How to write a simple student performance report generator using Java?
How to write a simple student performance report generator using Java?
The Student Performance Report Generator is a tool that can help teachers or educators quickly generate student performance reports. This article will introduce how to use Java to write a simple student performance report generator.
First, we need to define the student object and student grade object. The student object contains basic information such as the student's name and student number, while the student score object contains information such as the student's subject scores and average grade. The following is the definition of a simple student object:
public class Student { private String name; private String studentId; public Student(String name, String studentId) { this.name = name; this.studentId = studentId; } public String getName() { return name; } public String getStudentId() { return studentId; } }
Next, we need to define the data structure of the grade record. Here we use a HashMap to save student score records, where the key is the student object and the value is the score object. The following is the definition of a simple grade object:
public class Grade { private double mathGrade; private double englishGrade; // 可以按需增加其他科目的成绩 public Grade(double mathGrade, double englishGrade) { this.mathGrade = mathGrade; this.englishGrade = englishGrade; } public double getMathGrade() { return mathGrade; } public double getEnglishGrade() { return englishGrade; } }
Next, we can use an ArrayList to save the grade records of all students. The following is the implementation of a simple student score report generator:
import java.util.ArrayList; import java.util.HashMap; import java.util.Map; public class GradeReportGenerator { private ArrayList<Student> students; private HashMap<Student, Grade> studentGrades; public GradeReportGenerator() { students = new ArrayList<>(); studentGrades = new HashMap<>(); } public void addStudent(Student student, Grade grade) { students.add(student); studentGrades.put(student, grade); } public void generateReport() { for (Student student : students) { Grade grade = studentGrades.get(student); double averageGrade = (grade.getMathGrade() + grade.getEnglishGrade()) / 2; System.out.println("学生姓名:" + student.getName()); System.out.println("学号:" + student.getStudentId()); System.out.println("数学成绩:" + grade.getMathGrade()); System.out.println("英语成绩:" + grade.getEnglishGrade()); System.out.println("平均成绩:" + averageGrade); System.out.println("--------------------------"); } } }
Using this student score report generator, we can easily add student score records and generate a simple score report. The following is an example of how to use it:
public class Main { public static void main(String[] args) { GradeReportGenerator generator = new GradeReportGenerator(); Student student1 = new Student("张三", "2021001"); Grade grade1 = new Grade(94.5, 88.0); generator.addStudent(student1, grade1); Student student2 = new Student("李四", "2021002"); Grade grade2 = new Grade(87.0, 78.5); generator.addStudent(student2, grade2); generator.generateReport(); } }
The above code will generate the following score report:
学生姓名:张三 学号:2021001 数学成绩:94.5 英语成绩:88.0 平均成绩:91.25 -------------------------- 学生姓名:李四 学号:2021002 数学成绩:87.0 英语成绩:78.5 平均成绩:82.75 --------------------------
Through this simple example, we can see how to use Java to write a simple student score Report generator. You can extend this generator as needed to accommodate more complex requirements. Hope this article helps you!
The above is the detailed content of How to write a simple student performance report generator using Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


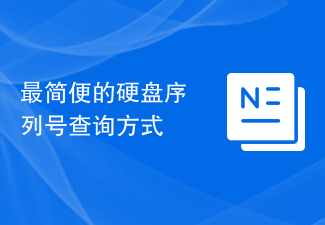
The hard disk serial number is an important identifier of the hard disk and is usually used to uniquely identify the hard disk and identify the hardware. In some cases, we may need to query the hard drive serial number, such as when installing an operating system, finding the correct device driver, or performing hard drive repairs. This article will introduce some simple methods to help you check the hard drive serial number. Method 1: Use Windows Command Prompt to open the command prompt. In Windows system, press Win+R keys, enter "cmd" and press Enter key to open the command
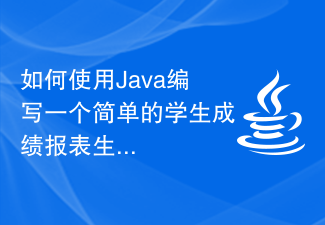
