How to implement distributed counters using Redis
Redis is a high-performance cache database that is widely used in web applications. Among them, a common scenario is to use Redis to implement distributed counters. In this article, we will introduce how to implement distributed counters using Redis and provide specific code examples.
1. What is a distributed counter?
Distributed counter is a shared resource used for counting, which is characterized by being accessed by multiple clients at the same time. In a traditional stand-alone environment, counters can be implemented through simple variables or files. However, in a distributed environment, simultaneous access by multiple clients needs to be considered. In this case, if you only use local variables or files, multiple clients may update at the same time, which may cause inconsistencies in the counters.
2. How to use Redis to implement distributed counters?
Redis provides an atomic operation - INCR, which can operate counters in Redis and ensure the consistency of counters. In Redis, you can use the INCR command to implement distributed counters. The INCR command is atomic, that is, multiple clients call the INCR command at the same time. Each call will increase the counter value by 1 and return the increased value. The execution process of the INCR command is as follows:
- 1. Check whether the counter exists. If it does not exist, initialize it to 0
- 2. Add the counter value to 1
- 3. Return the value of the counter
When using the INCR command, you need to pay attention to the following two points:
- 1. The initial value of the counter should be 0, otherwise Calling the INCR command once will not obtain the correct result
- 2. For counters that are not used for a long time, you can use the EXPIRE command to set the expiration time to avoid occupying too many memory resources.
Next, we will provide a specific code example to introduce how to use Redis to implement distributed counters.
3. Code Example
The following is a Python code example using Redis to implement a distributed counter:
import redis # 连接Redis数据库 r = redis.StrictRedis(host='localhost', port=6379) # 定义计数器的关键字 counter_key = 'my_counter' # 如果计数器不存在,则将其初始化为0 if not r.exists(counter_key): r.set(counter_key, 0) # 调用INCR操作,增加计数器的值 r.incr(counter_key) # 输出计数器的当前值 counter_value = r.get(counter_key) print('Counter value:', counter_value)
The above code first connects to the locally running Redis database, and then defines The counter keyword, then checks whether the counter exists, and initializes it to 0 if it does not exist. Finally, call the INCR command and obtain the current value of the counter and output it to the console.
4. Summary
This article introduces how to use Redis to implement distributed counters and provides a Python code example. Specifically, we used the atomic operation provided by Redis - the INCR command to operate the counter. In a distributed environment, using Redis to implement distributed counters can ensure the consistency of the counter and avoid inconsistency problems caused by multiple clients operating the counter at the same time.
The above is the detailed content of How to implement distributed counters using Redis. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
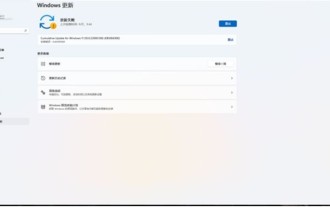
1. Start the [Start] menu, enter [cmd], right-click [Command Prompt], and select Run as [Administrator]. 2. Enter the following commands in sequence (copy and paste carefully): SCconfigwuauservstart=auto, press Enter SCconfigbitsstart=auto, press Enter SCconfigcryptsvcstart=auto, press Enter SCconfigtrustedinstallerstart=auto, press Enter SCconfigwuauservtype=share, press Enter netstopwuauserv , press enter netstopcryptS
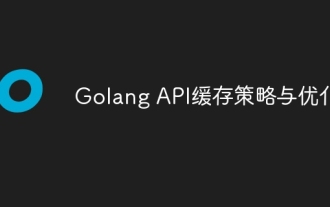
The caching strategy in GolangAPI can improve performance and reduce server load. Commonly used strategies are: LRU, LFU, FIFO and TTL. Optimization techniques include selecting appropriate cache storage, hierarchical caching, invalidation management, and monitoring and tuning. In the practical case, the LRU cache is used to optimize the API for obtaining user information from the database. The data can be quickly retrieved from the cache. Otherwise, the cache can be updated after obtaining it from the database.
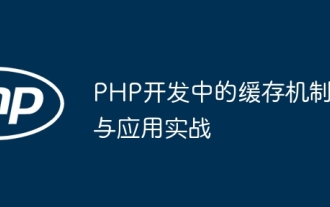
In PHP development, the caching mechanism improves performance by temporarily storing frequently accessed data in memory or disk, thereby reducing the number of database accesses. Cache types mainly include memory, file and database cache. Caching can be implemented in PHP using built-in functions or third-party libraries, such as cache_get() and Memcache. Common practical applications include caching database query results to optimize query performance and caching page output to speed up rendering. The caching mechanism effectively improves website response speed, enhances user experience and reduces server load.
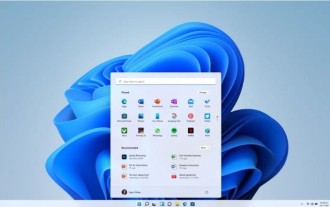
First you need to set the system language to Simplified Chinese display and restart. Of course, if you have changed the display language to Simplified Chinese before, you can just skip this step. Next, start operating the registry, regedit.exe, directly navigate to HKEY_LOCAL_MACHINESYSTEMCurrentControlSetControlNlsLanguage in the left navigation bar or the upper address bar, and then modify the InstallLanguage key value and Default key value to 0804 (if you want to change it to English en-us, you need First set the system display language to en-us, restart the system and then change everything to 0409) You must restart the system at this point.
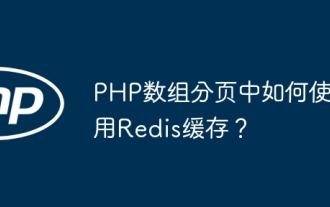
Using Redis cache can greatly optimize the performance of PHP array paging. This can be achieved through the following steps: Install the Redis client. Connect to the Redis server. Create cache data and store each page of data into a Redis hash with the key "page:{page_number}". Get data from cache and avoid expensive operations on large arrays.
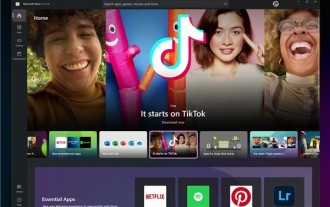
1. First, double-click the [This PC] icon on the desktop to open it. 2. Then double-click the left mouse button to enter [C drive]. System files will generally be automatically stored in C drive. 3. Then find the [windows] folder in the C drive and double-click to enter. 4. After entering the [windows] folder, find the [SoftwareDistribution] folder. 5. After entering, find the [download] folder, which contains all win11 download and update files. 6. If we want to delete these files, just delete them directly in this folder.
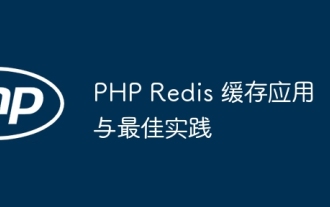
Redis is a high-performance key-value cache. The PHPRedis extension provides an API to interact with the Redis server. Use the following steps to connect to Redis, store and retrieve data: Connect: Use the Redis classes to connect to the server. Storage: Use the set method to set key-value pairs. Retrieval: Use the get method to obtain the value of the key.
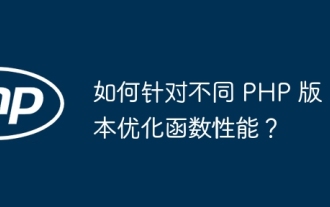
Methods to optimize function performance for different PHP versions include: using analysis tools to identify function bottlenecks; enabling opcode caching or using an external caching system; adding type annotations to improve performance; and selecting appropriate string concatenation and sorting algorithms according to the PHP version.
