


Use JavaScript functions to implement image carousels and slideshow effects
JavaScript is a scripting language that can be used to add interactive effects to web pages. Among them, image carousel and slideshow effects are common web page animation effects. This article will introduce how to use JavaScript functions to achieve these two effects and provide specific code examples.
- Picture carousel
Picture carousel is an effect that plays multiple pictures in turn in a certain way. When implementing image carousels, JavaScript timers and CSS style controls need to be used.
(1) Preparation
First, in the HTML file, you need to define a div container to display the carousel image. We can define another ul element to store all the images that need to be rotated. Each li element contains an image.
<div id="slider"> <ul> <li><img src="/static/imghw/default1.png" data-src="img1.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" ></li> <li><img src="/static/imghw/default1.png" data-src="img2.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" ></li> <li><img src="/static/imghw/default1.png" data-src="img3.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" ></li> <li><img src="/static/imghw/default1.png" data-src="img4.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" ></li> </ul> </div>
In the CSS file, some styling needs to be done on these elements. For example, set the width and height of the div container to the actual size of the image, and set the overflow attribute to hidden, so that the portion beyond the container can be hidden. At the same time, set the width of the ul element to the sum of the widths of all images, and set the height to the actual height of the image.
#slider { width: 600px; height: 400px; overflow: hidden; position: relative; } #slider ul { width: 2400px; /* 4张图片的宽度之和 */ height: 400px; position: absolute; left: 0; }
(2) Carousel implementation
Next, we need to use JavaScript functions to achieve the carousel effect. The specific implementation process is as follows:
① Define a variable index to record the serial number of the currently displayed picture.
var index = 0;
② Write a carousel function to switch pictures at certain intervals and update the value of the index variable. In this function, the left value of the ul element needs to be set to the opposite number (negative number) of the width of the current image, so that the carousel effect can be achieved.
function slide() { index++; if (index >= 4) { // 图片总数为4,如果index超过4,就将其重置为0 index = 0; } var leftVal = -index * 600 + "px"; // 每次切换,将ul元素的left值设置为当前图片的宽度的相反数 $("#slider ul").stop().animate({left: leftVal}, 500); // 使用jQuery的animate方法实现滑动效果 }
In the above code, we use the animate() method in the jQuery library, which can be used to achieve animation effects. The animate() method accepts two parameters. The first parameter is an object used to set the CSS properties and values of the animation. Here we set the left attribute of the ul element; the second parameter is a number used to specify the animation run. time, in milliseconds.
③ Call the carousel function and use the setInterval() method to execute it regularly.
setInterval(slide, 2000);
In the above code, we use the setInterval() method, which can be used to execute specified code regularly. The first parameter is the name of the function to be executed regularly, and the second parameter is the time interval in milliseconds.
Finally, the implementation code of the entire picture carousel is as follows:
<!DOCTYPE html> <html> <head> <title>图片轮播</title> <meta charset="utf-8"> <style> #slider { width: 600px; height: 400px; overflow: hidden; position: relative; } #slider ul { width: 2400px; height: 400px; position: absolute; left: 0; } #slider ul li { float: left; } </style> <script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <script> $(function () { var index = 0; function slide() { index++; if (index >= 4) { index = 0; } var leftVal = -index * 600 + "px"; $("#slider ul").stop().animate({left: leftVal}, 500); } setInterval(slide, 2000); }) </script> </head> <body> <div id="slider"> <ul> <li><img src="/static/imghw/default1.png" data-src="img1.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" ></li> <li><img src="/static/imghw/default1.png" data-src="img2.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" ></li> <li><img src="/static/imghw/default1.png" data-src="img3.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" ></li> <li><img src="/static/imghw/default1.png" data-src="img4.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" ></li> </ul> </div> </body> </html>
- Slideshow effect
The slideshow effect is a way to combine multiple pictures The effect of switching pictures in a certain order. When implementing slide effects, JavaScript event listening and CSS style control are required.
(1) Preparation
Similarly, in the HTML file, a div container needs to be defined to display the slide. We can define multiple img elements, each img element contains a picture.
<div id="slideshow"> <img src="/static/imghw/default1.png" data-src="img1.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" > <img src="/static/imghw/default1.png" data-src="img2.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" > <img src="/static/imghw/default1.png" data-src="img3.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" > <img src="/static/imghw/default1.png" data-src="img4.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" > </div>
In the CSS file, we need to style these elements. For example, set the width and height of the div container to the actual size of the image, and set the overflow attribute to hidden; set the position of all img elements to absolute so that they can overlap; and set all but the first image The image's transparency is set to 0.
