How to implement role-based dynamic permission switching in Laravel
Laravel is a popular web application development framework based on the PHP language. It has many excellent features that allow programmers to quickly build high-quality web applications. This includes the use of middleware to implement important functions such as authentication and permission control. In this article, we will explore how to implement role-based dynamic permission switching in Laravel, while providing specific code examples.
What is role-based dynamic permission switching?
Role-based dynamic permission switching is a common permission control mode. In this model, user roles in the system represent a set of operation permissions. After logging in, users will be assigned corresponding permissions based on their roles. During the user's use of the system, the system administrator can change the user's role in the background, thereby changing the user's operating permissions.
Implementing role-based dynamic permission switching in Laravel
Laravel provides many excellent functions to implement role-based dynamic permission switching. Below, we will implement a simple example step by step. Through this example, you can better understand how to implement role-based dynamic permission switching in Laravel.
Step 1: Create database and user table
First, let us create a database and a user table. User table includes ID, username, email, password, role ID, creation time and update time.
Step 2: Define the user model
Next, we need to define the corresponding user model. In Laravel, you can use the Artisan command to create a model:
php artisan make:model User
Then, we can define a belongsTo() method that associates the role model in the generated User model. The code example is as follows:
class User extends Model { public function role() { return $this->belongsTo('AppRole'); } }
Step 3: Create a role table and role model
Create a role table and a role model. The role table includes two fields: ID and role name.
Step 4: Define the relationship between role models
In the role model, we can define the permissions this role has. You can use the belongsToMany() method, which can establish a many-to-many relationship between roles and permissions. The code example is as follows:
class Role extends Model { public function permissions() { return $this->belongsToMany('AppPermission', 'permission_role'); } }
Step 5: Create a permission table and permission model
Create a permission table and a permission model. The permission table includes two fields: ID and permission name.
Step 6: Define the relationship between permission models
In the permission model, we can define the relationship between roles and permissions. You can use the belongsToMany() method, which can establish a many-to-many relationship between roles and permissions. The sample code is as follows:
class Permission extends Model { public function roles() { return $this->belongsToMany('AppRole', 'permission_role'); } }
Step 7: Define middleware
In Laravel, middleware is used to handle requests and responses, including authentication and authorization. We can define a middleware to check if the user has permission to perform the requested operation. The code example is as follows:
namespace AppHttpMiddleware; use Closure; use IlluminateSupportFacadesAuth; class CheckPermission { public function handle($request, Closure $next,$permissions) { $sessionUser = Auth::user(); foreach($sessionUser->role()->get() as $role) { $role_permissions = $role->permissions()->pluck('name')->toArray(); foreach($permissions as $perm) { if (in_array($perm, $role_permissions)) { return $next($request); } } } return redirect('/login'); } }
In the above code, we first retrieve the user information from the session, and return the permissions of the corresponding role through the user's role. The $permissions variable contains the permissions that need to be controlled. If the user has permission, execution continues. Otherwise, redirect to the login page.
Step 8: Use middleware
We can define the use of middleware CheckPermission in routing to restrict users from using certain routes. The code example is as follows:
Route::get('admin/dashboard',['middleware'=>['permission'],'uses'=>'AdminController@dashboard']);
In the controller, we can check if the user has permission to use this route as follows:
class AdminController extends Controller { public function dashboard() { $this->middleware('check-permission:user-list'); return view('admin.index'); } }
After accessing the route in the browser, get it in the session The current user's roles and permissions. If the user has permission, execution continues.
Conclusion
In this article, we discussed how to implement role-based dynamic permission switching in Laravel. Implementing permission control through middleware is a very common way. When implementing, please ensure that your system has a complete role management system and that there is a many-to-many relationship between roles and permissions. Only in this way can very powerful authentication and authorization functions be implemented in Laravel.
The above is the detailed content of How to implement role-based dynamic permission switching in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


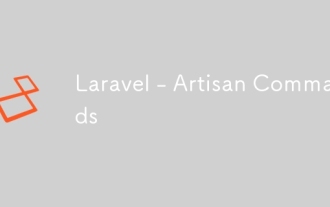
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?
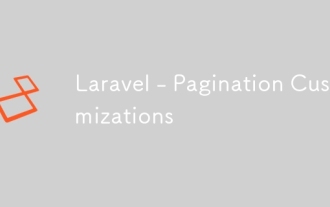
Laravel - Pagination Customizations - Laravel includes a feature of pagination which helps a user or a developer to include a pagination feature. Laravel paginator is integrated with the query builder and Eloquent ORM. The paginate method automatical
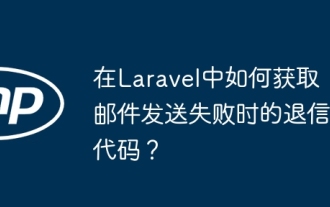
Method for obtaining the return code when Laravel email sending fails. When using Laravel to develop applications, you often encounter situations where you need to send verification codes. And in reality...
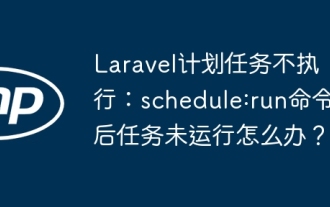
Laravel schedule task run unresponsive troubleshooting When using Laravel's schedule task scheduling, many developers will encounter this problem: schedule:run...
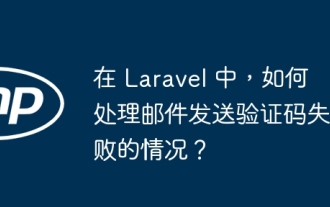
The method of handling Laravel's email failure to send verification code is to use Laravel...
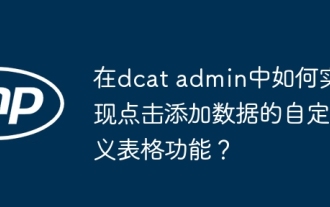
How to implement the table function of custom click to add data in dcatadmin (laravel-admin) When using dcat...
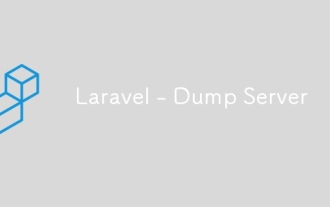
Laravel - Dump Server - Laravel dump server comes with the version of Laravel 5.7. The previous versions do not include any dump server. Dump server will be a development dependency in laravel/laravel composer file.
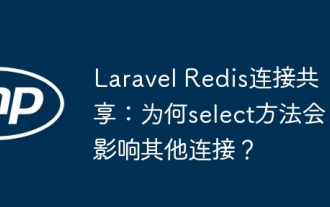
The impact of sharing of Redis connections in Laravel framework and select methods When using Laravel framework and Redis, developers may encounter a problem: through configuration...
