


Introduction to Python functions: functions and usage examples of globals functions
Introduction to Python functions: functions and usage examples of globals functions
Python is a powerful programming language that provides many built-in functions, among which the globals() function It's one of them. This article will introduce the functions and usage examples of the globals() function, with specific code examples.
1. Function of globals function
The globals() function is a built-in function used to return a dictionary of global variables of the current module. It returns a dictionary containing global variables, where the keys are the variable names and the values are the values of the variables.
Use the globals() function to access and operate global variables in the form of a dictionary. Within the function, if we want to obtain or modify global variables, we can use the globals() function to achieve this.
At the same time, the globals() function can also be used to inspect and debug code. By printing the returned dictionary, we can view all global variables of the current module and their corresponding values to better understand the execution process of the code.
2. Examples of using the globals function
The following are some specific code examples showing the usage scenarios and functions of the globals() function.
- Get global variables
Suppose we have a global variable x and want to access it inside a function. This can be achieved using the globals() function:
x = 10 def func(): global_x = globals()['x'] print(global_x) func() # 输出:10
In this example, we first define a global variable x and assign it a value of 10. Then, we define a function func(), use the globals() function inside the function to obtain the global variable x, and print it out. Run the func() function and the output result is 10.
- Modify global variables
In addition to obtaining the value of global variables, the globals() function can also be used to modify global variables. We can access global variables through dictionaries and modify their values through assignment.
x = 10 def modify_global(): globals()['x'] = 20 modify_global() print(x) # 输出:20
In this example, we define the function modify_global(), use the globals() function inside the function to obtain the global variable x, and assign it a value of 20. Then, we print the value of the global variable x, and the output is 20.
- Check global variables
In addition to using the globals() function to access and modify global variables inside the function, we can also use the globals() function to check all of the current module Global variables.
x = 10 y = 20 z = 30 def check_globals(): global_vars = globals() for var in global_vars: print(var, global_vars[var]) check_globals()
In this example, we define three global variables x, y, and z and assign them different values. Then, we defined the function check_globals(), used the globals() function inside the function to obtain the dictionary of global variables, and printed each global variable and its corresponding value through a for loop. Run the check_globals() function, the output result is as follows:
x 10 y 20 z 30
By printing the returned dictionary, we can clearly see all the global variables of the current module and their corresponding values.
Summary:
This article introduces the functions and usage examples of the globals() function. Through the globals() function, we can obtain, modify and check the global variables of the current module, which improves the flexibility and readability of the code. Using the globals() function can more conveniently access and operate global variables to adapt to different programming needs.
The above is the detailed content of Introduction to Python functions: functions and usage examples of globals functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


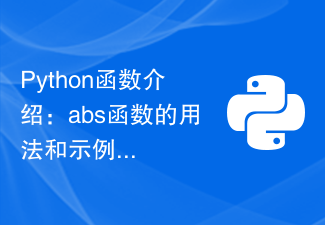
Introduction to Python functions: usage and examples of the abs function 1. Introduction to the usage of the abs function In Python, the abs function is a built-in function used to calculate the absolute value of a given value. It can accept a numeric argument and return the absolute value of that number. The basic syntax of the abs function is as follows: abs(x) where x is the numerical parameter to calculate the absolute value, which can be an integer or a floating point number. 2. Examples of abs function Below we will show the usage of abs function through some specific examples: Example 1: Calculation
