


Introduction to Python functions: usage and examples of hash functions
Introduction to Python functions: Usage and examples of hash function
- Introduction
In Python, the hash function is a built-in function that is used to generate The hash value of the object. A hash value is the result of an algorithm that maps a binary value of arbitrary length to a unique value of fixed length. The function of the hash function is to make the values well distributed and to be located quickly. In Python, the hash function can be applied to basic data types such as strings, integers, floating point numbers, etc., as well as hashable objects such as tuples and dictionaries. - Usage
The usage of the hash function is very simple. You only need to call hash and pass in the object to be hashed as a parameter. The function returns an integer hash value.
For example, we can use the hash function to hash the string:
string = "Hello World" hash_value = hash(string) print(hash_value)
In the above code, we use the hash function to hash the string "Hello World" , and assign the result to the hash_value variable. Finally, we output the hash value via the print function.
- Example
3.1 Hash string
string = "Hello World" hash_value = hash(string) print(hash_value)
Output: 2922927337147303222
In this example, we have the string " Hello World" performs a hash operation and prints out the hash value.
3.2 Hash integer
num = 12345 hash_value = hash(num) print(hash_value)
Output: 12345
In this example, we hash the integer 12345 and print out the hash value. Since an integer is an immutable object, its hash value is equal to itself.
3.3 Hash tuple
tuple_1 = (1, 2, 3) hash_value_1 = hash(tuple_1) tuple_2 = (4, 5, 6) hash_value_2 = hash(tuple_2) print(hash_value_1) print(hash_value_2)
Output:
- The hash value of tuple_1: 2528502973977326415
- The hash value of tuple_2: 2528502973977326683
In this example, we hash two tuples separately and print out their hash values.
3.4 Hash Dictionary
dict_1 = {"name": "Alice", "age": 18} hash_value_1 = hash(frozenset(dict_1.items())) dict_2 = {"name": "Bob", "age": 20} hash_value_2 = hash(frozenset(dict_2.items())) print(hash_value_1) print(hash_value_2)
Output:
- Hash value of dict_1: -4894169783345032514
- Hash value of dict_2: 2528502973977326681
In this example, we hash the two dictionaries separately and print out their hash values. Since the dictionary is a mutable object, we need to convert it to an immutable frozenset object before performing a hash operation.
Summary
- The hash function can be used to generate a hash value of an object.
- The hash function is a built-in function and can be used directly.
- The hash function is suitable for basic data types and hashable objects.
- The hash values of different objects are unique.
Through this article, we learned the usage and examples of hash function and understood its basic operation. In actual programming, reasonable use of hash functions can improve program performance and efficiency.
The above is the detailed content of Introduction to Python functions: usage and examples of hash functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


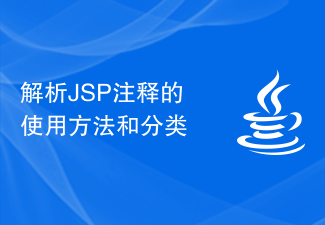
Classification and Usage Analysis of JSP Comments JSP comments are divided into two types: single-line comments: ending with, only a single line of code can be commented. Multi-line comments: starting with /* and ending with */, you can comment multiple lines of code. Single-line comment example Multi-line comment example/**This is a multi-line comment*Can comment on multiple lines of code*/Usage of JSP comments JSP comments can be used to comment JSP code to make it easier to read

The DECODE function in Oracle is a conditional expression that is often used to return different results based on different conditions in query statements. This article will introduce the syntax, usage and sample code of the DECODE function in detail. 1. DECODE function syntax DECODE(expr,search1,result1[,search2,result2,...,default]) expr: the expression or field to be compared. search1,
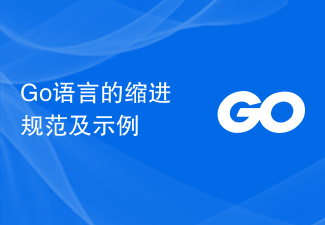
Indentation specifications and examples of Go language Go language is a programming language developed by Google. It is known for its concise and clear syntax, in which indentation specifications play a crucial role in the readability and beauty of the code. effect. This article will introduce the indentation specifications of the Go language and explain in detail through specific code examples. Indentation specifications In the Go language, tabs are used for indentation instead of spaces. Each level of indentation is one tab, usually set to a width of 4 spaces. Such specifications unify the coding style and enable teams to work together to compile
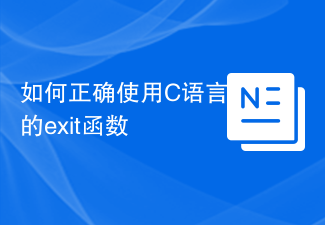
How to use the exit function in C language requires specific code examples. In C language, we often need to terminate the execution of the program early in the program, or exit the program under certain conditions. C language provides the exit() function to implement this function. This article will introduce the usage of exit() function and provide corresponding code examples. The exit() function is a standard library function in C language and is included in the header file. Its function is to terminate the execution of the program, and can take an integer
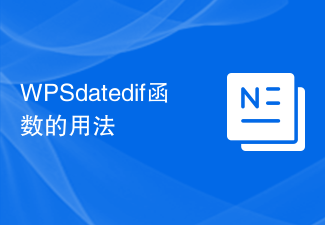
WPS is a commonly used office software suite, and the WPS table function is widely used for data processing and calculations. In the WPS table, there is a very useful function, the DATEDIF function, which is used to calculate the time difference between two dates. The DATEDIF function is the abbreviation of the English word DateDifference. Its syntax is as follows: DATEDIF(start_date,end_date,unit) where start_date represents the starting date.
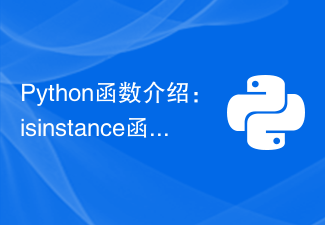
Introduction to Python functions: Usage and examples of the isinstance function Python is a powerful programming language that provides many built-in functions to make programming more convenient and efficient. One of the very useful built-in functions is the isinstance() function. This article will introduce the usage and examples of the isinstance function and provide specific code examples. The isinstance() function is used to determine whether an object is an instance of a specified class or type. The syntax of this function is as follows
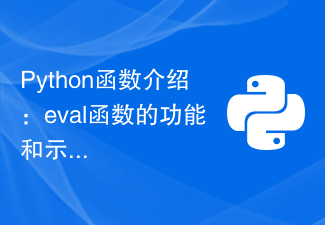
Introduction to Python functions: functions and examples of the eval function In Python programming, the eval function is a very useful function. The eval function can execute a string as program code, and its function is very powerful. In this article, we will introduce the detailed functions of the eval function, as well as some usage examples. 1. Function of eval function The function of eval function is very simple. It can execute a string as Python code. This means that we can convert a string
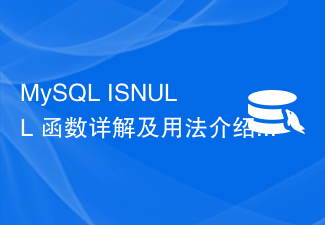
The ISNULL() function in MySQL is a function used to determine whether a specified expression or column is NULL. It returns a Boolean value, 1 if the expression is NULL, 0 otherwise. The ISNULL() function can be used in the SELECT statement or for conditional judgment in the WHERE clause. 1. The basic syntax of the ISNULL() function: ISNULL(expression) where expression is the expression to determine whether it is NULL or
