How to use Redis to implement distributed geographical location query
How to use Redis to implement distributed geographical location query
Geographical location query can be seen everywhere in our daily life, such as finding nearby restaurants, locating express packages, etc. In traditional relational databases, implementing geographical location queries requires complex spatial indexes and distance calculations, which are inefficient for large-scale data volumes. As a high-performance non-relational in-memory database, Redis has excellent caching characteristics and distributed support, and is very suitable for implementing distributed geographical location queries. This article will introduce how to use Redis to implement this function and provide specific code examples.
1. Data structure design
Before implementing distributed geographical location query, we need to design a suitable data structure first. Redis provides a sorted set to store geographical location information. Each geographical location can be represented by longitude and latitude.
We can use longitude and latitude as the score in the ordered set, and the unique identifier of the geographical location as the member in the ordered set. In this way, you can use the characteristics of ordered sets to quickly sort and search according to scores.
2. Data Insertion
Before inserting geographical location data, we need to connect to the Redis server first. This can be achieved using Jedis, the Java client for Redis. The following is a code example for inserting geographical location data:
import redis.clients.jedis.Jedis; public class GeoLocationInsert { public static void main(String[] args) { // 连接Redis服务器 Jedis jedis = new Jedis("localhost", 6379); // 设置地理位置经纬度 double longitude = 116.403834; double latitude = 39.915216; // 添加地理位置数据到有序集合 jedis.zadd("geo:locations", longitude, latitude, "Beijing"); // 关闭连接 jedis.close(); } }
3. Data query
When querying nearby geographical location data, we can use the range query function of an ordered collection. The following is a code example for querying nearby geographical location data:
import redis.clients.jedis.Jedis; import redis.clients.jedis.GeoRadiusResponse; import redis.clients.jedis.params.GeoRadiusParam; public class GeoLocationQuery { public static void main(String[] args) { // 连接Redis服务器 Jedis jedis = new Jedis("localhost", 6379); // 设置中心地理位置经纬度 double longitude = 116.403834; double latitude = 39.915216; // 查询附近地理位置数据 GeoRadiusResponse[] responses = jedis.georadius("geo:locations", longitude, latitude, 10, GeoUnit.KM, GeoRadiusParam.geoRadiusParam().withDist()); // 打印查询结果 for (GeoRadiusResponse response : responses) { System.out.println(response.getMemberByString() + ", 距离: " + response.getDistance()); } // 关闭连接 jedis.close(); } }
In the above code, we set the longitude and latitude of the central geographical location, and then use the georadius
command to query the specified geographical location away from the center Nearby location data within a distance range. The returned result contains the unique identifier (member) and distance (dist) of the nearby geographical location.
It should be noted that the last parameter of the jedis.georadius
method is GeoRadiusParam.geoRadiusParam().withDist()
, which means that distance information needs to be returned.
4. Distributed deployment
When implementing distributed geographical location query, we can store geographical location data on multiple Redis nodes and evenly distribute the data to on each node. This enables load balancing and high availability.
The following is a code example that uses Redis Cluster to implement distributed geographical location query:
import redis.clients.jedis.HostAndPort; import redis.clients.jedis.JedisCluster; import java.util.HashSet; import java.util.Set; public class GeoLocationClusterQuery { public static void main(String[] args) { Set<HostAndPort> jedisClusterNodes = new HashSet<>(); jedisClusterNodes.add(new HostAndPort("localhost", 7000)); jedisClusterNodes.add(new HostAndPort("localhost", 7001)); jedisClusterNodes.add(new HostAndPort("localhost", 7002)); jedisClusterNodes.add(new HostAndPort("localhost", 7003)); jedisClusterNodes.add(new HostAndPort("localhost", 7004)); jedisClusterNodes.add(new HostAndPort("localhost", 7005)); // 连接Redis Cluster JedisCluster jedisCluster = new JedisCluster(jedisClusterNodes); // 设置中心地理位置经纬度 double longitude = 116.403834; double latitude = 39.915216; // 查询附近地理位置数据 GeoRadiusResponse[] responses = jedisCluster.georadius("geo:locations", longitude, latitude, 10, GeoUnit.KM, GeoRadiusParam.geoRadiusParam().withDist()); // 打印查询结果 for (GeoRadiusResponse response : responses) { System.out.println(response.getMemberByString() + ", 距离: " + response.getDistance()); } // 关闭连接 jedisCluster.close(); } }
In the above code, we use the JedisCluster
class to connect to the Redis Cluster cluster, and then Geolocation query.
5. Summary
Using Redis to implement distributed geographical location query can greatly improve query efficiency and scalability. With appropriate data structure design and code implementation, we can easily store and query geolocation data. At the same time, distributed deployment can ensure high availability and load balancing.
The above is the method and sample code for using Redis to implement distributed geographical location query. Hope this article can be helpful to you.
The above is the detailed content of How to use Redis to implement distributed geographical location query. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


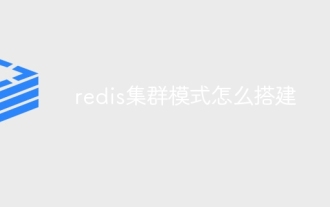
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
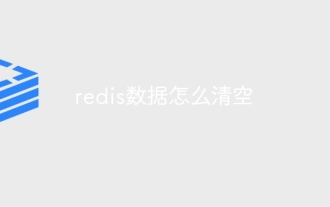
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
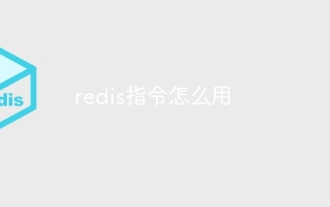
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).

Redis uses a single threaded architecture to provide high performance, simplicity, and consistency. It utilizes I/O multiplexing, event loops, non-blocking I/O, and shared memory to improve concurrency, but with limitations of concurrency limitations, single point of failure, and unsuitable for write-intensive workloads.
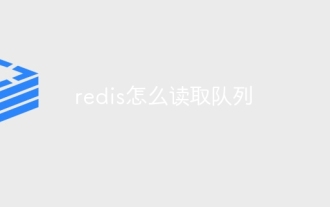
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
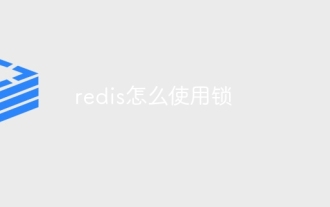
Using Redis to lock operations requires obtaining the lock through the SETNX command, and then using the EXPIRE command to set the expiration time. The specific steps are: (1) Use the SETNX command to try to set a key-value pair; (2) Use the EXPIRE command to set the expiration time for the lock; (3) Use the DEL command to delete the lock when the lock is no longer needed.

Redis uses hash tables to store data and supports data structures such as strings, lists, hash tables, collections and ordered collections. Redis persists data through snapshots (RDB) and append write-only (AOF) mechanisms. Redis uses master-slave replication to improve data availability. Redis uses a single-threaded event loop to handle connections and commands to ensure data atomicity and consistency. Redis sets the expiration time for the key and uses the lazy delete mechanism to delete the expiration key.
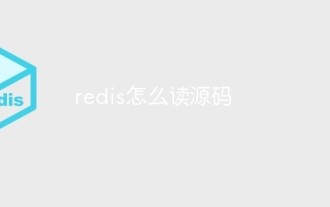
The best way to understand Redis source code is to go step by step: get familiar with the basics of Redis. Select a specific module or function as the starting point. Start with the entry point of the module or function and view the code line by line. View the code through the function call chain. Be familiar with the underlying data structures used by Redis. Identify the algorithm used by Redis.
