How to use middleware to limit request flow in Laravel
How to use middleware to limit request flow in Laravel
Introduction:
When we develop web applications, we often encounter the need to limit user requests. Situations where requests are restricted, for example, limiting a certain number of requests to be sent per minute, or limiting the number of concurrent requests for a certain interface, etc. In the Laravel framework, we can implement request flow limiting through middleware. This article will introduce how to use middleware to limit request flow and provide corresponding code examples.
1. Understanding middleware and request flow limiting
Middleware (Middleware) is a mechanism provided by Laravel. It can intervene at various stages of request processing and process, filter or enhance requests. . Request throttling is a mechanism that limits the frequency or number of user requests. It is usually used to control the access speed of resources and protect the stability of the server.
2. Create throttling middleware
In Laravel, you can create a middleware through the Artisan command: php artisan make:middleware ThrottleRequests
The generated middleware file is located in the app/Http/Middleware
directory, for example: ThrottleRequests.php
.
Next, we need to implement the request flow limiting logic in the handle
method of the middleware, such as the following code:
<?php namespace AppHttpMiddleware; use Closure; use IlluminateCacheRateLimiter; use SymfonyComponentHttpFoundationResponse; class ThrottleRequests { protected $limiter; public function __construct(RateLimiter $limiter) { $this->limiter = $limiter; } public function handle($request, Closure $next, $maxAttempts = 60, $decayMinutes = 1) { $key = $request->ip(); // 使用 IP 地址作为限流的关键字 if ($this->limiter->tooManyAttempts($key, $maxAttempts)) { return new Response('Too Many Attempts.', 429); } $this->limiter->hit($key, $decayMinutes * 60); return $next($request); } }
In the above code, we use Laravel The RateLimiter
class built into the framework implements the request flow limiting function. The TooManyAttempts
method is used to determine whether the request exceeds the maximum allowed number, and if so, a 429 status code is returned; the hit
method is used to record the number of requests and set the length of the time window.
3. Register middleware
To make the middleware we create effective, we need the $middleware
array in the app/Http/Kernel.php
file Register middleware in . Find the file and add the following code:
protected $routeMiddleware = [ // ... 'throttle' => AppHttpMiddlewareThrottleRequests::class, ];
4. Use middleware for request current limiting
Through the above steps, we have successfully created a request current limiting middleware and completed the middleware register. Next, we can use the middleware in route definitions or controllers.
Use middleware in route definition:
Route::middleware('throttle:10,1')->get('/test', function () { return 'Hello, Laravel!'; });
Copy after loginIn the above code, we apply
throttle
middleware to/test
Routing allows up to 10 requests per minute, and after reaching the maximum number of requests, the user will receive a 429 status code.Using middleware in the controller:
class TestController extends Controller { public function __construct() { $this->middleware('throttle:10,1'); } public function index() { return 'Hello, Laravel!'; } }
Copy after loginWith the above code, we apply the
throttle
middleware toTestController
Theindex
method in the controller.Summary:
This article introduces how to use middleware in Laravel to limit request flow, and provides corresponding code examples. By using current-limiting middleware, we can flexibly control the frequency and number of user requests, thereby protecting the stability and security of the server. In actual web development, request throttling is a very important technology. I hope this article can be helpful to everyone. FinishThe above is the detailed content of How to use middleware to limit request flow in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


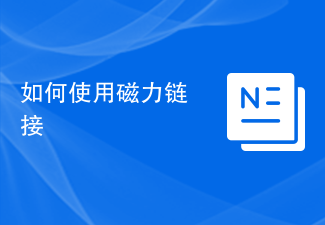
Magnet link is a link method for downloading resources, which is more convenient and efficient than traditional download methods. Magnet links allow you to download resources in a peer-to-peer manner without relying on an intermediary server. This article will introduce how to use magnet links and what to pay attention to. 1. What is a magnet link? A magnet link is a download method based on the P2P (Peer-to-Peer) protocol. Through magnet links, users can directly connect to the publisher of the resource to complete resource sharing and downloading. Compared with traditional downloading methods, magnetic
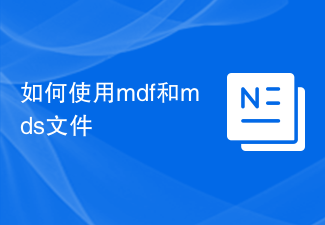
How to use mdf files and mds files With the continuous advancement of computer technology, we can store and share data in a variety of ways. In the field of digital media, we often encounter some special file formats. In this article, we will discuss a common file format - mdf and mds files, and introduce how to use them. First, we need to understand the meaning of mdf files and mds files. mdf is the extension of the CD/DVD image file, and the mds file is the metadata file of the mdf file.