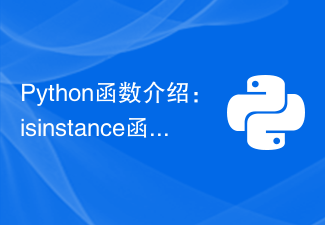
Introduction to Python functions: Usage and examples of the isinstance function Python is a powerful programming language that provides many built-in functions to make programming more convenient and efficient. One of the very useful built-in functions is the isinstance() function. This article will introduce the usage and examples of the isinstance function and provide specific code examples. The isinstance() function is used to determine whether an object is an instance of a specified class or type. The syntax of this function is as follows
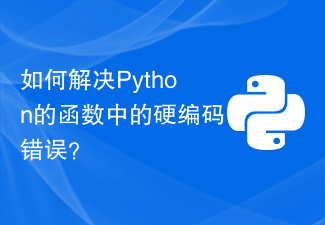
With the widespread use of the Python programming language, developers often encounter the problem of "hard-coded errors" in the process of writing programs. The so-called "hard coding error" refers to writing specific numerical values, strings and other data directly into the code instead of defining them as constants or variables. This approach has many problems, such as low readability, difficulty in maintaining, modifying and testing, and it also increases the possibility of errors. This article discusses how to solve the problem of hard-coded errors in Python functions. 1. What is hard
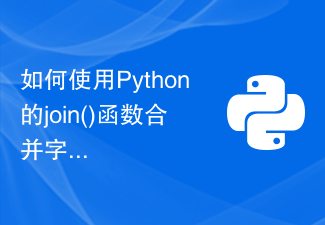
Overview of how to use Python's join() function to merge strings: In Python, we often encounter situations where we need to merge multiple strings. Python provides a very convenient method join() to merge strings. This article will introduce how to use the join() function and provide some specific code examples. How to use the join() function: The join() function is a method of string. It accepts an iterable object as a parameter and adds the elements in the object to the
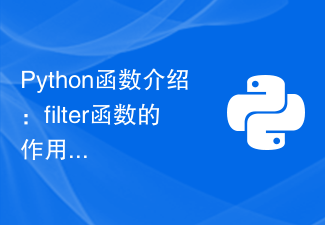
Introduction to Python functions: The role and examples of the filter function Python is a powerful programming language that provides many built-in functions, one of which is the filter function. The filter function is used to filter the elements in the list and return a new list composed of elements that meet the specified conditions. In this article, we will introduce what the filter function does and provide some examples to help readers understand its usage and potential. The syntax of the filter function is as follows: filter(function
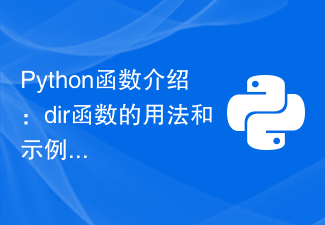
Introduction to Python functions: Usage and examples of dir function Python is an open source, high-level, interpreted programming language. It can be used to develop various types of applications, including web applications, desktop applications, games, etc. Python provides a large number of built-in functions and modules that can help programmers write efficient Python code quickly. Among them, the dir function is a very useful built-in function, which can help programmers view the properties and methods in objects, modules or classes.
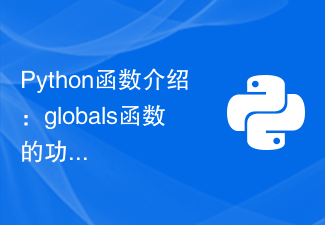
Introduction to Python functions: functions and usage examples of the globals function Python is a powerful programming language that provides many built-in functions, among which the globals() function is one of them. This article will introduce the functions and usage examples of the globals() function, with specific code examples. 1. Functions of the globals function The globals() function is a built-in function that returns a dictionary of global variables of the current module. It returns a dictionary containing global variables, where
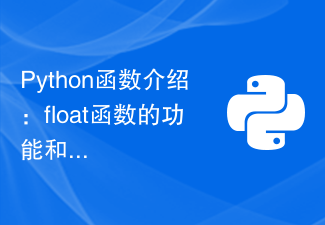
Introduction to Python functions: functions and usage examples of float functions. Python is a high-level programming language widely used in many fields. It provides a wealth of built-in functions so that developers can develop and process data more conveniently. One of them is the float function, which is used to convert strings or numbers into floating point types. In this article, we will introduce the functions of the float function in detail and give some usage examples. Introduction to the function of float function: float function in Python
