How to use Laravel middleware to secure your application
As web applications become more complex, it becomes increasingly important to secure your applications. Middleware in Laravel provides a simple and useful way to protect your application from malicious attacks while enhancing your application's security. This article explains how to use middleware in Laravel to secure your application and provides specific code examples.
What is middleware?
Middleware is code that is executed between the request and the response. Middleware allows you to transparently filter requests between routers and controllers. You can create custom middleware and link them to your application's router or controller.
Middleware is designed to solve the following problems:
- Authentication: Ensure that the user has been authenticated and has permission to access the application.
- Authorization: Determine whether the user/role has the authority to perform the requested action.
- Logging: Record key information in requests and responses for debugging or security auditing.
- Caching: Cache requests and responses to improve performance.
- Data transfer: Convert/format request and response data.
- Security: Ensure applications are protected against Cross-site Request Forgery (CSRF), Cross-site Scripting (XSS), and other security vulnerabilities.
How to create middleware?
Creating middleware in Laravel is very simple. Here are the steps to create middleware:
- Create a middleware class
First, you need to create a middleware class. You can use Artisan commands to create templates for middleware:
php artisan make:middleware MiddlewareName
- Configuring Middleware
Once the middleware class is created, you need to configure it in the HTTP kernel of your application Register middleware. This file is located in the /app/Http directory. Add your middleware to the $middleware array.
- Using Middleware in Routers/Controllers
Finally, you can attach your custom middleware to your application's router or controller. You can use the middleware method to add middleware to your router/controller. For example:
Route::get('/path', 'Controller@action') ->middleware('middlewareName');
This will cause the request to go through the middleware before reaching the controller.
Securing Your Application
Now that we understand how to create middleware, let’s explore how to use it to protect your application.
- CSRF
CSRF attack refers to an attack method in which the attacker uses the victim's login credentials (cookie or session) to complete an operation on his behalf. Unvalidated requests can easily lead to security vulnerabilities. These problems can be easily avoided using Laravel's built-in CSRF protection.
In your application, you can enable CSRF protection in the application HTTP core. You would typically do this:
// 在Http/Kernel.php文件中 class Kernel extends HttpKernel { protected $middleware = [ IlluminateFoundationHttpMiddlewareCheckForMaintenanceMode::class, IlluminateFoundationHttpMiddlewareValidatePostSize::class, AppHttpMiddlewareTrimStrings::class, IlluminateFoundationHttpMiddlewareConvertEmptyStringsToNull::class, IlluminateSessionMiddlewareStartSession::class, IlluminateViewMiddlewareShareErrorsFromSession::class, AppHttpMiddlewareVerifyCsrfToken::class, ]; }
- XSS
A cross-site scripting attack (XSS) is when an attacker executes malicious JavaScript code on the victim’s browser A way of attack. This can lead to information disclosure, injection of malicious code, and other security vulnerabilities. Middleware in Laravel can help you mitigate the damage caused by XSS attacks.
In Laravel, you can use HtmlPurifier or other third-party packages to filter your input data. Here is an example:
//在app/Http/Middleware/HtmlPurifier.php文件中 namespace AppHttpMiddleware; use Closure; use HTMLPurifier; class HtmlPurifier { public function handle($request, Closure $next) { $input = $this->purify($request->input()); $request->merge($input); return $next($request); } protected function purify(array $input) { $config = HTMLPurifier_Config::createDefault(); $purifier = new HTMLPurifier($config); foreach ($input as $key => $value) { $input[$key] = $purifier->purify($value); } return $input; } }
- Authorization
Authorization helps you determine whether the user/role has permission to perform the requested action. Laravel's built-in authorization makes this easy.
First, you need to create an authorization policy class. Use the Artisan command to generate a template for this class:
php artisan make:policy PostPolicy --model=Post
This will create a new PostPolicy class in your application's /app/Policies directory.
