How to use Vue to achieve a picture magnifying glass effect
How to use Vue to achieve the picture magnifying glass effect
Introduction:
The picture magnifying glass effect is a common web page interaction effect. When you hover the mouse over the picture, you can Enlarges the image and shows details of the enlarged portion. This article will introduce how to use the Vue framework to achieve the picture magnifying glass effect, and provide specific code examples for reference.
1. Requirements analysis:
We need to implement a picture magnifying glass effect in the Vue project. When the user hovers the mouse over the picture, the picture can be enlarged and the details of the enlarged part can be displayed. Specifically, we need to implement the following functions:
- When the mouse hovers over the picture, a magnifying glass frame is displayed, and the enlarged part of the image is displayed in the frame;
- When the mouse is on the picture When moving up, the position of the magnifying glass frame and the displayed magnified image are updated;
- When the mouse leaves the picture, the magnifying glass frame is hidden.
2. Technical implementation:
We will use the Vue framework and some basic HTML and CSS to achieve the picture magnifying glass effect. The following are the specific steps for implementation:
- Create a Vue component:
First, we need to create a Vue component to contain the code for the picture magnifying glass effect.
<template> <div class="image-magnifier"> <div class="magnifier" v-show="showMagnifier" :style="magnifierPosition"></div> <img class="image lazy" src="/static/imghw/default1.png" data-src="imageSrc" : @mousemove="onMouseMove" @mouseover="onMouseOver" @mouseout="onMouseOut" /> </div> </template> <script> export default { data() { return { showMagnifier: false, magnifierPosition: { left: 0, top: 0 }, imageSrc: 'path/to/your/image.jpg' }; }, methods: { onMouseMove(event) { // 更新放大镜框的位置 }, onMouseOver() { // 鼠标悬浮在图片上时显示放大镜框 }, onMouseOut() { // 鼠标离开图片时隐藏放大镜框 } } }; </script> <style scoped> .image-magnifier { position: relative; } .magnifier { position: absolute; width: 200px; // 定义放大镜框的宽度 height: 200px; // 定义放大镜框的高度 background-color: rgba(0, 0, 0, 0.5); // 定义放大镜框的背景颜色 pointer-events: none; // 禁用放大镜框的鼠标事件 } .image { display: block; max-width: 100%; height: auto; } </style>
- To achieve the magnifying glass effect:
In the above code, we use thev-show
instruction to control the display and hiding of the magnifying glass frame. In theonMouseMove
method, we will update the position of the magnifying glass frame and calculate theleft
andtop
properties of the magnifying glass frame based on the position of the mouse. In theonMouseOver
andonMouseOut
methods, we control the display and hiding of the magnifying glass frame respectively.
3. Usage Example:
It is very simple to use this picture magnifying glass component in a Vue project, just quote it where you need to display the picture.
<template> <div> <!-- 其他页面内容 --> <ImageMagnifier /> <!-- 其他页面内容 --> </div> </template> <script> import ImageMagnifier from './ImageMagnifier.vue'; export default { // 其他组件配置 components: { ImageMagnifier } } </script>
Summary:
Through the above steps, we have successfully implemented a simple picture magnifying glass effect using the Vue framework. When you hover the mouse over the image, you can enlarge the image and display the details of the enlarged part. Readers can modify and extend the code according to actual needs to meet more functional requirements.
Note: The imageSrc
attribute in the above code needs to be replaced with your image path. At the same time, the style and size of the magnifying glass frame can be customized according to actual needs.
The above is the detailed content of How to use Vue to achieve a picture magnifying glass effect. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


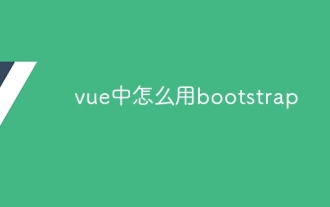
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
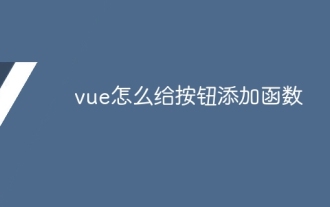
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
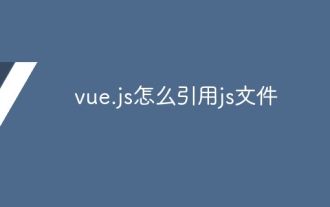
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
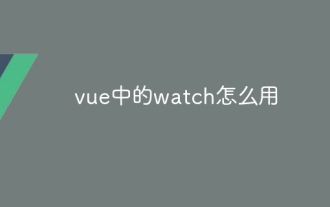
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
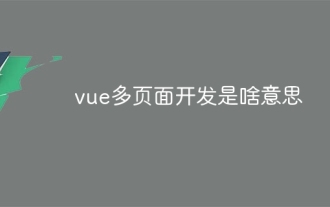
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
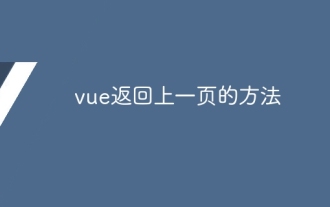
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.
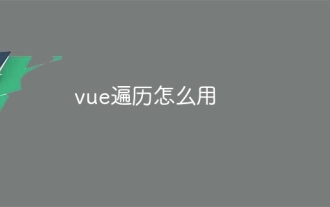
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
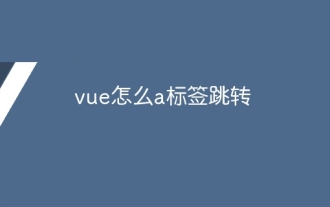
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
