system module. By defining the data model and implementing related functions, the delivery staff's working hours can be easily managed and external interfaces can be provided for use by other modules. Writing such a module can effectively improve the work efficiency and service quality of delivery personnel, and provide users with a better door-to-door cooking experience.
The above is the detailed content of How to use Go language to write the delivery staff working time management module in the door-to-door cooking system?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


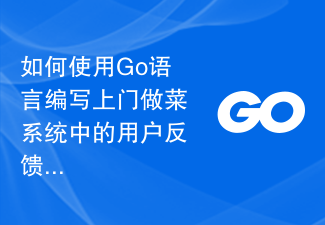
How to use Go language to write the user feedback module in the door-to-door cooking system? With the rise of takeout and door-to-door services, more and more users choose to enjoy delicious food at home. For door-to-door cooking services, user feedback is particularly important, which can help improve service quality and user satisfaction. This article will introduce how to use Go language to write the user feedback module in the door-to-door cooking system, and provide specific code examples. Database design and creation First, we need to design a database to store user feedback information. Suppose we have a feed called
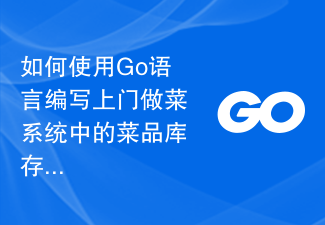
How to use Go language to write the dish inventory management module in the door-to-door cooking system? With the rise of takeout and home cooking, more and more people are choosing to enjoy delicious food at home. As a platform that provides door-to-door cooking services, food inventory management is an integral part. In this article, we will introduce how to use Go language to write the dish inventory management module in the door-to-door cooking system, and provide specific code examples. The functions of the dish inventory management module mainly include adding, querying, modifying and deleting dishes. First, we need to define a dish structure.
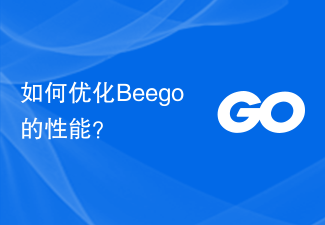
Beego is one of the commonly used web frameworks in the Go language. It has the advantages of rapid development, binary deployment, and high concurrency. However, in a high concurrent request environment, the performance optimization needs of the Beego framework are highlighted. This article will introduce how to optimize Beego's performance through reasonable configuration, optimized code, cache, etc. 1. Use an efficient caching mechanism. Caching can greatly improve application performance and reduce the number of database queries. The Beego framework’s caching mechanism is also very simple and easy to use, and can be applied to different scales.
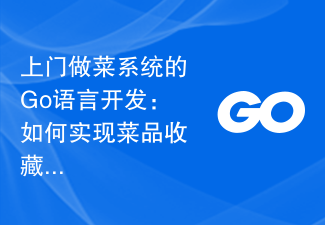
Go language development of door-to-door cooking system: How to implement the dish collection function? With the improvement of living standards, more and more people choose to have chefs come to cook for them. The door-to-door cooking system emerged as the times require, providing users with a convenient service platform. When developing such a system, the dish collection function is one of the most important functions. This article will introduce how to use Go language to develop a door-to-door cooking system and implement the dish collection function. 1. Project requirements analysis Before starting development, we first need to understand the specific requirements for the dish collection function.
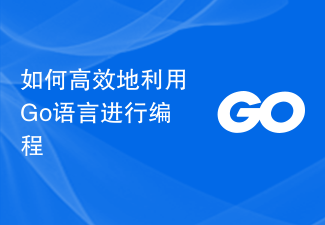
How to improve the efficiency of Go language programming? Why is Go language so important to programmers? With the rapid popularity of Go language in the field of software development, more and more developers are paying attention to this programming language. The Go language has been widely praised for its simplicity, efficiency, and ease of use, and has gradually become a mainstream programming language. So, how can we effectively use Go language for programming? 1. Make full use of the concurrency features of Go language. The concurrency model of Go language is one of its biggest features. Through goroutine and
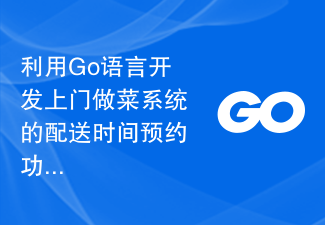
With the continuous development of society, people have higher and higher requirements for the quality of life and convenience. In this context, home catering services have attracted more and more people's attention, especially door-to-door cooking services have become the choice of many people. However, how to improve the efficiency and quality of door-to-door cooking services has become a problem that needs to be solved. In this regard, there are many innovations in using the Go language to develop the delivery time reservation function. 1. Efficient development using Go language. As a static language, Go language has high efficiency and concurrency, and is very suitable for large-scale
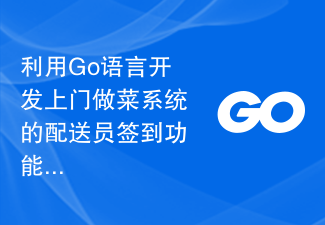
What are the innovations in using the Go language to develop the delivery person check-in function of the door-to-door cooking system? With the rapid development of the food delivery industry, door-to-door cooking services have become an increasingly popular choice in daily life. In order to provide a better user experience and improve delivery efficiency, the development of door-to-door cooking systems must have innovative delivery staff check-in functions. This article will explore the innovation of using Go language to develop this function and provide specific code examples. 1. Multi-terminal support The check-in function of the delivery person in the traditional door-to-door cooking system usually only supports operation on the mobile phone. And profit
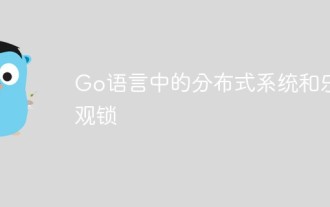
Go language is an efficient programming language that is increasingly used in distributed systems. At the same time, the optimistic locking mechanism has also become an important tool for developers to deal with concurrency issues. This article will explore distributed systems and optimistic locking in the Go language. 1. What is a distributed system? Distributed System refers to a system composed of multiple computers that are connected to each other through a network to complete tasks together. Distributed systems can improve system reliability and throughput. in distributed
