


Use JavaScript functions to implement user login and permission verification
Use JavaScript functions to implement user login and permission verification
With the development of the Internet, user login and permission verification have become essential functions for many websites and applications. In order to protect users' data security and access rights, we need to use some technologies and methods to verify the user's identity and restrict their access rights.
As a widely used scripting language, JavaScript plays an important role in front-end development. We can use JavaScript functions to implement user login and permission verification functions. The following will introduce in detail how to implement this function through JavaScript functions and provide some code examples.
First, we need to create a user login function. This function will accept the username and password entered by the user and verify their validity. The following is a sample code:
function login(username, password) { // 校验用户名和密码的逻辑 if (username === 'admin' && password === 'admin123') { return true; } else { return false; } }
In the above code, we determine whether the user login is successful by comparing the entered user name and password with the preset values. In actual development, we need to interact with the database or server to verify the correctness of the user name and password.
Next, we need to create a permission verification function. This function will determine whether the user has permission to perform an operation. The following is a sample code:
function checkPermission(user, operation) { // 获取用户权限的逻辑 if (user.role === 'admin' || (user.role === 'editor' && operation === 'edit')) { return true; } else { return false; } }
In the above code, we determine whether the user has permission to perform an operation by comparing the user's role and operation type. In actual development, we need to write logic according to specific business requirements and permission design.
Finally, we can combine the user login and permission verification functions to achieve complete login and permission verification functions. The following is a sample code:
function login(username, password) { // 校验用户名和密码的逻辑 if (username === 'admin' && password === 'admin123') { return true; } else { return false; } } function checkPermission(user, operation) { // 获取用户权限的逻辑 if (user.role === 'admin' || (user.role === 'editor' && operation === 'edit')) { return true; } else { return false; } } function loginUser(username, password, operation) { if (login(username, password)) { // 登录成功,进行权限验证 const user = { username: username, role: 'admin' }; // 用户信息可以从数据库或服务器获取 if (checkPermission(user, operation)) { console.log('用户登录成功且有权限进行操作!'); } else { console.log('用户登录成功但没有权限进行操作!'); } } else { console.log('用户登录失败!'); } } // 示例调用代码 loginUser('admin', 'admin123', 'edit');
In the above code, we combine the login and permission verification functions and implement login and permission verification by calling the loginUser
function. Depending on the actual situation, we can store user information in a database or server, and obtain and verify it when logging in.
Through the above sample code, we can see how to use JavaScript functions to implement user login and permission verification functions. Of course, actual development may involve more complex logic and processes, but by using JavaScript functions, we can flexibly implement various needs.
I hope this article is helpful to you, thank you for reading!
The above is the detailed content of Use JavaScript functions to implement user login and permission verification. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


