How to use Laravel to implement data sorting and filtering functions
How to use Laravel to implement data sorting and filtering functions
In web applications, data sorting and filtering are very common requirements. Laravel, as a popular PHP framework, provides powerful and flexible methods to implement these functions. This article will introduce how to use built-in functions to sort and filter data in Laravel, and provide specific code examples.
1. Data sorting function
In Laravel, you can use the orderBy method to sort the query results. The orderBy method accepts a field name as a parameter and can achieve multi-level sorting by specifying one or more field names. The following is a specific example:
$users = DB::table('users') ->orderBy('name', 'asc') ->get();
In the above example, the orderBy method is used to sort the data in the users table in ascending order according to the name field. You can also use the desc keyword to sort in descending order.
$users = DB::table('users') ->orderBy('name', 'desc') ->get();
If you need to sort multiple fields, you can continue to call the orderBy method. For example, sort the name field in ascending order, and then sort by the age field in descending order if the name fields are the same.
$users = DB::table('users') ->orderBy('name', 'asc') ->orderBy('age', 'desc') ->get();
2. Data filtering function
In Laravel, you can use the where method to filter the query results. The where method accepts a field name and a value as parameters and returns results that meet the conditions. The following is a specific example:
$users = DB::table('users') ->where('name', 'John') ->get();
In the above example, the user data whose name field is John is obtained through the where method. You can also use other operators to achieve more flexible filtering, such as greater than, less than, equal to, etc.
$users = DB::table('users') ->where('age', '>', 18) ->get();
In the above example, user data whose age field is greater than 18 is obtained through the where method. At the same time, combined filtering of multiple conditions can be achieved through logical operators AND and OR.
$users = DB::table('users') ->where('age', '>', 18) ->orWhere('gender', 'female') ->get();
In the above example, the user data whose age field is greater than 18 or whose gender field is female is obtained through the where method.
3. Combined use of sorting and filtering functions
In practical applications, it is often necessary to use sorting and filtering functions at the same time to process data. In Laravel, this can be achieved by chaining the orderBy and where methods. The following is a specific example:
$users = DB::table('users') ->where('age', '>', 18) ->orderBy('name', 'asc') ->get();
In the above example, the user data whose age field is greater than 18 is obtained through the where method, and sorted in ascending order according to the name field.
To sum up, it is relatively simple to use Laravel to implement data sorting and filtering functions. These functions can be easily implemented using the built-in methods provided by the framework. In actual applications, the orderBy and where methods can be used in combination according to needs to achieve more complex data processing. I hope this article will be helpful to developers who are learning or using Laravel.
The above is the detailed content of How to use Laravel to implement data sorting and filtering functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


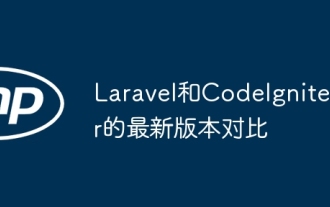
The latest versions of Laravel 9 and CodeIgniter 4 provide updated features and improvements. Laravel9 adopts MVC architecture and provides functions such as database migration, authentication and template engine. CodeIgniter4 uses HMVC architecture to provide routing, ORM and caching. In terms of performance, Laravel9's service provider-based design pattern and CodeIgniter4's lightweight framework give it excellent performance. In practical applications, Laravel9 is suitable for complex projects that require flexibility and powerful functions, while CodeIgniter4 is suitable for rapid development and small applications.
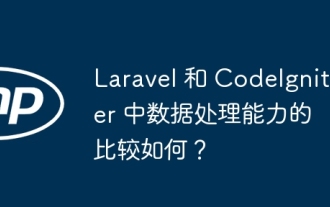
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
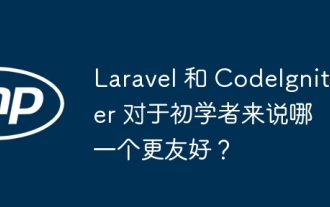
For beginners, CodeIgniter has a gentler learning curve and fewer features, but covers basic needs. Laravel offers a wider feature set but has a slightly steeper learning curve. In terms of performance, both Laravel and CodeIgniter perform well. Laravel has more extensive documentation and active community support, while CodeIgniter is simpler, lightweight, and has strong security features. In the practical case of building a blogging application, Laravel's EloquentORM simplifies data manipulation, while CodeIgniter requires more manual configuration.
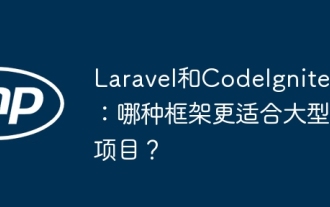
When choosing a framework for large projects, Laravel and CodeIgniter each have their own advantages. Laravel is designed for enterprise-level applications, offering modular design, dependency injection, and a powerful feature set. CodeIgniter is a lightweight framework more suitable for small to medium-sized projects, emphasizing speed and ease of use. For large projects with complex requirements and a large number of users, Laravel's power and scalability are more suitable. For simple projects or situations with limited resources, CodeIgniter's lightweight and rapid development capabilities are more ideal.
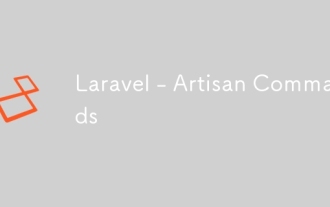
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?
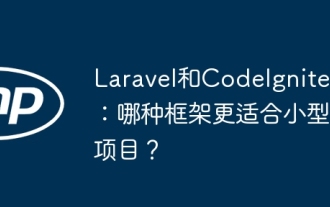
For small projects, Laravel is suitable for larger projects that require strong functionality and security. CodeIgniter is suitable for very small projects that require lightweight and ease of use.
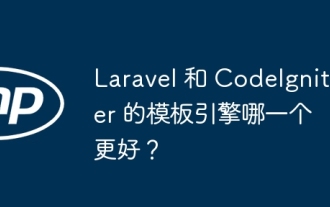
Comparing Laravel's Blade and CodeIgniter's Twig template engine, choose based on project needs and personal preferences: Blade is based on MVC syntax, which encourages good code organization and template inheritance. Twig is a third-party library that provides flexible syntax, powerful filters, extended support, and security sandboxing.
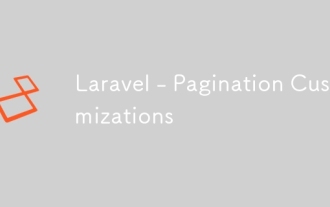
Laravel - Pagination Customizations - Laravel includes a feature of pagination which helps a user or a developer to include a pagination feature. Laravel paginator is integrated with the query builder and Eloquent ORM. The paginate method automatical
