Learn about design patterns and best practices in JavaScript
With the continuous development of JavaScript and the expansion of its application scope, more and more developers are beginning to realize the importance of design patterns and best practices. A design pattern is a software design solution that proves useful in certain situations. Best practices refer to some of the best specifications and methods that we can apply during the programming process.
In this article, we will explore design patterns and best practices in JavaScript and provide some concrete code examples. let's start!
1. Design patterns in JavaScript
- Singleton Pattern
The singleton pattern can ensure that a class has only one instance, and Provides a global access point. In JavaScript, the singleton pattern can be used to manage global state and resources.
Code example:
const Singleton = (function () { let instance; function createInstance() { const object = new Object({ name: "Singleton Object" }); return object; } return { getInstance: function () { if (!instance) { instance = createInstance(); } return instance; }, }; })(); const instance1 = Singleton.getInstance(); const instance2 = Singleton.getInstance(); console.log(instance1 === instance2); // true console.log(instance1.name); // 'Singleton Object'
- Observer Pattern
The Observer pattern allows one object (topic) to monitor another object ( Observer) and notify it of certain state changes. In JavaScript, the observer pattern can be used to achieve event management and better modularity.
Code example:
class Subject { constructor() { this.observers = []; } subscribe(observer) { this.observers.push(observer); } unsubscribe(observer) { const index = this.observers.findIndex((obs) => { return obs === observer; }); this.observers.splice(index, 1); } notify() { this.observers.forEach((observer) => { observer.update(); }); } } class Observer { constructor(name) { this.name = name; } update() { console.log(`${this.name} has been notified!`); } } const subject = new Subject(); const observer1 = new Observer("Observer 1"); const observer2 = new Observer("Observer 2"); subject.subscribe(observer1); subject.subscribe(observer2); subject.notify(); // Observer 1 has been notified! Observer 2 has been notified!
- Factory Pattern
Factory pattern can dynamically create objects based on parameters. In JavaScript, the factory pattern can be used to create objects of different types without having to expose the creation logic to the client.
Code sample:
class Shape { draw() {} } class Circle extends Shape { draw() { console.log("Drawing a Circle!"); } } class Square extends Shape { draw() { console.log("Drawing a Square!"); } } class ShapeFactory { static createShape(type) { switch (type) { case "Circle": return new Circle(); case "Square": return new Square(); default: throw new Error("Shape type not supported!"); } } } const circle = ShapeFactory.createShape("Circle"); const square = ShapeFactory.createShape("Square"); circle.draw(); // Drawing a Circle! square.draw(); // Drawing a Square!
2. Best practices in JavaScript
- Use let and const instead of var
In ES6, let and const are block-scope variables, while var is a function-scope variable. Using let and const prevents variable promotion and accidental modification of a variable's value.
- Encapsulate multiple properties and methods in one object
Encapsulating related properties and methods can make the code more readable and maintainable. Namespace-like structures can be easily created using object literals and classes.
Code example:
const myModule = { prop1: "value1", prop2: "value2", method1() { console.log("Method 1 called!"); }, method2() { console.log("Method 2 called!"); }, }; myModule.method1(); // Method 1 called!
- Avoid global variables
In JavaScript, global variables can lead to naming conflicts and code coupling. Encapsulating related variables and functions in a scope can prevent these problems.
Code example:
(function () { const a = "value1"; const b = "value2"; function doSomething() { console.log(a + b); } doSomething(); // value1value2 })();
- Use strict mode
Using strict mode can prevent some common mistakes, such as accidentally modifying global variables and forgetting to define variables . Strict mode also provides better support for future ECMAScript standards.
Code example:
"use strict"; let foo = "bar"; // OK delete foo; // Error: Delete of an unqualified identifier in strict mode.
Conclusion
Design patterns and best practices can help us better organize and manage JavaScript code, and improve readability and maintainability and reusability. In this article, we specifically discussed the Singleton, Observer, and Factory patterns, as well as best practices for variable encapsulation, global variable avoidance, block-level scoping, and strict mode. Hopefully this knowledge will help you write better JavaScript code.
The above is the detailed content of Learn about design patterns and best practices in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


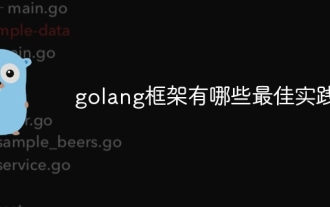
When using Go frameworks, best practices include: Choose a lightweight framework such as Gin or Echo. Follow RESTful principles and use standard HTTP verbs and formats. Leverage middleware to simplify tasks such as authentication and logging. Handle errors correctly, using error types and meaningful messages. Write unit and integration tests to ensure the application is functioning properly.
