Vue development experience sharing: how to automate code testing
Vue development experience sharing: How to conduct automated testing of code
With the rapid development of front-end development and the increasing demand for continuous integration, automated testing of code has become indispensable. A missing part. As a popular front-end framework, Vue also needs automated testing to ensure the quality and stability of the code. This article will share some experiences and techniques for automated testing in Vue development.
1. Choose the appropriate testing tool
The Vue project has many testing tools to choose from, common ones include Jest, Mocha, and Karma. These tools can be used to write and run test cases, and provide a rich set of test assertions and auxiliary functions. Choose a testing tool that best fits the project's specific needs and the development team's preferences.
2. Write unit test cases
Unit testing is the most basic type of automated testing and is used to test the smallest unit in the code. In Vue, the smallest unit can be a component, a method or a functional module. When writing unit test cases, you need to consider covering various situations and boundary conditions in the code to ensure the correctness and robustness of the code.
The following is a simple example:
import { mount } from '@vue/test-utils' import MyComponent from '@/components/MyComponent.vue' describe('MyComponent', () => { test('renders correctly', () => { const wrapper = mount(MyComponent) expect(wrapper.text()).toContain('Hello, World!') }) })
In the above example, we use the mount
function provided by @vue/test-utils
to mount the component and use expect
assertions to determine whether the component renders as expected.
3. Use snapshot testing
In addition to writing assertions to determine whether the component renders as expected, you can also use snapshot testing to ensure that the rendering results of the component are consistent under different circumstances. Snapshot testing records the rendering results of the component and saves them in a file. The next time the test is run, the current rendering results will be compared with the results in the snapshot file to determine whether the component has changed.
import { shallowMount } from '@vue/test-utils' import MyComponent from '@/components/MyComponent.vue' describe('MyComponent', () => { test('renders correctly', () => { const wrapper = shallowMount(MyComponent) expect(wrapper.html()).toMatchSnapshot() }) })
4. Conduct component integration testing
In addition to unit testing, component integration testing is also required to verify whether the interaction between different components and the overall function are normal. Integration testing can be performed by simulating user behavior, triggering events, etc. Common integration testing tools include Cypress and Nightwatch.
5. Write a test coverage report
The test coverage report is one of the important indicators to measure the quality of automated testing. Through the test coverage report, you can see which code is covered by the test cases and which code is not covered. In Vue, you can use tools such as Istanbul to generate test coverage reports, and perform code optimization and add test cases based on the reports.
Summary
Automated testing is one of the important means to ensure code quality and stability, and is especially important for Vue development. This article introduces the experience and skills of automated testing in Vue development, including selecting testing tools, writing unit test cases, using snapshot testing, conducting component integration testing, and writing test coverage reports. I hope these experiences can help readers better conduct automated testing of Vue code and improve code quality and development efficiency.
The above is the detailed content of Vue development experience sharing: how to automate code testing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




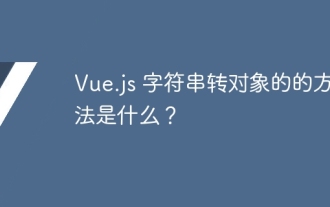
Using JSON.parse() string to object is the safest and most efficient: make sure that strings comply with JSON specifications and avoid common errors. Use try...catch to handle exceptions to improve code robustness. Avoid using the eval() method, which has security risks. For huge JSON strings, chunked parsing or asynchronous parsing can be considered for optimizing performance.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.

Vue.js is not difficult to learn, especially for developers with a JavaScript foundation. 1) Its progressive design and responsive system simplify the development process. 2) Component-based development makes code management more efficient. 3) The usage examples show basic and advanced usage. 4) Common errors can be debugged through VueDevtools. 5) Performance optimization and best practices, such as using v-if/v-show and key attributes, can improve application efficiency.

Vue.js is mainly used for front-end development. 1) It is a lightweight and flexible JavaScript framework focused on building user interfaces and single-page applications. 2) The core of Vue.js is its responsive data system, and the view is automatically updated when the data changes. 3) It supports component development, and the UI can be split into independent and reusable components.
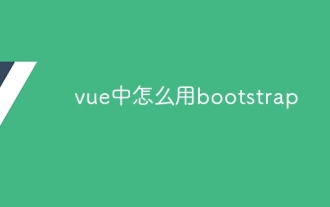
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
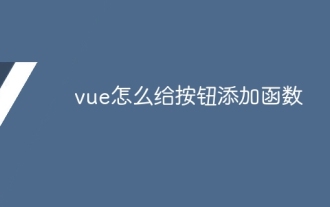
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
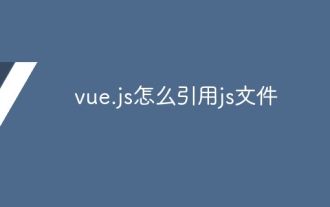
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
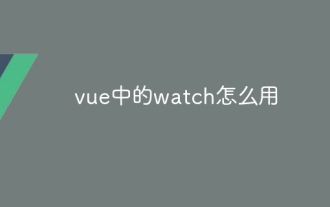
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