#slideshow { width: 600px; height: 400px; overflow: hidden; position: relative; } #slideshow img { position: absolute; top: 0; left: 0; opacity: 0; } #slideshow img:first-child { opacity: 1; }
In the above code, we use the :first-child pseudo-class to set the transparency of the first image to 1.
(2) Slideshow implementation
Next, we need to use JavaScript functions to achieve the slideshow effect. The specific process is as follows:
① Define a variable index to record the serial number of the currently displayed picture.
var index = 1;
② Write a function to switch pictures and update the value of the index variable. In this function, we first set the transparency of the currently displayed image to 0, then add 1 to the value of the index variable, and determine whether the total number of images is exceeded. If exceeded, reset it to 1. Then set the transparency of the next image to 1 and animate it.
function show() { $("#slideshow img:nth-child(" + index + ")").stop().animate({opacity: 0}, 1000); // 当前图片透明度减少 index++; if (index > 4) { index = 1; } $("#slideshow img:nth-child(" + index + ")").stop().animate({opacity: 1}, 1000); // 下一张图片透明度增加 }
In the above code, we use the :nth-child selector, which can select a child element under the specified parent element. In this example, we use this selector to select the index image.
③ Use the setInterval() method to execute the show function regularly.
$(function () { setInterval(show, 3000); })
In the above code, we use the $() method and setInterval() method of the jQuery library to implement scheduled calls. The $() method is used to obtain the specified element, and the setInterval() method can call the specified function periodically.
Finally, the implementation code of the entire slide effect is as follows:
<!DOCTYPE html> <html> <head> <title>幻灯片效果</title> <meta charset="utf-8"> <style> #slideshow { width: 600px; height: 400px; overflow: hidden; position: relative; } #slideshow img { position: absolute; top: 0; left: 0; opacity: 0; } #slideshow img:first-child { opacity: 1; } </style> <script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <script> $(function () { var index = 1; function show() { $("#slideshow img:nth-child(" + index + ")").stop().animate({opacity: 0}, 1000); index++; if (index > 4) { index = 1; } $("#slideshow img:nth-child(" + index + ")").stop().animate({opacity: 1}, 1000); } setInterval(show, 3000); }) </script> </head> <body> <div id="slideshow"> <img src="/static/imghw/default1.png" data-src="img1.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" > <img src="/static/imghw/default1.png" data-src="img2.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" > <img src="/static/imghw/default1.png" data-src="img3.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" > <img src="/static/imghw/default1.png" data-src="img4.jpg" class="lazy" alt="Use JavaScript functions to implement image carousels and slideshow effects" > </div> </body> </html>
Through the above code examples, we have implemented the use of JavaScript functions to achieve image carousel and slide effects, and introduced specific implementation process. These technologies are very helpful for improving web page interaction and animation effects. Readers can modify and optimize according to actual needs and continuously improve their development capabilities.