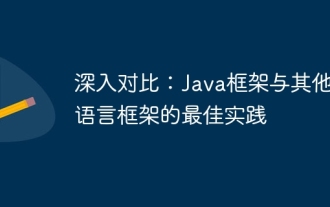
Java frameworks are suitable for projects where cross-platform, stability and scalability are crucial. For Java projects, Spring Framework is used for dependency injection and aspect-oriented programming, and best practices include using SpringBean and SpringBeanFactory. Hibernate is used for object-relational mapping, and best practice is to use HQL for complex queries. JakartaEE is used for enterprise application development, and the best practice is to use EJB for distributed business logic.
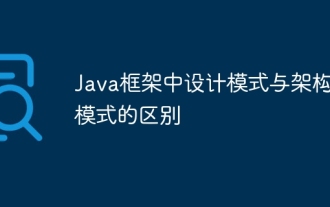
In the Java framework, the difference between design patterns and architectural patterns is that design patterns define abstract solutions to common problems in software design, focusing on the interaction between classes and objects, such as factory patterns. Architectural patterns define the relationship between system structures and modules, focusing on the organization and interaction of system components, such as layered architecture.
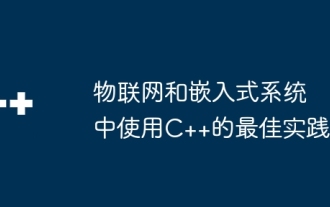
Introduction to Best Practices for Using C++ in IoT and Embedded Systems C++ is a powerful language that is widely used in IoT and embedded systems. However, using C++ in these restricted environments requires following specific best practices to ensure performance and reliability. Memory management uses smart pointers: Smart pointers automatically manage memory to avoid memory leaks and dangling pointers. Consider using memory pools: Memory pools provide a more efficient way to allocate and free memory than standard malloc()/free(). Minimize memory allocation: In embedded systems, memory resources are limited. Reducing memory allocation can improve performance. Threads and multitasking use the RAII principle: RAII (resource acquisition is initialization) ensures that the object is released at the end of its life cycle.
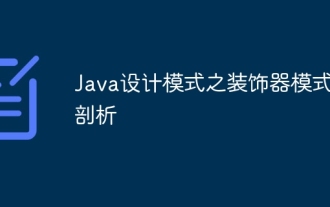
The decorator pattern is a structural design pattern that allows dynamic addition of object functionality without modifying the original class. It is implemented through the collaboration of abstract components, concrete components, abstract decorators and concrete decorators, and can flexibly expand class functions to meet changing needs. In this example, milk and mocha decorators are added to Espresso for a total price of $2.29, demonstrating the power of the decorator pattern in dynamically modifying the behavior of objects.
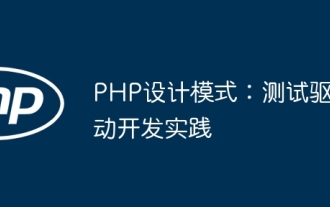
TDD is used to write high-quality PHP code. The steps include: writing test cases, describing the expected functionality and making them fail. Write code so that only the test cases pass without excessive optimization or detailed design. After the test cases pass, optimize and refactor the code to improve readability, maintainability, and scalability.
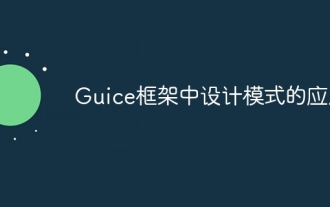
The Guice framework applies a number of design patterns, including: Singleton pattern: ensuring that a class has only one instance through the @Singleton annotation. Factory method pattern: Create a factory method through the @Provides annotation and obtain the object instance during dependency injection. Strategy mode: Encapsulate the algorithm into different strategy classes and specify the specific strategy through the @Named annotation.
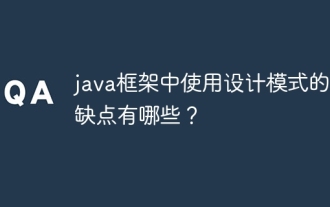
The advantages of using design patterns in Java frameworks include: enhanced code readability, maintainability, and scalability. Disadvantages include complexity, performance overhead, and steep learning curve due to overuse. Practical case: Proxy mode is used to lazy load objects. Use design patterns wisely to take advantage of their advantages and minimize their disadvantages.
