How to use Laravel to implement website visit statistics function
How to use Laravel to implement website access statistics function
Introduction:
In modern website development, understanding website access is important for evaluating website performance, user behavior and Business growth is critical. There is a powerful access statistics function that can help us monitor the activity and traffic of the website in real time and provide us with key data analysis. In this article, I will introduce to you how to use the Laravel framework to implement a simple and practical website access statistics function.
Step 1: Preparation
First, we need to make sure you have the Laravel framework installed and a basic Laravel project ready. If you haven't installed it yet, you can refer to Laravel official documentation to install and create a project.
Step 2: Create a database table
We need to create a table in the database to store the website access statistics. In Laravel's migration file, we can define the structure of the database table. Open the command line tool and enter the following command to create a migration file:
php artisan make:migration create_visit_stats_table --create=visit_stats
After execution, a new migration file will be generated in the database/migrations
directory for creation visit_stats
table. Open the file and add the following fields:
public function up() { Schema::create('visit_stats', function (Blueprint $table) { $table->bigIncrements('id'); $table->string('ip_address'); $table->string('url'); $table->timestamp('visited_at'); $table->timestamps(); }); }
Then, run the migration command to create the table:
php artisan migrate
Step 3: Create access statistics middleware
Using Laravel's middleware, we can Easily log the details of every request, including IP address, URL and access time. Create a middleware named VisitStatsMiddleware
and add the following code:
namespace AppHttpMiddleware; use Closure; use AppVisitStat; use IlluminateSupportFacadesAuth; class VisitStatsMiddleware { public function handle($request, Closure $next) { $visitStat = new VisitStat(); $visitStat->ip_address = $request->ip(); $visitStat->url = $request->url(); $visitStat->visited_at = now(); $visitStat->save(); return $next($request); } }
Step 4: Register the middleware
Open the app/Http/Kernel.php
file , add the middleware to the $routeMiddleware
array:
protected $routeMiddleware = [ // ...其他中间件... 'visit.stats' => AppHttpMiddlewareVisitStatsMiddleware::class, ];
Step 5: Apply Middleware
We need to select the route to apply the middleware. Open the routes/web.php
file and add the corresponding route to your route list. For example:
Route::group(['middleware' => ['visit.stats']], function () { // 这里是需要应用中间件的路由 Route::get('/', 'HomeController@index'); // ...其他路由... });
Step 6: Display access statistics
In your project, you can use Laravel's models and views to display statistics. For example, you can create a VisitStat
model and use the model in a view to display visit statistics.
namespace App; use IlluminateDatabaseEloquentModel; class VisitStat extends Model { // }
In the controller, you can query and pass statistical data to the view:
namespace AppHttpControllers; use IlluminateHttpRequest; use AppVisitStat; class StatsController extends Controller { public function index() { $stats = VisitStat::orderBy('visited_at', 'desc')->get(); return view('stats.index', ['stats' => $stats]); } }
In the view, you can use the Blade template engine to display statistical data:
@foreach($stats as $stat) <p>{{ $stat->url }} - {{ $stat->visited_at }}</p> @endforeach
Conclusion:
Through the above steps, we have implemented a simple website visit statistics function. Now you can record and display visit statistics on your website. Of course, this is just a basic implementation example, and you can further customize and extend it according to your own needs. Laravel provides a wealth of functions and tools to help you build a more powerful and flexible access statistics system. I hope this article is helpful to you, and I wish you success in using Laravel for website development!
The above is the detailed content of How to use Laravel to implement website visit statistics function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


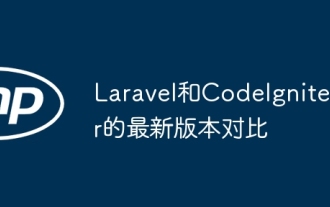
The latest versions of Laravel 9 and CodeIgniter 4 provide updated features and improvements. Laravel9 adopts MVC architecture and provides functions such as database migration, authentication and template engine. CodeIgniter4 uses HMVC architecture to provide routing, ORM and caching. In terms of performance, Laravel9's service provider-based design pattern and CodeIgniter4's lightweight framework give it excellent performance. In practical applications, Laravel9 is suitable for complex projects that require flexibility and powerful functions, while CodeIgniter4 is suitable for rapid development and small applications.
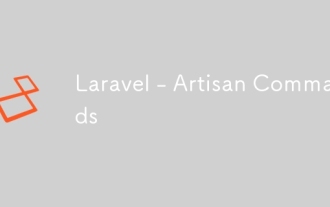
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?
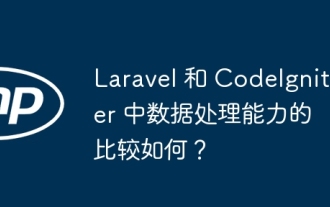
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
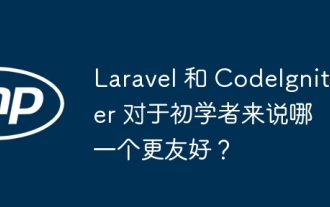
For beginners, CodeIgniter has a gentler learning curve and fewer features, but covers basic needs. Laravel offers a wider feature set but has a slightly steeper learning curve. In terms of performance, both Laravel and CodeIgniter perform well. Laravel has more extensive documentation and active community support, while CodeIgniter is simpler, lightweight, and has strong security features. In the practical case of building a blogging application, Laravel's EloquentORM simplifies data manipulation, while CodeIgniter requires more manual configuration.
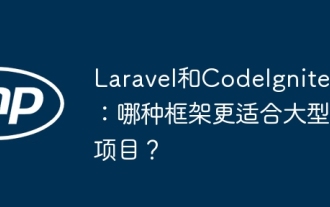
When choosing a framework for large projects, Laravel and CodeIgniter each have their own advantages. Laravel is designed for enterprise-level applications, offering modular design, dependency injection, and a powerful feature set. CodeIgniter is a lightweight framework more suitable for small to medium-sized projects, emphasizing speed and ease of use. For large projects with complex requirements and a large number of users, Laravel's power and scalability are more suitable. For simple projects or situations with limited resources, CodeIgniter's lightweight and rapid development capabilities are more ideal.
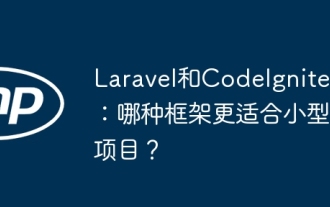
For small projects, Laravel is suitable for larger projects that require strong functionality and security. CodeIgniter is suitable for very small projects that require lightweight and ease of use.
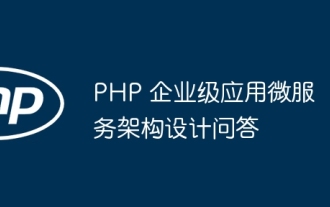
Microservice architecture uses PHP frameworks (such as Symfony and Laravel) to implement microservices and follows RESTful principles and standard data formats to design APIs. Microservices communicate via message queues, HTTP requests, or gRPC, and use tools such as Prometheus and ELKStack for monitoring and troubleshooting.
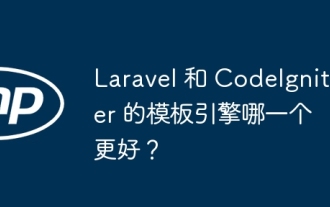
Comparing Laravel's Blade and CodeIgniter's Twig template engine, choose based on project needs and personal preferences: Blade is based on MVC syntax, which encourages good code organization and template inheritance. Twig is a third-party library that provides flexible syntax, powerful filters, extended support, and security sandboxing.
