How to use Redis to speed up website access
How to use Redis to accelerate website access speed
With the rapid development of the Internet, website access speed has become one of the important factors in user experience. In terms of optimizing website performance, Redis, as a high-performance in-memory database, is widely used to speed up website access. This article will introduce how to use Redis to speed up website access and provide specific code examples.
1. Why choose Redis
Redis is a high-performance key-value database based on memory. Its features include:
- Data is stored in memory and read The writing speed is extremely fast and is suitable for scenarios that require high response speed;
- Supports a variety of data structures, such as key-value pairs, lists, sets, etc., and provides many efficient operation methods;
- Supports data persistence, and can save data in memory to disk to prevent data loss;
- Supports distributed deployment, and can improve performance and availability by building a Redis cluster.
Due to the above characteristics, Redis is widely used in cache, message queue, counter and other scenarios, which can significantly improve website access speed.
2. How to use Redis to accelerate website access speed
- Page caching
Page caching is a common method to use Redis to accelerate website access speed. When a user accesses a page, first check from Redis whether the page has been cached. If it has been cached, the cached result will be returned directly; if it has not been cached, the page will be generated and stored in Redis, and the next time the user visits again, it will be obtained directly from the cache.
The following is a sample code that uses Python language and Redis to implement page caching:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
|
- Data caching
In addition to page caching, you can also use Redis Cache some popular data to reduce the pressure on the database and improve access speed. For example, frequently used data can be stored in Redis instead of querying the database every time it is accessed.
The following is a sample code that uses Java language and Redis to implement data caching:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
|
3. Notes
When using Redis to speed up website access, you need to pay attention to the following A few points:
- Set the cache time reasonably: According to the characteristics of the website and the frequency of access, set the cache time reasonably to avoid data expiration or not updating for a long time;
- Process data consistency Problem: Since the data is stored in Redis, it is necessary to ensure the data consistency between Redis and the database, which can be solved by updating the cache, delayed synchronization and other mechanisms;
- Control the cache size: Redis is a memory-based database. You need to pay attention to controlling the size of the cache to prevent system performance from degrading due to excessive memory usage;
- High availability and fault tolerance: Use Redis cluster to improve availability and fault tolerance and prevent single points of failure.
Summary:
Using Redis can greatly speed up website access. Through page caching and data caching, the frequency of database access can be significantly reduced and the response speed and concurrency capabilities can be improved. When using Redis, you need to set the cache time appropriately, deal with data consistency issues, control the cache size, and ensure high availability and fault tolerance.
Reference materials:
- Redis official documentation: https://redis.io/documentation
The above is the detailed content of How to use Redis to speed up website access. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


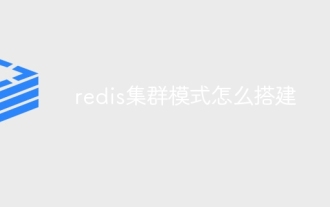
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
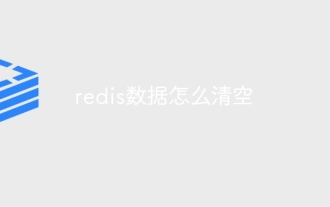
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
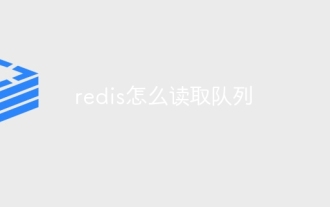
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
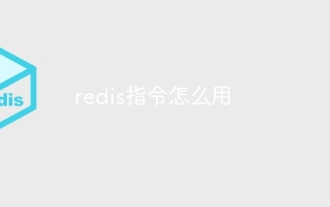
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
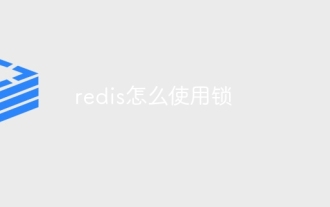
Using Redis to lock operations requires obtaining the lock through the SETNX command, and then using the EXPIRE command to set the expiration time. The specific steps are: (1) Use the SETNX command to try to set a key-value pair; (2) Use the EXPIRE command to set the expiration time for the lock; (3) Use the DEL command to delete the lock when the lock is no longer needed.
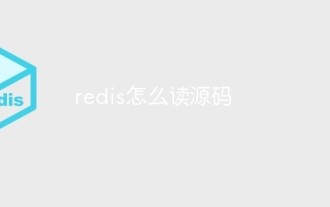
The best way to understand Redis source code is to go step by step: get familiar with the basics of Redis. Select a specific module or function as the starting point. Start with the entry point of the module or function and view the code line by line. View the code through the function call chain. Be familiar with the underlying data structures used by Redis. Identify the algorithm used by Redis.

Redis data loss causes include memory failures, power outages, human errors, and hardware failures. The solutions are: 1. Store data to disk with RDB or AOF persistence; 2. Copy to multiple servers for high availability; 3. HA with Redis Sentinel or Redis Cluster; 4. Create snapshots to back up data; 5. Implement best practices such as persistence, replication, snapshots, monitoring, and security measures.
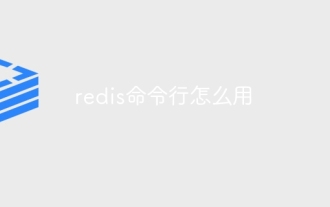
Use the Redis command line tool (redis-cli) to manage and operate Redis through the following steps: Connect to the server, specify the address and port. Send commands to the server using the command name and parameters. Use the HELP command to view help information for a specific command. Use the QUIT command to exit the command line tool.