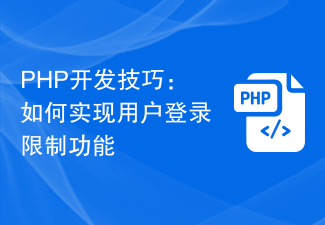
PHP development skills: How to implement user login restriction function In website or application development, user login restriction function is a very important security measure. By limiting the number of login attempts and frequency of users, you can effectively prevent accounts from being maliciously cracked or brute force cracked. This article will introduce how to use PHP to implement user login restriction function and provide specific code examples. 1. Requirements analysis of user login restriction function User login restriction function usually includes the following requirements: Limitation on the number of login attempts: when the user continuously inputs errors
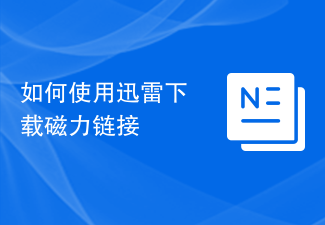
With the rapid development of network technology, our lives have also been greatly facilitated, one of which is the ability to download and share various resources through the network. In the process of downloading resources, magnet links have become a very common and convenient download method. So, how to use Thunder magnet links? Below, I will give you a detailed introduction. Xunlei is a very popular download tool that supports a variety of download methods, including magnet links. A magnet link can be understood as a download address through which we can obtain relevant information about resources.
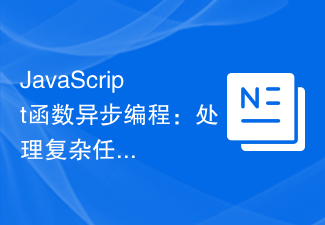
JavaScript Function Asynchronous Programming: Essential Skills for Handling Complex Tasks Introduction: In modern front-end development, handling complex tasks has become an indispensable part. JavaScript function asynchronous programming skills are the key to solving these complex tasks. This article will introduce the basic concepts and common practical methods of JavaScript function asynchronous programming, and provide specific code examples to help readers better understand and use these techniques. 1. Basic concepts of asynchronous programming In traditional synchronous programming, the code is
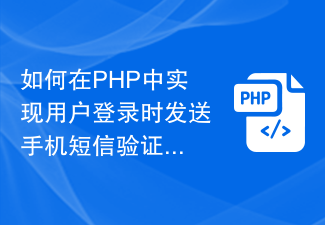
How to send SMS verification codes and email notifications when users log in in PHP. With the rapid development of the Internet, more and more applications require user login functions to ensure security and personalized experience. In addition to basic account and password verification, in order to improve user experience and security, many applications will also send mobile phone SMS verification codes and email notifications when users log in. This article will describe how to implement this functionality in PHP and provide corresponding code examples. 1. Send SMS verification code 1. First, you need someone who can send SMS
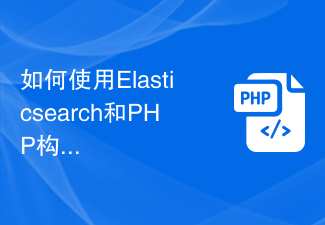
How to use Elasticsearch and PHP to build a user login and permission management system Introduction: In the current Internet era, user login and permission management are one of the necessary functions for every website or application. Elasticsearch is a powerful and flexible full-text search engine, while PHP is a widely used server-side scripting language. This article will introduce how to combine Elasticsearch and PHP to build a simple user login and permission management system

UniApp implements detailed analysis of user login and authorization. In modern mobile application development, user login and authorization are essential functions. As a cross-platform development framework, UniApp provides a convenient way to implement user login and authorization. This article will explore the details of user login and authorization in UniApp, and attach corresponding code examples. 1. Implementation of user login function Create login page User login function usually requires a login page, which contains a form for users to enter their account number and password and a login button
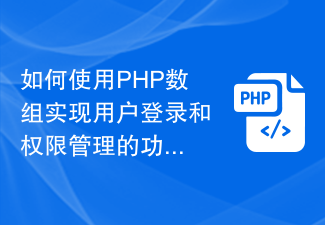
How to use PHP arrays to implement user login and permission management functions When developing a website, user login and permission management are one of the very important functions. User login allows us to authenticate users and protect the security of the website. Permission management can control users' operating permissions on the website to ensure that users can only access the functions for which they are authorized. In this article, we will introduce how to use PHP arrays to implement user login and permission management functions. We'll use a simple example to demonstrate this process. First we need to create
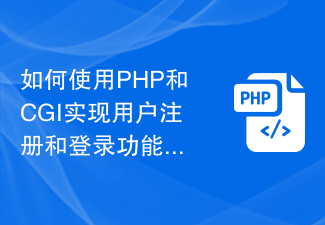
How to use PHP and CGI to implement user registration and login functions User registration and login are one of the necessary functions for many websites. In this article, we will introduce how to use PHP and CGI to achieve these two functions. We'll demonstrate the entire process with a code example. 1. Implementation of the user registration function The user registration function allows new users to create an account and save their information to the database. The following is a code example to implement the user registration function: Create a database table First, we need to create a database table to store user information. Can
