How to use middleware for error handling in Laravel
How to use middleware for error handling in Laravel
Introduction:
Laravel is a popular PHP framework with a powerful error handling system. Among them, middleware is one of the core functions of Laravel. It can handle errors by executing a series of logic before or after the request enters routing processing. This article will detail how to use middleware for error handling in Laravel and provide specific code examples.
1. Create error handling middleware
First, we need to create a custom middleware to handle errors. In Laravel, you can create middleware through the following command:
php artisan make:middleware HandleErrors
This command will create a file named # in the app/Http/Middleware
directory. ##HandleErrors middleware. Next, we need to add our error handling logic in the
handle method of the middleware. The following is a simple sample code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
|
try-catch block to catch exceptions that may occur. Once an exception is caught, we can perform corresponding error handling operations in the
catch block.
In Laravel, the middleware needs to be registered in the global middleware stack of the application. This can be accomplished by following these steps:
- Open the
- app/Http/Kernel.php
file.
Add the following line of code in the - $middleware
array to register the middleware:
'error.handler' => AppHttpMiddlewareHandleErrors::class,
To apply error handling middleware to a specific route or routing group, you can use Laravel's
route method or
groupmethod. Here is an example:
1 2 3 |
|
group method to create a routing group with error handling middleware. Routes defined within this group will automatically have error handling middleware applied.
Now that we have set up the error handling middleware, we will show how to handle errors in the middleware.
UserController, which contains a
create method for creating users. The following is a sample code:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
|
Log class to record error information to the log file and return a JSON response containing the error information .
Through the above steps, we successfully used middleware for error handling. First, we created a custom middleware to handle errors and registered it with the middleware stack. We then applied the middleware to a specific route or route group and implemented specific logic for error handling. In actual development, the logic and implementation of error handling can be customized as needed.
The above is the detailed content of How to use middleware for error handling in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
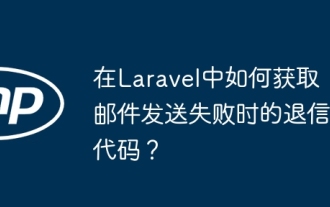
Method for obtaining the return code when Laravel email sending fails. When using Laravel to develop applications, you often encounter situations where you need to send verification codes. And in reality...
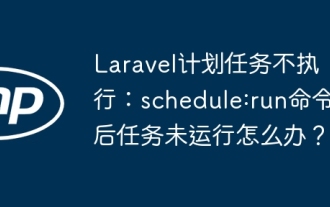
Laravel schedule task run unresponsive troubleshooting When using Laravel's schedule task scheduling, many developers will encounter this problem: schedule:run...
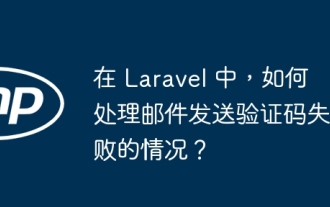
The method of handling Laravel's email failure to send verification code is to use Laravel...
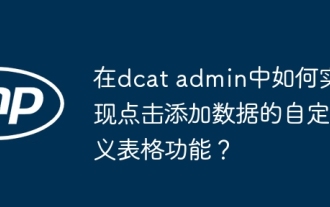
How to implement the table function of custom click to add data in dcatadmin (laravel-admin) When using dcat...
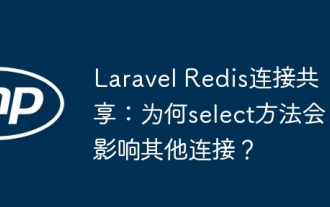
The impact of sharing of Redis connections in Laravel framework and select methods When using Laravel framework and Redis, developers may encounter a problem: through configuration...
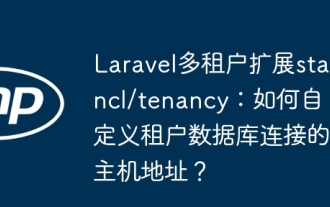
Custom tenant database connection in Laravel multi-tenant extension package stancl/tenancy When building multi-tenant applications using Laravel multi-tenant extension package stancl/tenancy,...
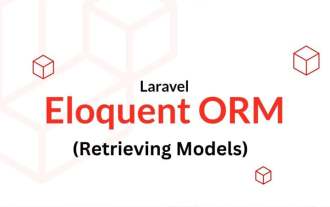
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
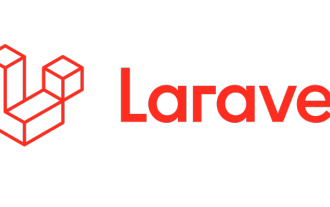
Efficiently process 7 million records and create interactive maps with geospatial technology. This article explores how to efficiently process over 7 million records using Laravel and MySQL and convert them into interactive map visualizations. Initial challenge project requirements: Extract valuable insights using 7 million records in MySQL database. Many people first consider programming languages, but ignore the database itself: Can it meet the needs? Is data migration or structural adjustment required? Can MySQL withstand such a large data load? Preliminary analysis: Key filters and properties need to be identified. After analysis, it was found that only a few attributes were related to the solution. We verified the feasibility of the filter and set some restrictions to optimize the search. Map search based on city
