


In-depth understanding of the flag.Usage function custom command line help information in the Go language documentation
In-depth understanding of the flag.Usage function custom command line help information in the Go language document
In the Go language, we often use the flag package to process the command line parameter. The flag package provides a convenient way to parse and process command line parameters, allowing our program to accept different options and parameters entered by the user. In the flag package, there is a very important function - flag.Usage, which can help us customize the command line help information.
flag.Usage function is defined in the standard library flag package. Its function signature is as follows:
func Usage()
flag.Usage function is used to print customized Command line help information and end the program. By default, the flag package provides a simple help message and prints the message when the user enters an incorrect parameter. But sometimes we want to customize the output of help information according to our own needs. In this case, we need to use the flag.Usage function.
Below we use a specific example to demonstrate the usage of the flag.Usage function. Suppose we write a program that can accept two parameters, one is the file path and the other is the directory of the output file. We want to customize the help information to tell the user how to use our program.
package main import ( "flag" "fmt" "os" ) func main() { flag.Usage = func() { fmt.Fprintf(os.Stderr, "Usage: %s [options] <filepath> <outputdir> ", os.Args[0]) flag.PrintDefaults() } // 定义命令行参数 filepath := flag.String("f", "", "Path to the file") outputdir := flag.String("o", "", "Path to the output directory") // 解析命令行参数 flag.Parse() // 检查参数是否符合要求 if *filepath == "" || *outputdir == "" { flag.Usage() // 输出帮助信息并结束程序 os.Exit(1) } // 程序的具体逻辑... fmt.Printf("File Path: %s ", *filepath) fmt.Printf("Output Directory: %s ", *outputdir) }
In the above example, we first use the flag.Usage function to customize the output help information. In the anonymous function, we use the fmt.Fprintf
function to format the customized help information and output it to the standard error stream. Then use the flag.PrintDefaults function to output the default values and descriptions of all parameters.
Before parsing the command line parameters, we performed a check. If the user did not provide the necessary parameters, the flag.Usage function was called to output help information, and the os.Exit function was used to end the program.
When running the above program, if the user does not provide the necessary parameters, the following output will be obtained:
Usage: ./program [options] <filepath> <outputdir> -f string Path to the file -o string Path to the output directory exit status 1
We can see that the help information is customized by us and contains the parameters. Default value and description. By using the flag.Usage function, we can flexibly customize the command line help information according to our own needs, allowing users to better understand and use our program.
To sum up, the flag.Usage function is a very useful function. When processing command line parameters, customized help information is very important. By using the flag.Usage function appropriately, we can improve the usability of command line tools and increase user experience. I hope this article can help you better understand and use the flag.Usage function in the flag package.
The above is the detailed content of In-depth understanding of the flag.Usage function custom command line help information in the Go language documentation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
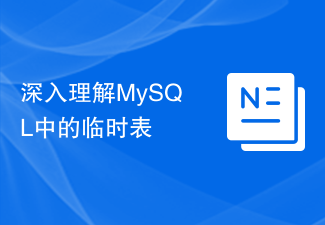
The temporary table in MySQL is a special table that can store some temporary data in the MySQL database. Temporary tables are different from ordinary tables in that they do not require users to manually create them in the database and only exist in the current connection and session. This article will take an in-depth look at temporary tables in MySQL. 1. What is a temporary table? A temporary table is a special type of table in MySQL that only exists in the current database session. Temporary tables do not require users to manually create them in the database in advance. Instead, they are created when the user performs SELECT, INSERT, or U
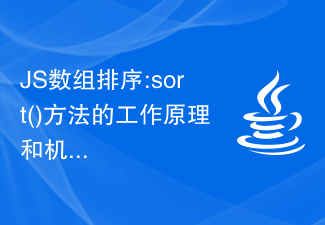