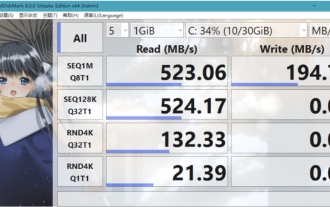
CrystalDiskMark is a small HDD benchmark tool for hard drives that quickly measures sequential and random read/write speeds. Next, let the editor introduce CrystalDiskMark to you and how to use crystaldiskmark~ 1. Introduction to CrystalDiskMark CrystalDiskMark is a widely used disk performance testing tool used to evaluate the read and write speed and performance of mechanical hard drives and solid-state drives (SSD). Random I/O performance. It is a free Windows application and provides a user-friendly interface and various test modes to evaluate different aspects of hard drive performance and is widely used in hardware reviews
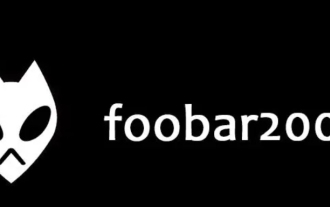
foobar2000 is a software that can listen to music resources at any time. It brings you all kinds of music with lossless sound quality. The enhanced version of the music player allows you to get a more comprehensive and comfortable music experience. Its design concept is to play the advanced audio on the computer The device is transplanted to mobile phones to provide a more convenient and efficient music playback experience. The interface design is simple, clear and easy to use. It adopts a minimalist design style without too many decorations and cumbersome operations to get started quickly. It also supports a variety of skins and Theme, personalize settings according to your own preferences, and create an exclusive music player that supports the playback of multiple audio formats. It also supports the audio gain function to adjust the volume according to your own hearing conditions to avoid hearing damage caused by excessive volume. Next, let me help you
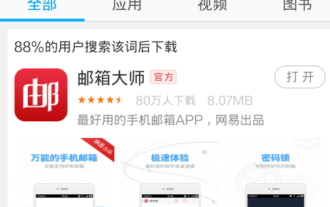
NetEase Mailbox, as an email address widely used by Chinese netizens, has always won the trust of users with its stable and efficient services. NetEase Mailbox Master is an email software specially created for mobile phone users. It greatly simplifies the process of sending and receiving emails and makes our email processing more convenient. So how to use NetEase Mailbox Master, and what specific functions it has. Below, the editor of this site will give you a detailed introduction, hoping to help you! First, you can search and download the NetEase Mailbox Master app in the mobile app store. Search for "NetEase Mailbox Master" in App Store or Baidu Mobile Assistant, and then follow the prompts to install it. After the download and installation is completed, we open the NetEase email account and log in. The login interface is as shown below
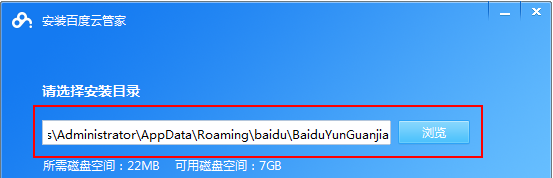
Cloud storage has become an indispensable part of our daily life and work nowadays. As one of the leading cloud storage services in China, Baidu Netdisk has won the favor of a large number of users with its powerful storage functions, efficient transmission speed and convenient operation experience. And whether you want to back up important files, share information, watch videos online, or listen to music, Baidu Cloud Disk can meet your needs. However, many users may not understand the specific use method of Baidu Netdisk app, so this tutorial will introduce in detail how to use Baidu Netdisk app. Users who are still confused can follow this article to learn more. ! How to use Baidu Cloud Network Disk: 1. Installation First, when downloading and installing Baidu Cloud software, please select the custom installation option.
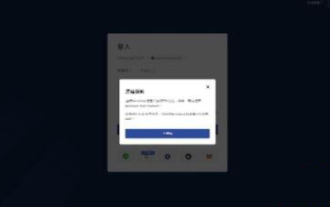
MetaMask (also called Little Fox Wallet in Chinese) is a free and well-received encryption wallet software. Currently, BTCC supports binding to the MetaMask wallet. After binding, you can use the MetaMask wallet to quickly log in, store value, buy coins, etc., and you can also get 20 USDT trial bonus for the first time binding. In the BTCCMetaMask wallet tutorial, we will introduce in detail how to register and use MetaMask, and how to bind and use the Little Fox wallet in BTCC. What is MetaMask wallet? With over 30 million users, MetaMask Little Fox Wallet is one of the most popular cryptocurrency wallets today. It is free to use and can be installed on the network as an extension
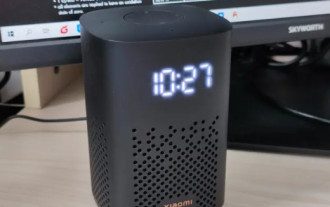
After long pressing the play button of the speaker, connect to wifi in the software and you can use it. Tutorial Applicable Model: Xiaomi 12 System: EMUI11.0 Version: Xiaoai Classmate 2.4.21 Analysis 1 First find the play button of the speaker, and press and hold to enter the network distribution mode. 2 Log in to your Xiaomi account in the Xiaoai Speaker software on your phone and click to add a new Xiaoai Speaker. 3. After entering the name and password of the wifi, you can call Xiao Ai to use it. Supplement: What functions does Xiaoai Speaker have? 1 Xiaoai Speaker has system functions, social functions, entertainment functions, knowledge functions, life functions, smart home, and training plans. Summary/Notes: The Xiao Ai App must be installed on your mobile phone in advance for easy connection and use.