You also need to register the authorization policy with the service provider in your application. Define the authorization policy in your application's AuthServiceProvider:
// 在app/Providers/AuthServiceProvider.php文件中 namespace AppProviders; use AppPost; use AppPoliciesPostPolicy; use IlluminateSupportFacadesGate; use IlluminateFoundationSupportProvidersAuthServiceProvider as ServiceProvider; class AuthServiceProvider extends ServiceProvider { protected $policies = [ Post::class => PostPolicy::class, ]; // 注册策略 public function boot() { $this->registerPolicies(); } }
Next, you need to use Laravel's authorize method in your controller to verify that the user has permission to perform the requested action. For example:
public function update(Request $request, Post $post) { $this->authorize('update', $post); // 只有具备操作权限的用户才能看到以下内容 return view('posts.update', [ 'post' => $post ]); }
After authorization, only users/roles allowed by the authorization policy can view the posts.update view.
Summary
Middleware in Laravel is a powerful security tool that can help developers quickly and easily protect applications from CSRF, XSS and other security vulnerabilities. This article provides specific code examples to show how to use middleware to protect your application. If you haven't started using middleware to protect your applications, now is the time to start.
The above is the detailed content of How to use Laravel middleware to secure your application. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


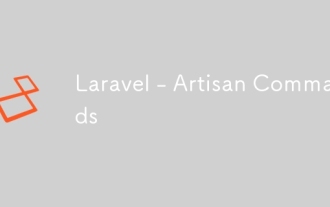
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?
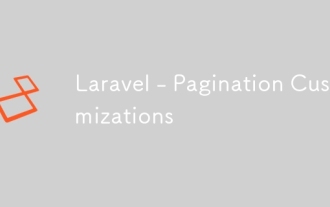
Laravel - Pagination Customizations - Laravel includes a feature of pagination which helps a user or a developer to include a pagination feature. Laravel paginator is integrated with the query builder and Eloquent ORM. The paginate method automatical
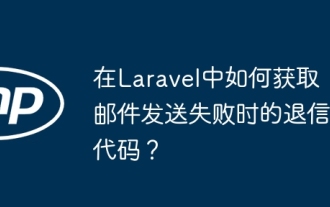
Method for obtaining the return code when Laravel email sending fails. When using Laravel to develop applications, you often encounter situations where you need to send verification codes. And in reality...
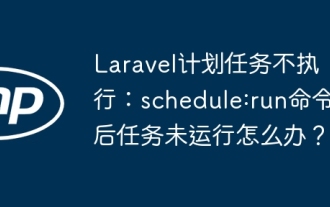
Laravel schedule task run unresponsive troubleshooting When using Laravel's schedule task scheduling, many developers will encounter this problem: schedule:run...
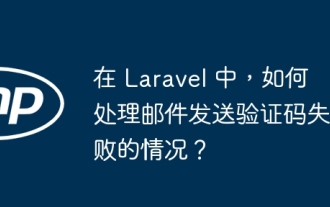
The method of handling Laravel's email failure to send verification code is to use Laravel...
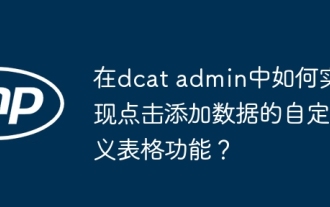
How to implement the table function of custom click to add data in dcatadmin (laravel-admin) When using dcat...
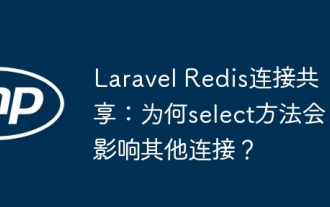
The impact of sharing of Redis connections in Laravel framework and select methods When using Laravel framework and Redis, developers may encounter a problem: through configuration...
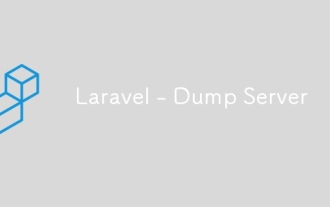
Laravel - Dump Server - Laravel dump server comes with the version of Laravel 5.7. The previous versions do not include any dump server. Dump server will be a development dependency in laravel/laravel composer file.