To deeply understand JS array sorting: the principles and mechanisms of the sort() method, specific code examples are required. Introduction: Array sorting is one of the very common operations in our daily front-end development work. The array sorting method sort() in JavaScript is one of the most commonly used array sorting methods. However, do you really understand the principles and mechanisms of the sort() method? This article will give you an in-depth understanding of the principles and mechanisms of JS array sorting, and provide specific code examples. 1. Basic usage of sort() method
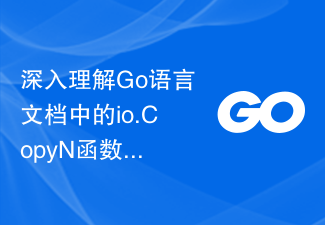
In-depth understanding of the io.CopyN function in the Go language documentation implements file copying with a limited number of bytes. The io package in the Go language provides many functions and methods for processing input and output streams. One of the very useful functions is io.CopyN, which can copy files with a limited number of bytes. This article will provide an in-depth understanding of this function and provide specific code examples. First, let's understand the basic definition of the io.CopyN function. It is defined as follows: funcCopyN(dstWriter,
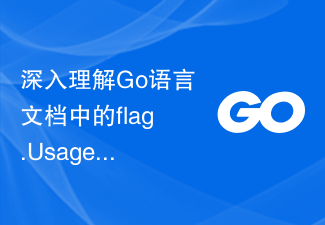
Deeply understand the custom command line help information of the flag.Usage function in the Go language documentation. In the Go language, we often use the flag package to process command line parameters. The flag package provides a convenient way to parse and process command line parameters, allowing our program to accept different options and parameters entered by the user. In the flag package, there is a very important function - flag.Usage, which can help us customize the command line help information. The flag.Usage function is in the standard library fl
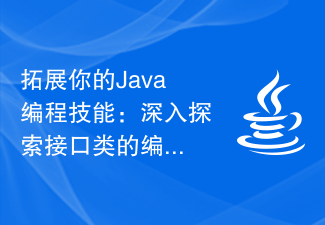
Improve your Java programming capabilities: In-depth understanding of how to write interface classes Introduction: In Java programming, interface is a very important concept. It can help us achieve program abstraction and modularization, making the code more flexible and extensible. In this article, we will delve into how to write interface classes and give specific code examples to help readers better understand and apply interfaces. 1. Definition and characteristics of interface In Java, interface is an abstract type. It is similar to a contract or contract, which defines the specifications of a set of methods without mentioning
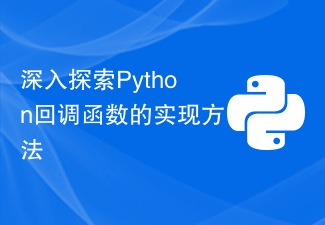
To deeply understand the implementation of Python callback functions, you need specific code examples. Preface: The callback function is a common programming concept that achieves code flexibility and scalability by passing another function as a parameter in the function. In Python, there are many ways to implement callback functions. This article will use specific code examples to help readers understand in depth. 1. Basic concepts A callback function refers to calling another function to process the result or respond to the event when a function is executed or an event is triggered. The callback function usually does
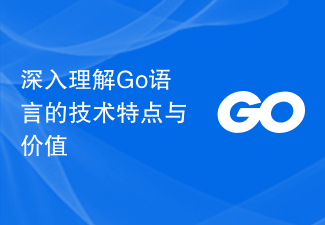
Go is a programming language developed by Google. It was first released in 2009 and has received widespread attention for its simplicity, efficiency, and ease of learning. The Go language is designed to handle applications with excellent concurrent performance, while also having fast compilation speed and concise coding style. This article will delve into the technical features and value of the Go language, and attach specific code examples to further illustrate. First, the concurrency model of Go language is very powerful. The Go language is provided through goroutines and channels
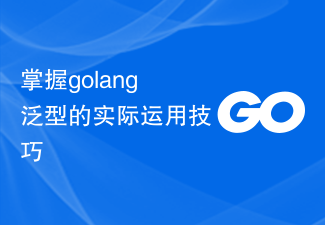
In-depth understanding of the use of golang generics requires specific code examples. Introduction: Among many programming languages, generics are a powerful programming tool that can realize type parameterization and improve code reusability and flexibility. . However, due to historical reasons, the Go language has not added direct support for generics, which makes many developers confused about implementing generic functions. This article will discuss some implementation methods of generics in golang and provide specific code examples to help readers understand golan in depth.