The above is the detailed content of Use JavaScript functions to implement image carousels and slideshow effects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
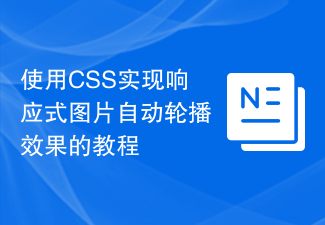
With the popularity of mobile devices, web design needs to take into account factors such as device resolution and screen size of different terminals to achieve a good user experience. When implementing responsive design of a website, it is often necessary to use the image carousel effect to display the content of multiple images in a limited visual window, and at the same time, it can also enhance the visual effect of the website. This article will introduce how to use CSS to achieve a responsive image automatic carousel effect, and provide code examples and analysis. Implementation ideas The implementation of responsive image carousel can be implemented through CSS flex layout. exist
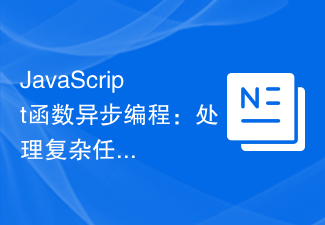
JavaScript Function Asynchronous Programming: Essential Skills for Handling Complex Tasks Introduction: In modern front-end development, handling complex tasks has become an indispensable part. JavaScript function asynchronous programming skills are the key to solving these complex tasks. This article will introduce the basic concepts and common practical methods of JavaScript function asynchronous programming, and provide specific code examples to help readers better understand and use these techniques. 1. Basic concepts of asynchronous programming In traditional synchronous programming, the code is
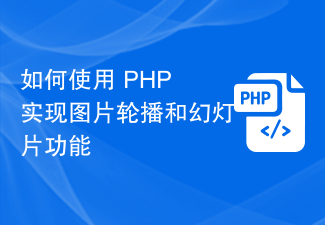
How to use PHP to implement image carousel and slideshow functions In modern web design, image carousel and slideshow functions have become very popular. These features can add some dynamics and appeal to web pages and improve user experience. This article will introduce how to use PHP to implement image carousel and slideshow functions to help readers master this technology. Creating the Infrastructure in HTML First, create the infrastructure in the HTML file. Let's say our image carousel has a container and several image elements. The HTML code is as follows
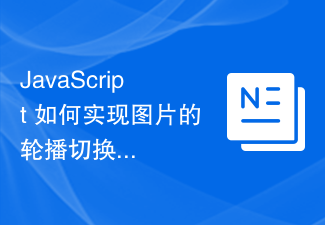
How to implement image carousel switching effect and add fade-in and fade-out animation with JavaScript? Image carousel is one of the common effects in web design. By switching images to display different content, it gives users a better visual experience. In this article, I will introduce how to use JavaScript to achieve a carousel switching effect of images and add a fade-in and fade-out animation effect. Below is a specific code example. First, we need to create a container containing the carousel in the HTML page and add it
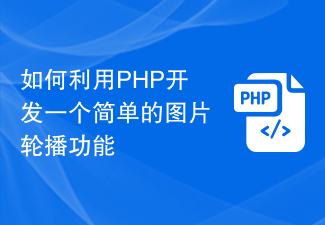
How to use PHP to develop a simple image carousel function. The image carousel function is very common in web design, and can present users with better visual effects and improve user experience. This article will introduce how to use PHP to develop a simple image carousel function and give specific code examples. First, we need to prepare some image resources as carousel images. Place these images in a folder and name it "slider", making sure the folder path is correct. Next, we need to write a PHP script to get these graphs
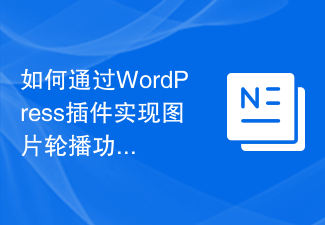
How to implement image carousel function through WordPress plug-in In today’s website design, image carousel function has become a common requirement. It can make the website more attractive and can display multiple pictures to achieve better publicity effect. In WordPress, we can implement the image carousel function by installing plug-ins. This article will introduce a common plug-in and provide code samples for reference. 1. Plug-in introduction In the WordPress plug-in library, there are many image carousel plug-ins to choose from, one of which is often
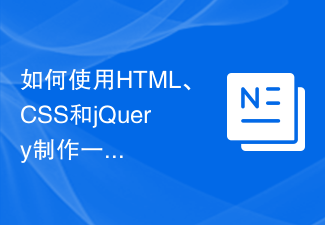
How to use HTML, CSS and jQuery to create a dynamic image carousel. In website design and development, image carousel is a frequently used function for displaying multiple images or advertising banners. Through the combination of HTML, CSS and jQuery, we can achieve a dynamic image carousel effect, adding vitality and appeal to the website. This article will introduce how to use HTML, CSS and jQuery to create a simple dynamic image carousel, and provide specific code examples. Step 1: Set up HTML junction
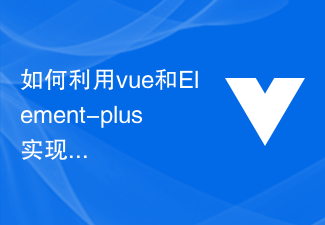
How to use Vue and ElementPlus to implement image carousels and slideshows. In web design, image carousels and slideshows are common functional requirements. These functions can be easily implemented using Vue and ElementPlus framework. This article will introduce how to use Vue and ElementPlus to create a simple and beautiful picture carousel and slideshow component. First, we need to install Vue and ElementPlus. Execute the following commands on the command line:
