


How to use Memcache to optimize data calculation operations in your PHP application?
Memcache is an open source distributed memory cache system that can quickly access data and improve application response speed. In PHP applications, Memcache can be used to cache calculation results, thereby optimizing the speed of data calculation operations. This article will introduce how to use Memcache to optimize data calculation operations in PHP applications and provide specific code examples.
- Installation and configuration of Memcache
Before using Memcache, you need to install and configure Memcache. You can install Memcache through the following command:
sudo apt-get install memcached php-memcached
After installation, you need to add the Memcache configuration option in the PHP configuration file:
extension=memcached.so
After configuring Memcache, you can test Memcache through the following code Whether the installation is successful:
$memcache = new Memcache(); $memcache->connect('localhost', 11211) or die ("Could not connect to Memcache"); $version = $memcache->getVersion(); echo "Memcache version: " . $version . "<br/>";
If the version information of Memcache is output, it means that Memcache has been successfully installed and configured.
- Cache calculation results
Next, consider how to use Memcache to cache calculation results. Suppose we have a calculation function calculate()
. Its calculation results may need to be used frequently. If it is recalculated every time, it will seriously reduce the response speed of the application. We can cache the calculation results in Memcache and read them directly from the cache the next time we use them.
function calculate($a, $b) { // 计算逻辑 return $result; } $memcache = new Memcache(); $memcache->connect('localhost', 11211) or die ("Could not connect to Memcache"); $key = md5($a . $b); // 计算缓存键值 $result = $memcache->get($key); if (!$result) { // 缓存未命中 $result = calculate($a, $b); $memcache->set($key, $result, 0, 3600); // 将结果缓存1小时 }
In the above code, we use the md5()
function to calculate a unique cache key value $key from the parameters $a and $b. If the cache of the key value exists, the result is read directly from the cache; otherwise, the result is recalculated and cached in Memcache, and the cache time is set to 1 hour. In this way, the next time the calculation function is used, the result can be read directly from the cache, avoiding the cost of repeated calculations.
- Automatic expiration cache
If the results returned by our calculation function calculate()
are affected by data updates, the cached results may Invalid. At this point, we need to manually clear the cache or set an appropriate cache time. However, manually clearing the cache may introduce more code complexity, and setting a cache time that is too long may lead to inconsistent cached results. To solve this problem, we can use Memcache's automatic expiration cache mechanism.
Memcache provides the parameter $expiration of the set()
function, which can be used to set the cache expiration time. Once the cache expires, Memcache will automatically clear the cache. Therefore, we can set the cache time to the data update cycle, so that even if the cache expires, there will only be a small performance loss.
function calculate($a, $b) { // 计算逻辑 return $result; } $memcache = new Memcache(); $memcache->connect('localhost', 11211) or die ("Could not connect to Memcache"); $key = md5($a . $b); // 计算缓存键值 $result = $memcache->get($key); if (!$result) { // 缓存未命中 $result = calculate($a, $b); $memcache->set($key, $result, 0, 60); // 将结果缓存1分钟,自动过期 }
In the above code, we set the cache time to 1 minute, that is, each calculation result can only be cached for 1 minute. If the data update cycle is within 1 minute, the cached results will basically not become invalid, and there is no need to manually clear the cache.
- Attention to Memcache details
When using Memcache to optimize data calculation operations, you need to pay attention to the following issues:
- When using memcached extension, When using Memcache, please pay attention to capitalization issues. For example, the first letters of operations such as set, get, add, etc. are capitalized; when using memcache extension, set, get, add, etc. are all lowercase.
- You need to pay attention to the meaning of the third parameter (flag) during the set operation. The default is 0. If it is written as 1, compression will be used during storage. This is different from zip or gzip in different languages. You can study the source code yourself and will not go into details in this article.
- Memcache distributed cache strongly recommends using version 1.4 or above.
- Note that some special characters cannot be encoded using md5, and an error will be reported. You need to base64 encode them first or use other methods.
- Memcache has certain limits on data size, generally no more than 1MB.
In general, using Memcache to optimize data calculation operations can greatly improve the response speed of the application and improve the user experience. It should be noted that Memcache is suitable for caching infrequently changing data such as calculation results, but is not suitable for caching frequently changing data. At the same time, you need to pay attention to the uniqueness of the cache key value, cache expiration time, Memcache size limit and other issues, in order to truly take advantage of Memcache.
The above is the detailed content of How to use Memcache to optimize data calculation operations in your PHP application?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


