How to implement an audio player using Vue
Vue is a popular JavaScript framework with an efficient component-based development model and responsive data binding capabilities, suitable for developing richly interactive Web applications. In our actual development, the customization and development of UI components is a common requirement. This article will focus on how to use Vue to implement an audio player.
First, we need to install Vue.js. We can download the Vue.js file on the Vue official website, or install it using npm or yarn:
npm install vue
After the installation is complete, we can start building our audio player.
HTML part
In the HTML part, we need to first declare an audio tag <audio>
and all audio player control components. We can see that we use several buttons to control various states of the player respectively. These buttons will be bound to vue components. We can also use a div to display the music list, which will also be bound by the vue component. We also bound the playlist so we can add and remove music dynamically.
<div id="app"> <div class="audio-player"> <audio src="" id="audio" ref="audio"></audio> <!-- 播放按钮 --> <button class="button" v-on:click="playAudio"><i class="fa fa-play"></i></button> <!-- 暂停按钮 --> <button class="button" v-on:click="pauseAudio"><i class="fa fa-pause"></i></button> <!-- 上一个按钮 --> <button class="button" v-on:click="prevAudio"><i class="fa fa-chevron-left"></i></button> <!-- 下一个按钮 --> <button class="button" v-on:click="nextAudio"><i class="fa fa-chevron-right"></i></button> <div class="playlist"> <div class="list-item" v-for="(audio,index) in audioList" v-bind:key="index" v-on:click="changeAudio(index)"> {{audio.name}} </div> </div> </div> </div>
Definition of Vue component
Next, we need to define the Vue component and implement the method we just defined in HTML:
var vm = new Vue({ el: '#app', data: { audioList: [], // 音乐列表 currentAudio: { // 当前音乐信息 src: '', name: '', artist: '', }, currentIndex: 0, // 当前播放音乐在列表中的索引 playStatus: false, // 播放状态 }, methods: { // 播放音乐 playAudio: function() { this.playStatus = true this.$refs.audio.play() }, // 暂停音乐 pauseAudio: function() { this.playStatus = false this.$refs.audio.pause() }, // 播放下一首 nextAudio: function() { this.currentIndex++ if (this.currentIndex > this.audioList.length - 1) { this.currentIndex = 0 } this.currentAudio = this.audioList[this.currentIndex] this.$refs.audio.src = this.currentAudio.src this.playAudio() }, // 播放上一首 prevAudio: function() { this.currentIndex-- if (this.currentIndex < 0) { this.currentIndex = this.audioList.length - 1 } this.currentAudio = this.audioList[this.currentIndex] this.$refs.audio.src = this.currentAudio.src this.playAudio() }, // 切换音乐 changeAudio: function(index) { this.currentIndex = index this.currentAudio = this.audioList[this.currentIndex] this.$refs.audio.src = this.currentAudio.src this.playAudio() } } })
The core of the Vue component isdata
and methods
attributes. The data
attribute contains a set of variables containing music information and playlist information. They are monitored and updated at any time to ensure synchronization of page views and data. The methods
attribute contains a set of methods to update our music player as needed.
As we described before, we use an array of music information audioList
, and another object currentAudio
, which contains the currently playing music Complete information. We also define the currentIndex
variable to track the currently playing song, and use playStatus
to switch the playback status.
Our methods include: playAudio
and pauseAudio
control the playback (or pause) of music, nextAudio
and prevAudio
To switch to the next or previous music in the playlist respectively, changeAudio
to switch to the selected music.
Finally, use the $refs
method to reference the audio tag audio we previously declared in the HTML section, so that its play and pause methods can be called.
Bind Music List
We can now bind our player to the music list. Appropriate music files can be selected online and added to the music list. code show as below.
vm.audioList = [ { name: 'A Chill Sound', artist: 'Faster san', src: 'music/1.a-chill-sound.mp3' }, { name: 'Calm Breeze', artist: 'Suraj Bista', src: 'music/2.calm-breeze.mp3', }, { name: 'Happiness', artist: 'Erick McNerney', src: 'music/3.happiness.mp3' } ]; vm.currentAudio = vm.audioList[vm.currentIndex]; vm.$refs.audio.src = vm.currentAudio.src;
We can enjoy our music now. This article shows how to create a simple music player using Vue.js, and we see how to use its data binding and method calling capabilities to create dynamic applications. When implementing features, it is crucial to organize your code neatly and clearly, with security and ease of use in mind for end-to-end functionality.
The above is the detailed content of How to implement an audio player using Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




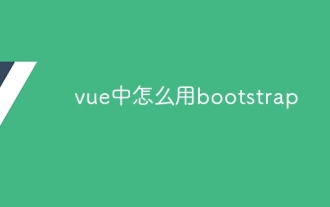
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
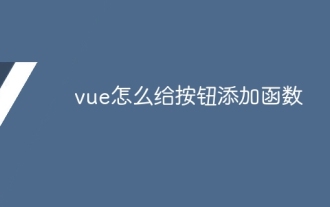
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
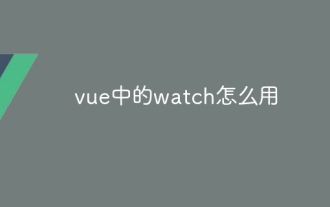
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
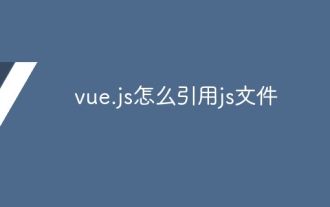
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
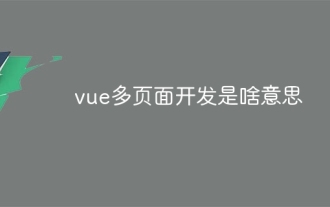
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
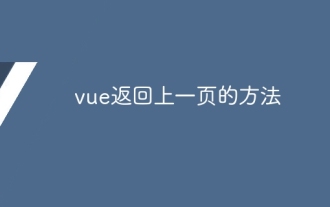
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.
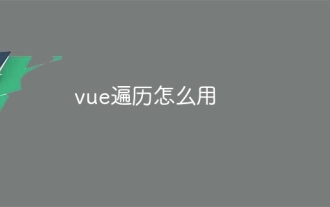
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
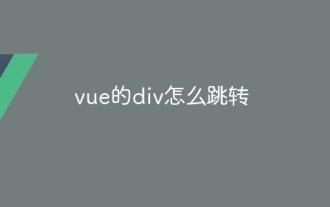
There are two ways to jump div elements in Vue: use Vue Router and add router-link component. Add the @click event listener and call this.$router.push() method to jump.