How to write a simple student performance report generator using Java? Student Performance Report Generator is a tool that helps teachers or educators quickly generate student performance reports. This article will introduce how to use Java to write a simple student performance report generator. First, we need to define the student object and student grade object. The student object contains basic information such as the student's name and student number, while the student score object contains information such as the student's subject scores and average grade. The following is the definition of a simple student object: public
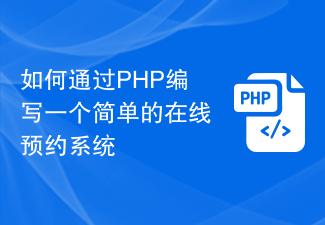
How to write a simple online reservation system through PHP. With the popularity of the Internet and users' pursuit of convenience, online reservation systems are becoming more and more popular. Whether it is a restaurant, hospital, beauty salon or other service industry, a simple online reservation system can improve efficiency and provide users with a better service experience. This article will introduce how to use PHP to write a simple online reservation system and provide specific code examples. Create database and tables First, we need to create a database to store reservation information. In MyS
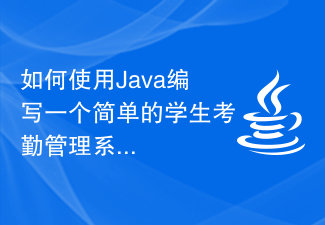
How to write a simple student attendance management system using Java? With the continuous development of technology, school management systems are also constantly updated and upgraded. The student attendance management system is an important part of it. It can help the school track students' attendance and provide data analysis and reports. This article will introduce how to write a simple student attendance management system using Java. 1. Requirements Analysis Before starting to write, we need to determine the functions and requirements of the system. Basic functions include registration and management of student information, recording of student attendance data and
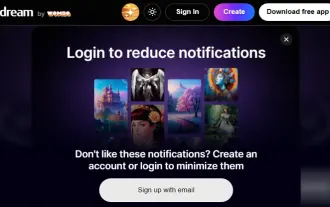
If you are eager to find the top free AI animation art generator, you can end your search. The world of anime art has been captivating audiences for decades with its unique character designs, captivating colors and captivating plots. However, creating anime art requires talent, skill, and a lot of time. However, with the continuous development of artificial intelligence (AI), you can now explore the world of animation art without having to delve into complex technologies with the help of the best free AI animation art generator. This will open up new possibilities for you to unleash your creativity. What is an AI anime art generator? The AI Animation Art Generator utilizes sophisticated algorithms and machine learning techniques to analyze an extensive database of animation works. Through these algorithms, the system learns and identifies different animation styles
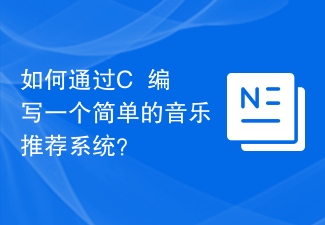
How to write a simple music recommendation system in C++? Introduction: Music recommendation system is a research hotspot in modern information technology. It can recommend songs to users based on their music preferences and behavioral habits. This article will introduce how to use C++ to write a simple music recommendation system. 1. Collect user data First, we need to collect user music preference data. Users' preferences for different types of music can be obtained through online surveys, questionnaires, etc. Save data in a text file or database
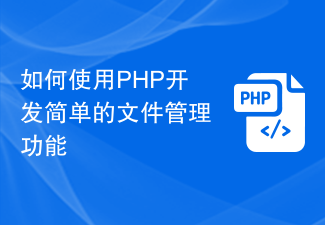
Introduction to how to use PHP to develop simple file management functions: File management functions are an essential part of many web applications. It allows users to upload, download, delete and display files, providing users with a convenient way to manage files. This article will introduce how to use PHP to develop a simple file management function and provide specific code examples. 1. Create a project First, we need to create a basic PHP project. Create the following file in the project directory: index.php: main page, used to display the upload table
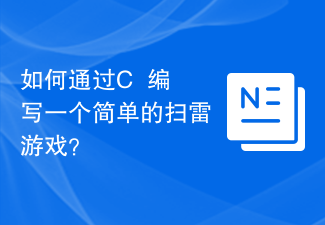
How to write a simple minesweeper game in C++? Minesweeper is a classic puzzle game that requires players to reveal all the blocks according to the known layout of the minefield without stepping on the mines. In this article, we will introduce how to write a simple minesweeper game using C++. First, we need to define a two-dimensional array to represent the map of the Minesweeper game. Each element in the array can be a structure used to store the status of the block, such as whether it is revealed, whether there are mines, etc. In addition, we also need to define
