


How to use PHP to develop cache to improve the user conversion rate of the website
How to use PHP to develop cache to improve the user conversion rate of the website
Introduction:
With the development of the Internet, the number of users of the website is also becoming larger and larger. In this digital era, users have increasingly higher requirements for website access speed and experience. Especially for sites that need to refresh data frequently, such as e-commerce websites, how to improve the response speed of the website has become an important issue. Using PHP to develop cache is a simple and effective way to improve website performance and user conversion rate.
1. What is caching
Caching refers to temporarily saving calculation results for future use. In website development, cache can be used to store some frequently accessed data, which can reduce the number of accesses to the database and improve the response speed of the website.
2. Why use caching
- Improve performance: By storing frequently accessed data in the cache, you can reduce the number of queries to the database, thereby improving the response speed of the website.
- Reduce server load: Caching can reduce access to databases and other external resources, reduce server load, and improve website stability.
- Improve user experience: The faster the website responds, the better the user experience, and the conversion rate will increase accordingly.
3. How to use PHP to develop caching
-
Using file caching
File caching is a simple and direct caching method that saves data in file, and set the appropriate expiration time, you can use the file_put_contents() function to write data to the file, and use the file_get_contents() function to read data from the file.Sample code:
function getDataFromCache($key, $expire = 3600) { $file = './cache/' . md5($key) . '.txt'; if (file_exists($file) && time() - filemtime($file) < $expire) { return file_get_contents($file); } else { return false; } } function setDataToCache($key, $data) { $file = './cache/' . md5($key) . '.txt'; file_put_contents($file, $data); }
Copy after login Using memory cache
Memory cache is an efficient caching method that saves data in memory and is faster. In PHP, you can use caching systems such as Memcached or Redis to achieve this.Sample code (using Memcached):
$memcached = new Memcached(); $memcached->addServer('localhost', 11211); function getDataFromCache($key) { global $memcached; return $memcached->get($key); } function setDataToCache($key, $data, $expire = 3600) { global $memcached; $memcached->set($key, $data, $expire); }
Copy after login
4. Further optimization of cache
Use cache tags
Cache tags are flags set to facilitate cache management. By adding tags to cached data, you can distinguish the cached data of different pages, thereby better controlling the update and deletion of cached data.Sample code:
function getDataFromCache($key, $tag) { // 根据标记获取缓存数据 } function setDataToCache($key, $tag, $data, $expire = 3600) { // 设置缓存数据并标记 } function deleteCacheByTag($tag) { // 删除指定标记的缓存数据 }
Copy after login- Set the cache update policy
The validity time of the cache is very important. If the cache time is too long, it may cause the data to expire or be inaccurate; if the cache If the time is too short, the frequency of database access will increase. Reasonable cache update strategies can be set based on different business needs, such as regularly refreshing the cache and dynamically updating the cache based on data updates.
Conclusion:
Using PHP to develop cache is an important method to improve website performance and user conversion rate. By rationally using cache, you can reduce access to external resources such as databases, improve the response speed and stability of the website, and improve user experience. During the development process, it is necessary to select the appropriate cache type based on specific business needs and consider the cache update strategy to achieve optimal performance. I hope this article will be helpful to your development work and improve the conversion rate of website users.
The above is the detailed content of How to use PHP to develop cache to improve the user conversion rate of the website. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


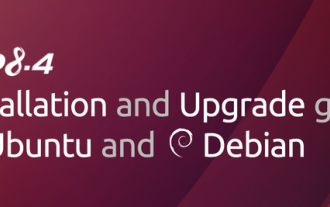
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
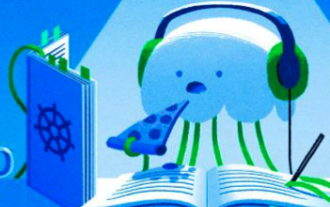
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
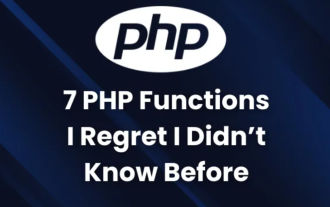
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
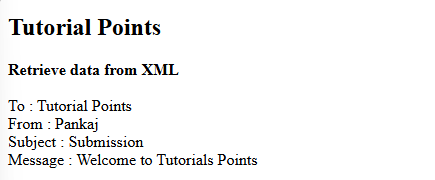
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
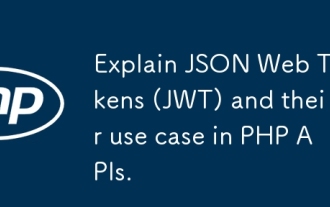
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
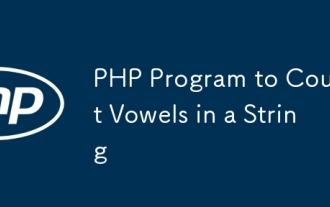
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
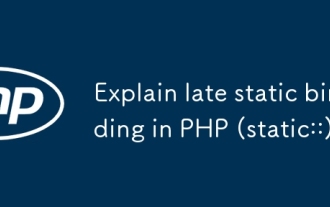
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