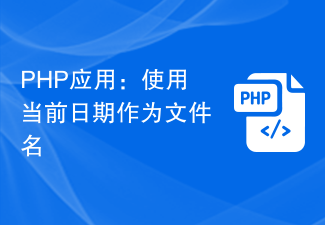
In PHP applications, we sometimes need to save or upload files using the current date as the file name. Although it is possible to enter the date manually, it is more convenient, faster and more accurate to use the current date as the file name. In PHP, we can use the date() function to get the current date. The usage method of this function is: date(format, timestamp); where format is the date format string, and timestamp is the timestamp representing the date and time. If this parameter is not passed, it will be used
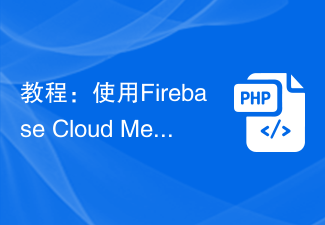
Tutorial: Using Firebase Cloud Messaging to implement scheduled message push functions in PHP applications Overview Firebase Cloud Messaging (FCM) is a free message push service provided by Google, which can help developers send real-time messages to Android, iOS and Web applications. This tutorial will lead you to use FCM to implement scheduled message push functions through PHP applications. Step 1: Create a Firebase project First, in F

1. What is generic programming? Generic programming refers to the implementation of a common data type in a programming language so that this data type can be applied to different data types, thereby achieving code reuse and efficiency. PHP is a dynamically typed language. It does not have a strong type mechanism like C++, Java and other languages, so it is not easy to implement generic programming in PHP. 2. Generic programming in PHP There are two ways to implement generic programming in PHP: using interfaces and using traits. Create an interface in PHP using an interface
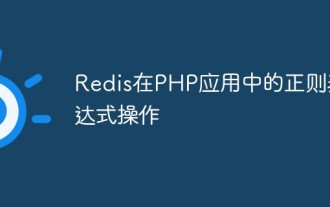
Redis is a high-performance key-value storage system that supports a variety of data structures, including strings, hash tables, lists, sets, ordered sets, etc. At the same time, Redis also supports regular expression matching and replacement operations on string data, which makes it highly flexible and convenient in developing PHP applications. To use Redis for regular expression operations in PHP applications, you need to install the phpredis extension first. This extension provides a way to communicate with the Redis server.
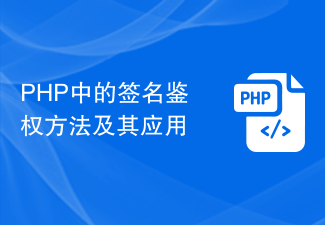
Signature Authentication Method and Application in PHP With the development of the Internet, the security of Web applications has become increasingly important. Signature authentication is a common security mechanism used to verify the legitimacy of requests and prevent unauthorized access. This article will introduce the signature authentication method and its application in PHP, and provide code examples. 1. What is signature authentication? Signature authentication is a verification mechanism based on keys and algorithms. The request parameters are encrypted to generate a unique signature value. The server then decrypts the request and verifies the signature using the same algorithm and key.
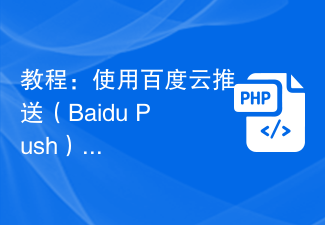
Tutorial: Use Baidu Cloud Push (BaiduPush) extension to implement message push function in PHP applications Introduction: With the rapid development of mobile applications, message push function is becoming more and more important in applications. In order to realize instant notification and message push functions, Baidu provides a powerful cloud push service, namely Baidu Cloud Push (BaiduPush). In this tutorial, we will learn how to use Baidu Cloud Push Extension (PHPSDK) to implement message push functionality in PHP applications. We will use Baidu Cloud
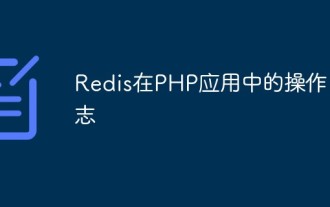
Redis operation logs in PHP applications In PHP applications, it has become more and more common to use Redis as a solution for caching or storing data. Redis is a high-performance key-value storage database that is fast, scalable, highly available, and has diverse data structures. When using Redis, in order to better understand the operation of the application and for data security, we need to have a Redis operation log. Redis operation log can record all clients on the Redis server
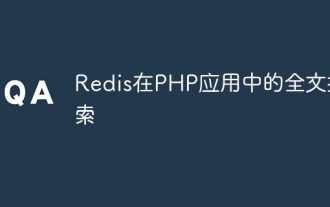
With the continuous development of Internet technology, the application of search engines is becoming more and more widespread. In the context of the Internet, search engines have become one of the main ways for users to obtain information. In this process, full-text search technology plays a crucial role. Full-text search indexes text content to quickly locate matching text when users query. There are many solutions to implement full-text search in PHP applications, and this article will focus on Redis' full-text search in PHP applications. Redis is a high-performance non-relational memory
